Question
defined the class boxType by extending the definition of the class rectangleType. In this exercise, derive the class boxType from the class rectangleType, defined in
defined the class boxType by extending the definition of the class rectangleType. In this exercise, derive the class boxType from the class rectangleType, defined in Exercise 1, add the functions to overload the operators +,, *, ==, !=, <=, <, >=, >, and pre- and post-increment and decrement operators as members of the class boxType. Also overload the operators << and >>. Overload the relational operators by considering the volume of the boxes. For example, two boxes are the same if they have the same volume.
Write the definitions of the functions of the class boxType as defined above and then write a test program that tests various operations on the class. It is showing that I failed to overload the *, ++, +, --, -, ==, and != operators.
Most of the code is done I am not getting this part
This is not in the correct format because you need to pass three parameters. You need to add #include "rectangleType.h".
boxType::boxType(double side) { setDimension(side, side); }
For example
void boxType::setDimension(double l, double w, double h) { rectangleType::setDimension(l, w);
here is my code:
-------------------------boxType.cpp---
#include #include #include "boxType.h"
using namespace std;
boxType::boxType(double side) { setDimension(side, side); }
boxType::boxType() { length = 0; width = 0; }
boxType boxType::operator++() { //increment the length and width ++length; ++width;
return *this; //return the incremented value of the object }
boxType boxType::operator++(int u) { boxType temp = *this; //use this pointer to copy //the value of the object //increment the length and width length++; width++;
return temp; //return the old value of the object }
boxType boxType::operator--() { //Decrement the length and width assert(length != 0 && width != 0); --length; width = length;
return *this; //return the incremented value of the object }
boxType boxType::operator--(int u) { boxType temp = *this; //use this pointer to copy //the value of the object
//Decrement the length and width assert(length != 0 && width != 0); length--; width = length;
return temp; //return the old value of the object }
boxType boxType::operator+ (const boxType& box) const { boxType tempBox;
tempBox.length = length + box.length; tempBox.width = tempBox.length;
return tempBox; }
boxType boxType::operator- (const boxType& box) const { boxType tempBox;
assert(length >= box.length && width >= box.width);
tempBox.length = length - box.length; tempBox.width = tempBox.length;
return tempBox; }
boxType boxType::operator*(const boxType& box) const { boxType tempBox;
tempBox.length = length * box.length; tempBox.width = tempBox.length;
return tempBox; }
bool boxType::operator== (const boxType& box) const { return (area() == box.area()); }
bool boxType::operator!= (const boxType& box) const { return (area() != box.area()); }
bool boxType::operator<= (const boxType& box) const { return (area() <= box.area()); }
bool boxType::operator< (const boxType& box) const { return (area() < box.area()); }
bool boxType::operator>= (const boxType& box) const { return (area() >= box.area()); }
bool boxType::operator> (const boxType& box) const { return (area() > box.area()); }
ostream& operator<<(ostream& osObject, const boxType& box) { osObject << "Side = " << box.length;
return osObject; }
istream& operator>>(istream& isObject, boxType& box) { isObject >> box.length; box.width = box.length; return isObject; }
------------------------------boxType.h----------
#ifndef H_boxType #define H_boxType
#include #include "rectangleType.h" using namespace std;
class boxType : public rectangleType { //Overload the stream insertion and extraction operators friend ostream& operator<<(ostream&, const boxType &); friend istream& operator>>(istream&, boxType &);
public: //Overload the arithmetic operators boxType operator + (const boxType &) const; boxType operator - (const boxType &) const; boxType operator * (const boxType&) const;
//Overload the increment and decrement operators boxType operator ++ (); //pre-increment boxType operator ++ (int); //post-increment boxType operator -- (); //pre-decrement boxType operator -- (int); //post-decrement
//Overload the relational operators bool operator == (const boxType&) const; bool operator != (const boxType&) const; bool operator <= (const boxType&) const; bool operator < (const boxType&) const; bool operator >= (const boxType&) const; bool operator > (const boxType&) const;
//constructors boxType(); boxType(double side); };
#endif
-----------------------rectangleType.cpp-------------------
#ifndef H_boxType #define H_boxType
#include #include "rectangleType.h" using namespace std;
class boxType : public rectangleType { //Overload the stream insertion and extraction operators friend ostream& operator<<(ostream&, const boxType &); friend istream& operator>>(istream&, boxType &);
public: //Overload the arithmetic operators boxType operator + (const boxType &) const; boxType operator - (const boxType &) const; boxType operator * (const boxType&) const;
//Overload the increment and decrement operators boxType operator ++ (); //pre-increment boxType operator ++ (int); //post-increment boxType operator -- (); //pre-decrement boxType operator -- (int); //post-decrement
//Overload the relational operators bool operator == (const boxType&) const; bool operator != (const boxType&) const; bool operator <= (const boxType&) const; bool operator < (const boxType&) const; bool operator >= (const boxType&) const; bool operator > (const boxType&) const;
//constructors boxType(); boxType(double side); };
#endif
-----------------------------rectangleType.h-------------------
#ifndef H_rectangleType
#define H_rectangleType
#include
using namespace std;
class rectangleType
{
public:
//Overload the stream insertion and extraction operators
friend ostream& operator<<(ostream&, const rectangleType& rectangle);
friend istream& operator>>(istream&, rectangleType& rectangle);
//Overload the arithmetic operators
rectangleType operator+(const rectangleType&);
rectangleType operator-(const rectangleType&);
rectangleType operator*(const rectangleType&);
//Overload the increment and decrement operators
rectangleType operator++();
//pre-increment
rectangleType operator++(int);
//post-increment
rectangleType operator--();
//pre-decrement
rectangleType operator--( int);
//post-decrement
//Overload the relational operators
bool operator==(
const rectangleType&);
bool operator!=(
const rectangleType&);
bool operator<=(
const rectangleType&);
bool operator<(
const rectangleType&);
bool operator>=(
const rectangleType&);
bool operator>(
const rectangleType&);
void setDimension(double l, double w);
double getLength() const;
double getWidth() const;
double area() const;
double perimeter() const;
rectangleType();
rectangleType(double l, double w);
protected:
double length;
double width;
};
#endif
-----------------------------------main.cpp-----------------------------
#include #include "rectangleType.h" #include "boxType.h"
using namespace std;
int main() { rectangleType rectangle1(10, 5); rectangleType rectangle2(8, 7); rectangleType rectangle3; rectangleType rectangle4;
cout << "rectangle1: " << rectangle1 << endl; cout << "rectangle2: " << rectangle2 << endl;
rectangle3 = rectangle1 + rectangle2; cout << "rectangle3: " << rectangle3 << endl;
rectangle4 = rectangle1 * rectangle2; cout << "rectangle4: " << rectangle4 << endl;
if (rectangle1 > rectangle2) cout << "Area of rectangle1 is greater than the area " << "of rectangle2 ." << endl; else cout << "Area of rectangle1 is less than or equal to the area " << "of rectangle2 ." << endl;
rectangle1++;
cout << "After increment the length and width of " << "rectangle1 by one unit, rectangle1: " << rectangle1 << endl;
rectangle4 = ++rectangle3;
cout << "New dimension of rectangle3: " << rectangle3 << endl; cout << "New dimension of rectangle4: " << rectangle4 << endl;
boxType box1(10); boxType box2(8); boxType box3; boxType box4;
cout << "box1: " << box1 << endl; cout << "box2: " << box2 << endl;
box3 = box1 + box2; cout << "box3: " << box3 << endl;
box4 = box1 * box2; cout << "box4: " << box4 << endl;
if (box1 > box2) cout << "Area of box1 is greater than the area " << "of box2 ." << endl; else cout << "Area of box1 is less than or equal to the area " << "of box2 ." << endl;
box1++;
cout << "After increment the side of " << "box1 by one unit, box1: " << box1 << endl;
box4 = ++box3;
cout << "New dimension of box3: " << box3 << endl; cout << "New dimension of box4: " << box4 << endl;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
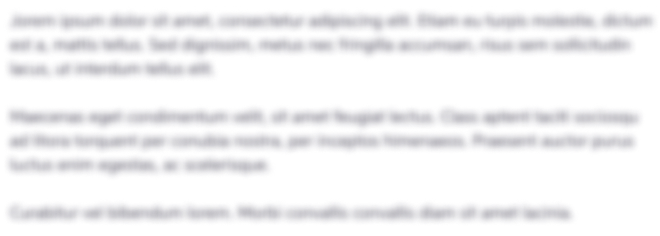
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started