Question
Define a class ArraySet that represents a set and implements the interface described in Segment 1.21 of the previous chapter. Use the class ResizableArrayBag in
Define a class "ArraySet" that represents a set and implements the interface described in Segment 1.21 of the previous chapter. Use the class "ResizableArrayBag" in your implementation. Then write a program that adequately demonstrates your implementation. HOWEVER, use the java.util.ArrayList class instead of the ResizableArrayBag class as mentioned in the project description.
Here is the interface code:
public interface SetInterface
{
/** Gets the current number of entries in this set.
@return The integer number of entries currently in the set. */
public int getCurrentSize();
/** Sees whether this set is empty.
@return True if the set is empty, or false if not. */
public boolean isEmpty();
/** Adds a new entry to this set, avoiding duplicates.
@param newEntry The object to be added as a new entry.
@return True if the addition is successful, or
false if the item already is in the set. */
public boolean add(T newEntry);
/** Removes a specific entry from this set, if possible.
@param anEntry The entry to be removed.
@return True if the removal was successful, or false if not. */
public boolean remove(T anEntry);
/** Removes one unspecified entry from this set, if possible.
@return Either the removed entry, if the removal was successful,
or null. */
public T remove();
/** Removes all entries from this set. */
public void clear();
/** Tests whether this set contains a given entry.
@param anEntry The entry to locate.
@return True if the set contains anEntry, or false if not .*/
public boolean contains(T anEntry);
/** Retrieves all entries that are in this set.
@return A newly allocated array of all the entries in the set. */
public T[] toArray();
} // end SetInterface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
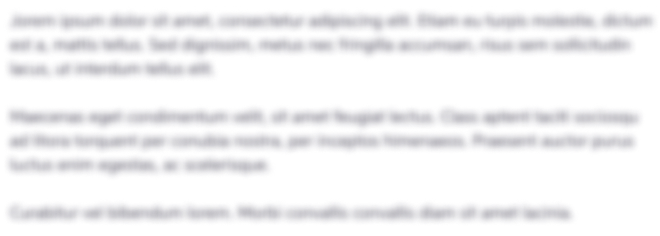
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started