Question
Dating App (JAVASCRIPT) Use loops, branches, and short circuit operators to create a dating app. Your app will ask a user for their information, including
Dating App (JAVASCRIPT)
Use loops, branches, and short circuit operators to create a dating app. Your app will ask a user for their information, including their birthday and favorite food, then ask for the same information for other people (matches). Your app will then compare information between the user and matches to determine a compatibility score.
General Guidelines:
Import the scanner and create the scanner object.
Create and update variables for each of the prompts provided in the template (lines 9-20). Consider what data types should be used.
When prompting for the month, make sure that the user puts in a month between 1 and 12.
When prompting for a day, make sure that the user puts in a proper day. Consider how some months have 28, 29, 30, and 31 days in them. We will be checking for proper leap year implementation.
Your app will first ask for the users own information.
After the user has entered their own information, the app will continually request the same information for other people (matches), until the exit string is entered when asking for a snapchat username.
While we are inputting user information, check if the snapchat username is equal to the string ~801~. This is our exit string. If the user puts in this string for the snapchat username, then the program prints out the best match and exits out of the loop.
For each additional matches information, your app will calculate a compatibility score between them and the user, using the users own information. This score should be updated as we gain information about the matches.
Calculate an initial compatibility score using the users name and the matches name. Concatenate the users first name and last name, and concatenate the matches first name and last name (e.g. Bob Jones becomes BobJones). Use the pseudocode below to create the letter share compatibility algorithm, which calculates a compatibility score by counting how many letters are shared between the names then dividing by the users name length.
for letter1 in userName
for letter2 in matchesName
if letter1 equals letter2
increase count
break
compatibility = count / length of users name
Update the compatibility score based on the users and matches birthdays. Dont forget to validate all birthdays.
If the inputted user was born 3 years of the original birthday, multiply current compatibility by 1% (1.01).
If the inputted user was born 2 months of the original birthday of the user, then multiply the current compatibility by 3% (1.03).
If the inputted user was born in the same part of the month 10 days of the original birthday of the user, then multiply the compatibility by 1% (1.01).
Update the compatibility score by comparing the favorite food, favorite movie, and favorite music genre of the user with the matches. Ignore the letter case when comparing if they are equal.
If their favorite food is the same, multiply by 2% (1.02).
If their favorite movie is the same, multiply compatibility by 3% (1.03).
If their favorite song genre is the same, multiply compatibility by 4% (1.04).
When asking for a matches snap score, if their snap score is equal to 0, display that the matches is a bot and restart execution from the top of the loop for a new matchee.
As the user enters matches, multiply the current compatibility score by 100 and store the highest compatibility score found so far, along with that matches snapchat username.
After the exit string is entered, display the best match and top compatibility score as a percentage.
Test Code:
Hello, user, please put in your snapchat username:
croisantboi23
Put in your first name:
Carl
Put in your last name:
Wheezer
Put in the year you were born:
2002
Put in your birthday month as an integer:
11
Put in your birthday day:
25
Put in your favorite food:
croissant
Put in your favorite movie:
JimmyNeutron
Put in your favorite song genre:
LlamaNoises
Please put in your snap score:
17832
Please put the snapchat username of an account:
jimmysmomxoxo
Put in their first name:
JimmysMom
Put in their last name:
Neutron
Put in the year they were born:
1986
Put in their birthday month as an integer:
3
Put in their birthday day:
17
Put in their favorite food:
croissant
Put in their favorite movie:
Holes
Put in their favorite song genre:
Country
Please put in their snap score:
12453
Please put the snapchat username of an account:
~801~
Your closest match is jimmysmomxoxo with a compatibility rating of 46.83%.
Sample Code w/ Text Inputs:
import java.util.Scanner;
public class DatingApp {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
System.out.println("Hello, user, please put in your snapchat username:");
System.out.println("Put in your first name:");
System.out.println("Put in your last name:");
System.out.println("Put in the year you were born:");
System.out.println("Put in your birthday month as an integer:");
System.out.println("Invalid birthday month. Please put in a birthday month between 1 and 12:");
System.out.println("Put in your birthday day:");
System.out.println("Invalid day. Please put in a valid day:");
System.out.println("Put in your favorite food:");
System.out.println("Put in your favorite movie:");
System.out.println("Put in your favorite song genre:");
System.out.println("Please put in your snap score:");
System.out.println("Please put the snapchat username of an account:");
System.out.println("Put in their first name:");
System.out.println("Put in their last name:");
System.out.println("Put in the year they were born:");
System.out.println("Put in their birthday month as an integer:");
System.out.println("Invalid birthday month. Please put in a birthday month between 1 and 12:");
System.out.println("Put in their birthday day:");
System.out.println("Invalid day. Please put in a valid day:");
System.out.println("Put in their favorite food:");
System.out.println("Put in their favorite movie:");
System.out.println("Put in their favorite song genre:");
System.out.println("Please put in their snap score:");
System.out.println("Bot has been detected. Do not consider dating this user.");
//Add a print statement in the form of
//"Your closest match is (closest match snapchat username) with a compatibility rating of (best compatibility)%."
scnr.close();
}
} ---------------------------------------------------------------------------------------------------- Code in JavaScript Only use Java Scanner Import No Specific Functions
-------------------------------------------------------------------------------------------------------
Thanks in advance for any help!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
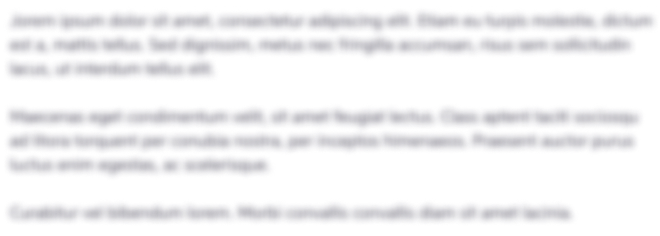
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started