Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Date Class A class the encapsulates year, and month and day values for Date stamp, comparison and Date IO purposes. MenuItem Class A class that
Date Class A class the encapsulates year, and month and day values for Date stamp, comparison and Date IO purposes. MenuItem Class A class that hold a text Item; (an option or title to be displayed) in a menu to be selected by the user. This is a fully private class that is only accessible by Menu (see next class) Menu Class A class that has several MenuItems to be displayed so the user can select one of them for an action to be executed in the program The Date Class The Date class was partially implemented by another program that left the company and it is your responsibility to complete the implementation: The date class encapsulates the following values: Year; an integer between the year 1500 till today Month, an integer between 1 and 12 Day, an integer between 1 and the number of days in the month. Error code; an integer that holds the code that corresponds to an error that recently happened or ZERO if the date object is valid and ready to be used. Current year; an integer that is automatically initialized to the current date of the system for validation purposes when a Date object is instantiated. The Date module (implemented in files Date.h and Date.cpp) is well documented and is placed in the project directory. Already implemented methods of the Date class and helper functions of Date Private functions: int daysSince0001_1_1()const; returns number of days passed since the date 0001/1/1 bool validate(); Validates the date setting the error code and then returns true, if valid, and false if invalid. void errCode(int theErrorCode); sets the error code value int systemYear()const; returns the current system year (2021 for this semester) bool bad()const; returns true if the Date is in an erroneous state. int mdays()const; returns the number of days in current month (the month stored in m_mon attribute) void setToToday(); sets the date to the current date (system date) Public functions and Constructors Date(); creates a date with current date Date(int year, int mon, int day); creates a date with assigned values then validates the date and sets the error code accordingly int errCode()const; returns the error code or zero if date is valid const char* dateStatus()const; returns a string stating the current status of the date int currentYear()const; returns the m_CUR_YEAR attribute value std::ostream& operator<<(std::ostream& os, const Date& RO); std::istream& operator>>(std::istream& is, Date& RO); Overloads of insertion and extraction operators to call write and read methods of Date Study the class and learn what each constant, variable and member function does and then using those functions and your knowledge of iostream, cin and cout, add the following member functions to the Date class: Your task is to develop the following the read method std::istream& read(std::istream& is = std::cin); This function reads a date from the console in the following format YYYY/MM/DD as follows: Clears the error code by setting it NO_ERROR Reads the year, the month and the day member variables using istream and ignores a single character after the year and the month values to bypass the Slashes. Note that the separators do not have to be Slash characters "/" but any separator that is not an integer number. Checks if istream has failed. If it did fail, it will set the error code to CIN_FAILED and clears the istream. If not, it will validate the values entered. Flushes the keyboard Returns the istream object The write method std::ostream& write(std::ostream& os = std::cout)const; If the Date object is in a "bad" state (it is invalid) print the "dateStatus()". Otherwise, the function should write the date in the following format using the ostream object: Prints the year Prints a Slash "/" Prints the month in two spaces, padding the left digit with zero if the month is a single-digit number Prints a Slash "/" Prints the day in two spaces, padding the left digit with zero if the day is a single-digit number Makes sure the padding is set back to spaces from zero Returns the ostream object. Comparison operator overload methods Overload the following comparison operators to compare two dates. bool operator== bool operator!= bool operator>= bool operator<= bool operator< bool operator> Use the return value of the daysSince0001_1_1() method to compare the two dates: Operator- method Returns the difference between two Dates in days. Example Date D1(2019, 12, 02), D2(2019, 11, 11); int days = D1 - D2; days in the above code snippet will hold the value 21. bool type conversion operator It will return true if the date is valid and false if it is not. Date Tester program Write your own tester or use the following tester program to test and debug your Date class. // Final Project Milestone 1 // Date Tester program // File dateTester.cpp // Version 1.0 // Date 2021/10/29 // Author Fardad Soleimanloo // Description // This programs test the student coding to complete of the Date class // // When testing before submission: DO NOT MODIFY THIS FILE IN ANY WAY // // // Revision History // ----------------------------------------------------------- // Name Date Reason // Fardad 2021/10/29 Preliminary release ///////////////////////////////////////////////////////////////// #include using namespace std; #include "Date.h" using namespace sdds; // A fool proof Date entry from console Date getDate(); int main() { Date D1, // daysSince0001_1_1 to be recieved from console D2(2021, 10, 29); cout << "Current Date: " << D1 << endl; cout << D1 - D2 << " days since ms1 was published" << endl; cout << "Please enter a date to be compared to " << D2 << endl << "(Also try some invalid values for testing): " << endl << "YYYY/MM/DD > "; D1 = getDate(); cout << "Your Entry: " << D1 << endl; cout << "Set by program to 2021/10/29: " << D2 << endl; cout << "Days between the two dates: " << D1 - D2 << endl; cout << "All the following statements must be correct: " << endl; if (D1 > D2) { cout << D1 << " > " << D2 << endl; } else { cout << D1 << " <= " << D2 << endl; } if (D1 < D2) { cout << D1 << " < " << D2 << endl; } else { cout << D1 << " >= " << D2 << endl; } if (D1 <= D2) { cout << D1 << " <= " << D2 << endl; } else { cout << D1 << " > " << D2 << endl; } if (D1 >= D2) { cout << D1 << " >= " << D2 << endl; } else { cout << D1 << " < " << D2 << endl; } if (D1 == D2) { cout << D1 << " == " << D2 << endl; } else { cout << D1 << " != " << D2 << endl; } if (D1 != D2) { cout << D1 << " != " << D2 << endl; } else { cout << D1 << " == " << D2 << endl; } return 0; } Date getDate() { Date D; do { cin >> D; // get D from console } while (!D && cout << D.dateStatus() << ", Please try again > "); // if D is invalid, print error message and loop return D; } output sample Note that the current date will change based on the day of execution Current Date: 2021/10/29 0 days since ms1 was published Please enter a date to be compared to 2021/10/29 (Also try some invalid values for testing): YYYY/MM/DD > abc cin Failed, Please try again > 1/1/1 Bad Year Value, Please try again > 2021/0/0 Bad Month Value, Please try again > 2021/10/30 Your Entry: 2021/10/30 Set by program to 2021/10/29: 2021/10/29 Days between the two dates: 1 All the following statements must be correct: 2021/10/30 > 2021/10/29 2021/10/30 >= 2021/10/29 2021/10/30 > 2021/10/29 2021/10/30 >= 2021/10/29 2021/10/30 != 2021/10/29 2021/10/30 != 2021/10/29 The Menu Module Create a module called Menu (in files Menu.cpp and Menu.h) this module will hold both MenuItem and Menu Classes' implementation code. Since the Menu class owns its MenuItem objects, it must have full control over the MenuItem creation. To implement this, have the declaration of the MenuItem and Menu class in the following sequence: Forward declare the class Menu in the header file. Implement the MenuItem class declaration (fully private) with Menu class as a friend. (see MenuItem class) Implement the Menu class declaration. (See Menu Class) The MenuItem Class Create a class called MenuItem. This class holds only one Cstring of characters for the content of the menu item dynamically. The length of the content is unknown. This class should be fully private (no public members; even constructor is private!): Make the "Menu" class a friend of this class (which makes MenuItem class only accessible by the Menu class). friend class Menu; Constructor Allocates and sets the content of the MenuItem to a Cstring value at the moment of instantiation (or initialization). If no value is provided for the description at the moment of creation, the MenuItem should be set to an empty state. Rule of Three A MenuItem object cannot be copied from or assigned to another MenuItem object. (Copy constructor and Copy assignment are deleted) Destructor Deallocates the content bool type conversion When a MenuItem is casted to "bool" it should return true, if it is not empty and it should return false if it is empty. const char* type conversion When a MenuItem is casted to "const char*" it should return the address of the content Cstring. displaying the MenuItem Create a method to display the content of the MenuItem on ostream. (No newline is printed after) Nothing is printed if MenuItem is empty. Remember that the MenuItem class is fully private. The Menu Class Create the Menu class as follows: Rule of Three A Menu Object can not be copied or assigned to another Menu Object. (Copy constructor and Copy assignment are deleted) Attributes This class has a minimum of three attributes. A MenuItem to possibly hold the title of the Menu. An array of MenuItem pointers. The size of this array is set by a constant unsigned integer defined in the Menu header file; called MAX_MENU_ITEMS. Have the MAX_MENU_ITEMS integer initialized to 20. This array will keep potential MenuItems added to the Menu. Each individual element of this array will hold the address of a dynamically allocated MenuItem as they are added to the Menu. (See insertion operator overload for Menu) Initialize this array of pointers to nullptrs. An integer to keep track of how many MenuItem pointers are pointing to dynamic MenuItem objects. (obviously, the value of this variable is always between 0 and MAX_MENU_ITEMS). Constructors A Menu is always created empty; with no MenuItems, with or without a title. Example: Menu A; Menu B("Lunch Menu"); Destructor Looping through the MenuItems array of pointers, it deletes each pointer up to the number of menu items in the menu. Methods Suggestion: create a function to display the title of the Menu on ostream if the title is not empty, otherwise, it does nothing. Create a function to display the entire Menu on ostream: This function first displays the title (if it is not empty) followed by a ":" and a new-line, then it will display all the MenuItems one by one; adding a row number in front of each. The row numbers are printed in two spaces, right justified followed by a "dash" and a "space". After printing all the MenuItems it should print " 0- Exit" and new-line and "> ". For example if the title is "Lunch Menu" and the menu items are "Omelet", "Tuna Sandwich" and "California Rolls", the Menu object should be printed like this: Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Rolls 0- Exit > Create a member function called run. This function displays the Menu and gets the user selection. (this function should be completely foolproof) The function receives nothing and returns an unsigned integer (That is the user's selection). After displaying the menu, ask for an integer and make sure the value of the integer is between 0 and the number of the menu items. If the user enters anything incorrect, print: "Invalid Selection, try again: " and get the integer again until a valid selection is made. Nice to do: The action of a foolproof integer entry within limits, with a prompt and an error message is a good candidate for a separate function implementation in the Utils module Overload operator~ to do exactly what the run function does (two different ways to run the menu) Overload a member insertion operator (operator<<) to add a MenuItem to the Menu. Menu& Menu::operator<<(const char* menuitemConent); This operator receives a C-style string containing the content of the MenuItem and returns the reference of the Menu object (*this). To accomplish this, check if the next spot for a MenuItem is available in the array of MenuItem pointers. If it is, dynamically create a MenuItem out of the content received through the operator argument and then store the address in the available spot and finally increase the number of allocated MenuItem pointers by one. If no spot is available, ( that is; if number of allocated MenuItem pointers is equal to MAX_MENU_ITEMS) this function silently ignores the action. At the end, return the reference of the Menu object. Usage example: int a; Menu M; M << "Omelet" << "Tuna Sandwich" << "California Rolls"; a = M.run() cout << "Your selection " << a << endl; output: 1- Omelet 2- Tuna Sandwich 3- California Rolls 0- Exit > 3 Your selection 3 Overload two type conversions for int and unsigned int to return the number of MenuItems on the Menu. Overload the type conversion for bool to return true if the Menu has one or more MenuItems otherwise, false; Overload the insertion operator to print the title of the Menu using cout. Example for the last three overloads: Menu M ("Lunch Menu"); M << "Omelet" << "Tuna Sandwich" << "California Rolls"; if (M) { // bool conversion cout << "The " << M << " is not empty and has " // insertion operator overload << unsigned int(M) << " menu items." << endl; // const int conversion (int conversion not tested) } Output: The Lunch Menu is not empty and has 3 menu items. Overload the indexing operator to return the const char* cast of the corresponding MenuItem in the array of MenuItem pointers. If the index passes the number of MenuItems in the Menu, loop back to the beginning. (use modulus) Example: Menu M; M << "Omelet" << "Tuna Sandwich" << "California Rolls"; cout << M[0] << endl; cout << M[1] << endl; cout << M[3] << endl; // << note that the last valid index is "2" The above code snippet will print the following: Omelet Tuna Sandwich Omelet Menu tester program Write your own tester or use the following tester program to test and debug your Menu class. // Final Project Milestone 1 // Menu Module // File menuTester.cpp // Version 1.0 // Author Fardad Soleimanloo // Revision History // ----------------------------------------------------------- // Name Date Reason // Fardad 2021/10/29 Preliminary release ///////////////////////////////////////////////////////////////// #include #include "Menu.h" #include "Utils.h" using namespace std; using namespace sdds; void showOrder(const unsigned int* cnt, const Menu& M); int main() { unsigned int selections[3]{}; unsigned int s; Menu m1("Lunch Menu"), m2; if (m2) { cout << "Wrong output, your bool cast is done incorrenctly!"; } else { cout << "The >" << m2 << "< menu is empty" << endl; } m1 << "Omelet" << "Tuna Sandwich" << "California Roll"; m2 << "Order more"; if (m1) { cout << "The " << m1 << " is not empty and has " << (unsigned int)(m1) << " menu items." << endl; } do { s = m1.run(); if (s) { selections[s - 1]++; cout << "you chose " << m1[s - 1] << endl; } } while (s != 0 || ~m2 != 0); showOrder(selections, m1); return 0; } void showOrder(const unsigned int* cnt, const Menu& M) { bool orderedSomthing = false; cout << "Your orders: " << endl; for (int i = 0; i < 3; i++) { if (cnt[i]) { orderedSomthing = true; cout << cnt[i] << " " << M[i] << (cnt[i] > 1 ? "s" : "") << endl; } } if (!orderedSomthing) cout << "You didn't order anything!" << endl; } Sample Execution The >< menu is empty The Lunch Menu is not empty and has 3 menu items. Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > 1 you chose Omelet Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > 1 you chose Omelet Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > 2 you chose Tuna Sandwich Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > 2 you chose Tuna Sandwich Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > 2 you chose Tuna Sandwich Lunch Menu: 1- Omelet 2- Tuna Sandwich 3- California Roll 0- Exit > abc Invalid Selection, try again: 5 Invalid Selection, try again: -1 Invalid Selection, try again: 0 1- Order more 0- Exit > 0 Your orders: 2 Omelets 3 Tuna Sandwichs
Step by Step Solution
There are 3 Steps involved in it
Step: 1
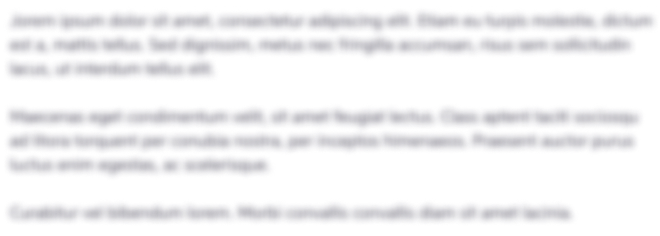
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started