Question
Currently I'm learning how to read from .txt files, and to print them out to a .txt file. I'm still pretty new to java coding.
Currently I'm learning how to read from .txt files, and to print them out to a .txt file. I'm still pretty new to java coding. So with my assignment I'm supposed to read in from a file that was provided by the professor, and write out to an outFile.txt. I cannot get anything to print in the outFile.txt. It keeps coming blank. I can't figure out what I did wrong. Can someone help please? I've got 5 classes, plus the driver class, and a text file. Thank you. Driver.java--
package project3;
import java.io.*;
import java.util.*;
import java.util.Scanner;
public class Driver {
public static void main(String[] args) throws IOException {
// TODO Auto-generated method stub
PrintWriter outFile = new PrintWriter(new FileWriter("outFile.txt"));
Scanner inFile = new Scanner(new FileReader("inFile.txt"));
while (inFile.hasNext()) {
String shapeType = inFile.next();
if (shapeType == "Rectangle") {
int rectLength = inFile.nextInt();
int rectWidth = inFile.nextInt();
Rectangle rectangle1 = new Rectangle(rectLength, rectWidth);
Rectangle.getrectArea();
Rectangle.getrectPerimiter();
outFile.println(rectangle1);
} // end of if
else if (shapeType == "SquAre") {
int sqSide = inFile.nextInt();
Square square1 = new Square(sqSide);
Square.getSqrArea();
Square.getSqrPerimeter();
outFile.println(square1);
}
else if (shapeType == "Circle") {
double circleRadius = inFile.nextInt();
double circleCircumference = inFile.nextInt();
Circle circle1 = new Circle(circleRadius, circleCircumference);
Circle.area();
Circle.circumference();
outFile.println(circle1);
}
else if (shapeType == "Triangle") {
int triBase = inFile.nextInt();
int triHeight = inFile.nextInt();
Triangle triangle1 = new Triangle(triBase, triHeight);
Triangle.gettriArea();
Triangle.gettriPerimiter();
outFile.println(triangle1);
}
else if (shapeType == "ParallelograM") {
int paraBase = inFile.nextInt();
int paraHeight = inFile.nextInt();
Parallelogram para1 = new Parallelogram(paraBase, paraHeight);
Parallelogram.getparaArea();
Parallelogram.getparaPerimiter();
outFile.println(para1);
}
else if (shapeType == "Square") {
int sqSide = inFile.nextInt();
Square square2 = new Square(sqSide);
Square.getSqrArea();
Square.getSqrPerimeter();
outFile.println(square2);
}
else if (shapeType == "rectangle") {
int rectLength = inFile.nextInt();
int rectWidth = inFile.nextInt();
Rectangle rectangle2 = new Rectangle(rectLength, rectWidth);
Rectangle.getrectArea();
Rectangle.getrectPerimiter();
outFile.println(rectangle2);
}
} // end of while
outFile.close();
inFile.close();
}// end of main
}// end of class
------
Circle.java--
package project3;
import java.text.DecimalFormat;
public class Circle {
DecimalFormat df = new DecimalFormat("#.00");
private static double radius;
private double area;
private double circumference;
// default constructor
public Circle() {
radius = 0;
area = 0;
circumference = 0;
}
// alt 1
public Circle(double Radius, double Circumference) {
radius = Radius;
circumference = Circumference;
}
// alt 2
public Circle(double Radius) {
radius = Radius;
}
// copy constructor
public Circle(Circle cir) {
this.radius = cir.radius;
this.area = cir.area;
this.circumference = cir.circumference;
}
// setter for radius
public void setRadius(double Radius) {
radius = Radius;
}
// getter for radius
public double getradius() {
return radius;
}
public static double area() {
double circleArea = (Math.PI * (radius * radius));
return circleArea;
}
public static double circumference() {
double circleCircumference = (2 * (Math.PI * radius));
return circleCircumference;
}
public String toString() {
String circleOutput = "The area of the circle is: " + df.format(area()) + ", and the circumference is: "
+ df.format(circumference());
return circleOutput;
}
}
---
Parallelogram.java--
package project3;
import java.text.DecimalFormat;
public class Parallelogram {
DecimalFormat df = new DecimalFormat("#.00");
private static int paraBase;
private static int paraHeight;
private int paraArea;
private int paraPerimiter;
// default constructor
public Parallelogram() {
paraBase = 0;
paraHeight = 0;
paraArea = paraBase * paraHeight;
paraPerimiter = 2 * (paraBase + paraHeight);
}
// alt constructor
public Parallelogram(int Base, int Height) {
paraBase = Base;
paraHeight = Height;
paraArea = paraBase * paraHeight;
paraPerimiter = 2 * (paraBase + paraHeight);
}
// alt constructor 2
public Parallelogram(int Base, int Height, int Area, int Perimiter) {
paraBase = Base;
paraHeight = Height;
paraArea = Area;
paraPerimiter = Perimiter;
}
// copy constructor
public Parallelogram(Parallelogram para) {
this.paraBase = para.paraBase;
this.paraHeight = para.paraHeight;
this.paraArea = para.paraArea;
this.paraPerimiter = para.paraPerimiter;
}
// setter for paraBase
public void setparaBase(int Base) {
paraBase = Base;
}
// getter for paraBase
public int getparaBase() {
return paraBase;
}
// setter for paraHeight
public void setparaHeight(int Height) {
paraHeight = Height;
}
// getter for paraHeight
public int getparaHeight() {
return paraHeight;
}
// setter for paraArea
public void setparaArea(int Area) {
paraArea = Area;
}
// getter for paraArea
public static int getparaArea() {
return paraBase * paraHeight;
}
// setter for paraPerimiter
public void setparaPerimiter(int Perimiter) {
paraPerimiter = Perimiter;
}
// getter for paraPerimiter
public static int getparaPerimiter() {
return 2 * (paraBase + paraHeight);
}
public String toString() {
String paraOutput = "The area of the Parallelogram is: " + df.format(getparaArea()) + ", and the Perimiter is: "
+ df.format(getparaPerimiter());
return paraOutput;
}
}
----
Triangle.java--
package project3;
import java.text.DecimalFormat;
public class Triangle {
DecimalFormat df = new DecimalFormat("#.00");
private static int triBase;
private static int triHeight;
private double triArea;
private double triPerimiter;
// default constructor
public Triangle() {
triBase = 0;
triHeight = 0;
triArea = (1 / 2 * (triBase * triHeight));
triPerimiter = triBase + triHeight + Math.sqrt(triBase * triBase + triHeight * triHeight);
}
// alt constructor 1
public Triangle(int Base, int Height) {
triBase = Base;
triHeight = Height;
}
// alt constructor 2
public Triangle(int Base, int Height, int Area, int Perimiter) {
triBase = Base;
triHeight = Height;
triArea = Area;
triPerimiter = Perimiter;
}
// copy constructor
public Triangle(Triangle tri) {
this.triBase = tri.triBase;
this.triHeight = tri.triHeight;
this.triArea = tri.triArea;
this.triPerimiter = tri.triPerimiter;
}
// setter for triBase
public void settriBase(int Base) {
triBase = Base;
}
// getter for triBase
public int gettriBase() {
return triBase;
}
// setter for triHeight
public void settriHeight(int Height) {
triHeight = Height;
}
// getter for triHeight
public int gettriHeight() {
return triHeight;
}
// setter for triArea
public void settriArea(double Area) {
triArea = Area;
}
// getter for triArea
public static double gettriArea() {
return 0.5 * triBase * triHeight;
}
// setter for triPerimiter
public void settriPerimiter(double Perimiter) {
triPerimiter = Perimiter;
}
// getter for triPerimiter
public static double gettriPerimiter() {
return triBase + triHeight + Math.sqrt(triBase * triBase + triHeight * triHeight);
}
public String toString() {
String triOutput = "The area of the triangle is: " + df.format(gettriArea()) + ", and the Perimiter is: "
+ df.format(gettriPerimiter());
return triOutput;
}
}
----
Rectangle.java--
package project3;
import java.text.DecimalFormat;
public class Rectangle {
DecimalFormat df = new DecimalFormat("#.00");
private static int rectLength;
private static int rectWidth;
private int rectArea;
private int rectPerimiter;
public Rectangle() {
rectLength = 0;
rectWidth = 0;
rectArea = rectLength * rectWidth;
rectPerimiter = 2 * (rectLength + rectWidth);
}// end of default
public Rectangle(int Length, int Width) {
rectLength = Length;
rectWidth = Width;
rectArea = Length * Width;
rectPerimiter = 2 * (Length + Width);
}// end of alt 1
public Rectangle(int Length, int Width, int Area, int Perimiter) {
rectLength = Length;
rectWidth = Width;
rectArea = Area;
rectPerimiter = Perimiter;
}// end of alt 2
// copy constructor
public Rectangle(Rectangle rec) {
this.rectLength = rec.rectLength;
this.rectWidth = rec.rectWidth;
this.rectArea = rec.rectArea;
this.rectPerimiter = rec.rectPerimiter;
}
// setter for rectLength
public void setrectLength(int Length) {
rectLength = Length;
}
// getter for rectLength
public int getrectLength() {
return rectLength;
}
// setter for rectWidth
public void setrectWidth(int Width) {
rectWidth = Width;
}
// getter for rectWidth
public int getrectWidth() {
return rectWidth;
}
// setter for rectArea
public void setrectArea(int Area) {
rectArea = Area;
}
// getter for rectArea
public static int getrectArea() {
return rectLength * rectWidth;
}
// setter for rectPerimiter
public void setrectPerimiter(int Perimiter) {
rectPerimiter = Perimiter;
}
// getter for rectPerimiter
public static int getrectPerimiter() {
return 2 * (rectLength + rectWidth);
}
public String toString() {
String rectOutput = "The area of the Rectangle is: " + df.format(getrectArea()) + ", and the Perimiter is: "
+ df.format(getrectPerimiter());
return rectOutput;
}
}
----
Square.java---
package project3;
import java.text.DecimalFormat;
public class Square {
DecimalFormat df = new DecimalFormat("#.00");
private static int side;
private int perimeter;
private int area;
public Square() {
side = 0;
}// end default
public Square(int Side) {
side = Side;
}// end of alt
// setter for SqrSide
public void setSqrSide(int Side) {
side = Side;
}
// getter for SqrSide
public int getSqrSide() {
return side;
}
// setter for SqrPerimeter
public void setSqrPerimeter(int SqrPerimeter) {
perimeter = SqrPerimeter;
}
// getter for SqrPerimeter
public static int getSqrPerimeter() {
return side * side * side * side;
}
// setter for SqrArea
public void setSqrArea(int SqrArea) {
area = SqrArea;
}
// getter for SqrArea
public static int getSqrArea() {
return (side * side);
}
public String toString() {
String sqrOutput = "The area of the Square is: " + df.format(getSqrArea()) + ", and the Perimiter is: "
+ df.format(getSqrPerimeter());
return sqrOutput;
}
}
----
And finally the inFile.txt that we're supposed to be reading from.
inFile.txt--
Rectangle 4 5 SquAre 3.0 Circle 4.5 circle 3.1 Triangle 10 10 10 ParallelograM 12 3 Square 10 rectangle 12 12
Step by Step Solution
There are 3 Steps involved in it
Step: 1
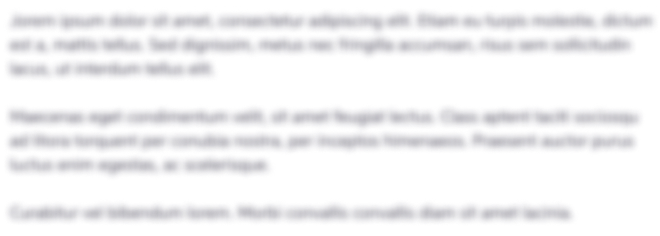
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started