Question
Current Program looks like this: import java.util.Random; import java.util.Arrays; import java.util.Scanner; /** * This class contains the program to execute a text version of the
Current Program looks like this:
import java.util.Random;
import java.util.Arrays;
import java.util.Scanner;
/**
* This class contains the program to execute a text version of the MineSweeper game.
*
* @author
*
*/
public class MineSweeper extends Config {
/**
* This is the main method for Mine Sweeper game!
* This method contains the within game and play again loops and calls
* the various supporting methods.
*
* @param args (unused)
*/
public static void main(String[] args) {
while(true){
String resp;
Scanner in = new Scanner(System.in);
System.out.println("Welcome to Mine Sweeper!");
int width = promptUser(in, "What width of map would"
+ " you like (3 - 20): ", MIN_SIZE, MAX_SIZE);
int height = promptUser(in, "What height of map would"
+ " you like (3 - 20): ", MIN_SIZE, MAX_SIZE);
char map[][] = new char[height][width];
boolean[][] mines = new boolean[height][width];
eraseMap(map);
Random randGen = new Random(Config.SEED);
int totalMines = placeMines(mines, randGen);
while(true){
System.out.println();
System.out.println("Mines: " + totalMines);
simplePrintMap(map);
int row = promptUser(in, "row: ", 1, height);
int column = promptUser(in, "column: ", 1, width);
if(mines[row-1][column-1] ) {
map[row-1][column-1] = Config.SWEPT_MINE;
showMines(map,mines);
simplePrintMap(map);
System.out.println("Sorry, you lost.");
break;
}
else {
int nearby = numNearbyMines(mines, row-1, column-1);
map[row-1][column-1] = (char) (nearby + 48);
if(allSafeLocationsSwept(map, mines)) {
showMines(map,mines);
simplePrintMap(map);
System.out.println("You Win!");
break;
}
}
}
System.out.print("Would you like to play again (y)? ");
resp = in.nextLine();
resp = resp.trim();
if(resp.toLowerCase().charAt(0)=='y') {
continue;
}
else {
System.out.println("Thank you for playing Mine Sweeper!");
break;
}
}
}
/**
* This method prompts the user for a number, verifies that it is between min
* and max, inclusive, before returning the number.
*
* If the number entered is not between min and max then the user is shown
* an error message and given another opportunity to enter a number.
* If min is 1 and max is 5 the error message is:
* Expected a number from 1 to 5.
*
* If the user enters characters, words or anything other than a valid int then
* the user is shown the same message. The entering of characters other
* than a valid int is detected using Scanner's methods (hasNextInt) and
* does not use exception handling.
*
* Do not use constants in this method, only use the min and max passed
* in parameters for all comparisons and messages.
* Do not create an instance of Scanner in this method, pass the reference
* to the Scanner in main, to this method.
* The entire prompt should be passed in and printed out.
*
* @param in The reference to the instance of Scanner created in main.
* @param prompt The text prompt that is shown once to the user.
* @param min The minimum value that the user must enter.
* @param max The maximum value that the user must enter.
* @return The integer that the user entered that is between min and max,
* inclusive.
*/
public static int promptUser(Scanner in, String prompt, int min, int max){
int inp;
System.out.print(prompt);
while (true) {
if (in.hasNextInt()) {
inp = in.nextInt();
if(inp>=min && inp
in.nextLine();
return inp;
}
else {
System.out.println("Expected a number from " + min + " to " + max+".");
}
}
else {
System.out.println("Expected a number from " + min + " to " + max+".");
}
in.nextLine();
}
}
/**
* This initializes the map char array passed in such that all
* elements have the Config.UNSWEPT character.
* Within this method only use the actual size of the array. Don't
* assume the size of the array.
* This method does not print out anything. This method does not
* return anything.
*
* @param map An allocated array. After this method call all elements
* in the array have the same character, Config.UNSWEPT.
*/
public static void eraseMap(char[][] map) {
int i,j,row=map.length,col=map[0].length;
for(i=0;i for(j=0;j map[i][j]= UNSWEPT; return; } /** * This prints out a version of the map without the row and column numbers. * A map with width 4 and height 6 would look like the following: * . . . . * . . . . * . . . . * . . . . * . . . . * . . . . * For each location in the map a space is printed followed by the * character in the map location. * @param map The map to print out. */ public static void simplePrintMap(char[][] map) { for(char[] row : map){ for(char val : row) System.out.print( " "+val); System.out.println(); } return; } /** * This prints out the map. This shows numbers of the columns * and rows on the top and left side, respectively. * map[0][0] is row 1, column 1 when shown to the user. * The first column, last column and every multiple of 5 are shown. * * To print out a 2 digit number with a leading space if the number * is less than 10, you may use: * System.out.printf("%2d", 1); * * @param map The map to print out. */ public static void printMap(char[][] map) { return; //FIXME } /** * This method initializes the boolean mines array passed in. A true value for * an element in the mines array means that location has a mine, false means * the location does not have a mine. The MINE_PROBABILITY is used to determine * whether a particular location has a mine. The randGen parameter contains the * reference to the instance of Random created in the main method. * * Access the elements in the mines array with row then column (e.g., mines[row][col]). * * Access the elements in the array solely using the actual size of the mines * array passed in, do not use constants. * * A MINE_PROBABILITY of 0.3 indicates that a particular location has a * 30% chance of having a mine. For each location the result of * randGen.nextFloat() * determines whether that location has a mine. * * This method does not print out anything. * * @param mines The array of boolean that tracks the locations of the mines. * @param randGen The reference to the instance of the Random number generator * created in the main method. * @return The number of mines in the mines array. */ public static int placeMines(boolean[][] mines, Random randGen) { int minesNum =0; for(int i=0;i for(int j=0;j mines[i][j] = (randGen.nextFloat() if(mines[i][j]) minesNum++; } } return minesNum; } /** * This method returns the number of mines in the 8 neighboring locations. * For locations along an edge of the array, neighboring locations outside of * the mines array do not contain mines. This method does not print out anything. * * If the row or col arguments are outside the mines array, then return -1. * This method (or any part of this program) should not use exception handling. * * @param mines The array showing where the mines are located. * @param row The row, 0-based, of a location. * @param col The col, 0-based, of a location. * @return The number of mines in the 8 surrounding locations or -1 if row or col * are invalid. */ public static int numNearbyMines(boolean[][] mines, int row, int col) { int nearbyMines = 0; if (row >= 0 && row = 0 && col for (int i = row - 1; i for (int j = col - 1; j if (i >= 0 && i = 0 && j if (i != row || j != col) { if (mines[i][j] == true) nearbyMines++; } } } } } else { return -1; } return nearbyMines;} // If there exists a mine on a surrounding tile, add one to // "nearbyMines" // Test if the user selection is valid, if it is, test surrounding tiles (if they are valid) /** * This updates the map with each unswept mine shown with the Config.HIDDEN_MINE * character. Swept mines will already be mapped and so should not be changed. * This method does not print out anything. * * @param map An array containing the map. On return the map shows unswept mines. * @param mines An array indicating which locations have mines. No changes * are made to the mines array. */ public static void showMines(char[][] map, boolean[][] mines) { for(int i=0;i for(int j=0;j
Step by Step Solution
There are 3 Steps involved in it
Step: 1
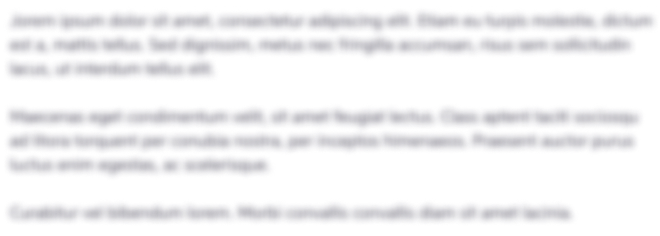
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started