Question
Define a class called Month. Your class will have one member variable of type int to represent a month (1 for January, 2 for February,
Define a class called Month. Your class will have one member variable of type int to represent a month (1 for January, 2 for February, and so forth). The complete class will include all the following member functions:
- a constructor to set the month using the first three letters in the name of the month.
- a constructor to set the month using an integer argument (1 for January, 2 for February, and so forth),
- a default constructor,
- an input function that reads the month as an integer,
- an input function that reads the month as the first three letters in the name of the month,
- an output function that outputs the month as an integer,
- an output function that outputs the first three letters in the name of the month,
- a member function that returns the next month as a value of type Month.
Embed the class definition in a test program.
Using the code:
Month.h:
#ifndef MONTH_H
#define MONTH_H
#include
#include
using namespace std;
class Month
{
public:
Month();
Month(int);
Month(string);
~Month();
void inputInt();
void inputString();
void outputInt();
void outputString();
Month nextMonth();
private:
int monthInt;
};
#endif
Month.cpp
#include "Month.h"
string Month_Int2String(int month_int) {
switch (month_int) {
case 1: return "Jan";
case 2: return "Feb";
case 3: return "Mar";
case 4: return "Apr";
case 5: return "May";
case 6: return "Jun";
case 7: return "Jul";
case 8: return "Aug";
case 9: return "Sep";
case 10: return "Oct";
case 11: return "Nov";
case 12: return "Dec";
default: return "Not Accepted";
}
}
int Month_String2Int(string month_str) {
if (month_str == "Jan")
return 1;
else if (month_str == "Feb")
return 2;
else if (month_str == "Mar")
return 3;
else if (month_str == "Apr")
return 4;
else if (month_str == "May")
return 5;
else if (month_str == "Jun")
return 6;
else if (month_str == "Jul")
return 7;
else if (month_str == "Aug")
return 8;
else if (month_str == "Sep")
return 9;
else if (month_str == "Oct")
return 10;
else if (month_str == "Nov")
return 11;
else if (month_str == "Dec")
return 12;
else {
cout << "Wrong, The program will be terminated" << endl;
system("pause");
exit(0);
}
}
Month::Month() {
// assign 1 to monthInt
}
Month::Month(int M) {
// assign M to monthInt, make sure that M is between 1 and 12
// if it's not, show an error Message
}
Month::Month(string Mstring) {
// call the function Month_String2Int
// assign the return value to monthInt
}
Month::~Month() { }
void Month::inputInt() {
// create an integer variable, call it M
// ask the user to enter a value of M
// assign M to monthInt, make sure that M is between 1 and 12
// if it's not, show an error Message
}
void Month::inputString()
{
// create a string variable, call it S
// ask the user to enter a value of S
// call the function Month_String2Int
// assign the return value to monthInt
}
void Month::outputInt() {
// print the monthInt value
}
void Month::outputString() {
// call the function Month_Int2String to print the month as a string
}
Month Month::nextMonth() {
if (this->monthInt == 12)
this->monthInt = 1;
else
this->monthInt = this->monthInt + 1;
return *(this);
}
Source.cpp
#include "Month.h"
void main() {
// create an object of Month test all the methods in Month class
system("pause");
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
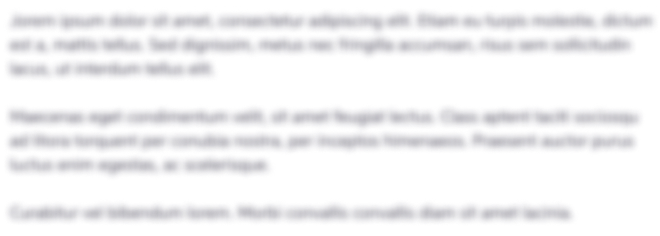
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started