Question
Date.h #ifndef DATE_H #define DATE_H #include #include using namespace std; class Date { private: int month, day, year; public: //default constructor Date(); //constructor with 3
Date.h
#ifndef DATE_H
#define DATE_H
#include
#include
using namespace std;
class Date
{
private:
int month, day, year;
public:
//default constructor
Date();
//constructor with 3 arguments
Date (int m, int d, int y);
//declare getters for the day and year
int getMonth() const;
int getDay() const;
int getYear() const;
void setMonth(int m);
//declare setters for the day and year
void setDay(int d);
void setYear(int y);
void printDate() const;
bool sameDay(const Date &d) const;
};
#endif
Date.cpp
#include "Date.h"
Date::Date()
{
month = 1;
day = 1;
year = 1;
}
Date::Date (int m, int d, int y)
{
assert (m >= 1 && m <= 12);
month = m;
assert (d >= 1 && d <= 31);
day = d;
assert (y > 0);
year = y;
}
int Date::getMonth() const
{
return month;
}
int Date::getDay() const
{
return day;
}
int Date::getYear() const
{
return year;
}
void Date::setMonth(int m)
{
assert (m >= 1 && m <= 12);
month = m;
}
void Date::setDay(int d)
{
assert (d >= 1 && d <= 31);
day = d;
}
void Date::setYear(int y)
{
assert (y > 0);
year = y;
}
void Date::printDate() const
{
cout << "The date is (M-D-Y): " << month << "-" << day << "-" << year << "-" << endl;
}
//implement sameDay function
bool Date::sameDay(const Date&d) const
{
//Write a code to implement same day function
}
main.cpp
#include
#include "Date.h"
using namespace std;
int main()
{
int tempMonth, tempDay, tempYear;
Date date1;
//instantiate one date object (date1) using the default constructor
cout << "Testing the default constructor and the getters" << endl
<< "The initialized date is (M-D-Y): "<< endl;
//use the getters to display the month, day, and year of date1 (should print the default values)
cout<cout<cout<cout<
//read keyboard input from the user for a month, day and year
cout<<"Please enter a date:(Month Day Year): "<cin>>tempMonth>>tempDay>>tempYear;
//use the setters to set the values of date1 to the values that came from the user
date1.setMonth(tempMonth);
date1.setDay(tempDay);
date1.setYear(tempYear);
//read keyboard input from the user for a second date
cout<<"Please enter a second date:(Month Day Year): "<cin>>tempMonth>>tempDay>>tempYear;
//use the constructor with three arguments to instantiate date2 to the second date input from the user
Date date2(tempMonth, tempDay, tempYear);
//print both objects using printDate
cout<date1.printDate();
date2.printDate();
//print a message to say if the two days are the same (testing the sameDay function)
if (date1.sameDay(date2))
{
cout<< "The two days are same "<}
else
{
cout<< "The two days are different "<}
return 0;
}
Implement the sameDay function to check if the two days are the same in date.cpp(testing the sameDay function )
Step by Step Solution
3.51 Rating (151 Votes )
There are 3 Steps involved in it
Step: 1
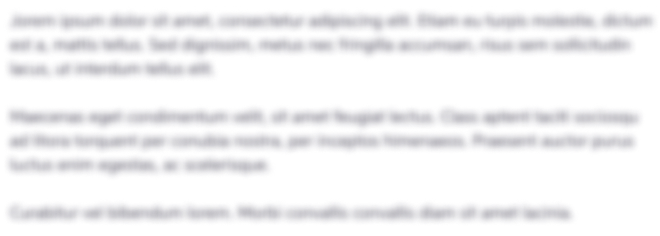
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started