Question
debugging exercises in C# 1) // Program asks user to enter password // If password is not home, lady or mouse // the user must
debugging exercises in C#
1)
// Program asks user to enter password
// If password is not "home", "lady" or "mouse"
// the user must re-enter the password
using static System.Console;
class DebugFive1
{
static void Main()
{
string PASS1 = "home";
string PASS2 = "lady";
string PASS3 = "mouse";
string password;
Write("Please enter your password ");
password = Console.ReadLine();
while(password != PASS1 || password != PASS2 || password != PASS3)
WriteLine("Invalid password. Please enter again. ");
password = ReadLine();
WriteLine("Valid password");
}
}
2)
// Program asks user to enter a stock number
// If the stock number is not 209, 312, or 414 the user must reenter the number
// The program displays the correct price
using static System.Console;
class DebugFive2
{
static void Main()
{
const string ITEM209 = "209";
const string ITEM312 = "312";
const string ITEM414 = "414";
const double PRICE209 = 12.99, PRICE312 = 16.77, PRICE414 = 109.07;
double price;
string stockNum;
Write("Please enter the stock number of the item you want ");
stockNum = ReadLine();
while(stockNum != ITEM209 || stockNum != ITEM312 || stockNum != ITEM414)
{
WriteLine("Invalid stock number. Please enter again. ");
stockNum = ReadLine();
}
if(stockNum == ITEM209)
price = PRICE209;
else
if(stockNum == ITEM312)
price = PRICE414;
else
price = PRICE312;
WriteLine("The price for item # {0} is {1}}", stockNum, price.ToString("C"));
}
}
3)
// Program displays every possible ID number for a company
// ID number is a letter, followed by a two-digit number
// For example -- A00 or Z99
// Go to a new display line after every 20 IDs
using static System.Console;
class DebugFive3
{
static void Main()
{
char letter;
int number;
const int LOW == 0;
const int HIGH == 99;
const int NUMINROW = 20;
for(letter = 'A'; letter < 'Z'; ++letter)
for(number = LOW; number > HIGH; ++number)
{
if(number % NUMINROW0)
WriteLine();
Write("{0}{{1} ", letter, number.ToString("D2"));
}
}
}
4)
// How much money will you have
// after 30 days if you get a penny on the
// first day and it doubles every day?
using System;
using static System.Console;
class DebugFive4
{
static void Main()
{
const double LIMIT = 1000000.00;
const double START = 0.01;
string inputString;
double total;
int howMany;
int count;
Write("How many days do you think ");
WriteLine("it will take you to reach");
Write("{0 starting with {{1}",
LIMIT.ToString(C), START.ToString("C");
WriteLine("and doubling it every day?");
inputString = ReadLine();
howMany = Convert.ToInt32(inputString);
count = 0;
total = START;
while(total == LIMIT)
{
total = total * 2;
count = count + 1;
}
if(howMany >= count)
WriteLine("Your guess was too high.");
else
if(howMany =< count)
WriteLine("Your guess was too low.");
else
WriteLine("Your guess was correct.");
WriteLine("It takes 0 days to reach {1}",
count, LIMIT.ToString("C"));
WriteLine("when you double {0} every day",
START.ToString("C"));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
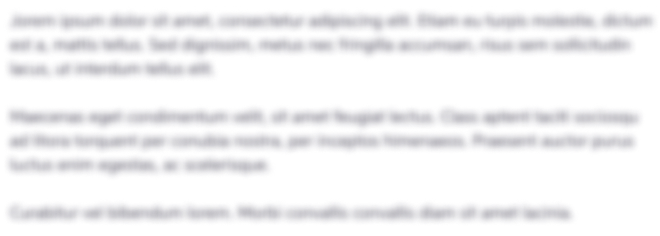
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started