Describe the four access regimes from public to private that may be applied to Java fields and methods. Why are they useful? [4 marks] (b)
Describe the four access regimes from public to private that may be applied to Java fields and methods. Why are they useful? [4 marks] (b) When you extend a class, the constructor for your new class will reference the constructor of the parent class, and this latter constructor may have any of the four possible access regimes. Comment on the consequences of each of the four possibilities. [4 marks] (c) If the only constructor for a class is marked as private, is it ever possible to have an instance of that class or any subclass of it? Explain why or why not. [2 marks]
T). At each time step all cells transform simultaneously. If a cell is dead, it becomes alive if it had (just before this time step) exactly three live neighbours. If it is alive, it becomes dead unless it has two or three live neighbours. In this question, locations beyond a 1000 1000 board are to be treated as permanently dead. (a) Show how to set up a simple Java 2-dimensional array of boolean values to represent a Life Board, with all cells initially "dead". [2 marks] (b) For a location (i, j) on the board, give code that will decide whether the next state of that cell should be alive or dead. Make it clear how your code copes if the cell is at the boundary of the board. [4 marks] (c) Referring to part (b), write code that takes one board representing the current state of the game and fills in a second board-array with the state arrived at after one time step. What would happen if instead of using two arrays you wrote the new cell state directly back, using just a single copy of the board? [3 marks] (d) Re-work your solution to part (c) so that you can perform a time step using just one board. You may need to use a 1000-element vector to store information in a way that makes the update safe. [7 marks] (e) All the code you have written so far uses an array of boolean values. Some programmers would instead use an array of int values and treat each of the 32 bits in each int as giving the status of a cell. Suppose you have a 2-dimensional array of integers of size 1024 by 32 (that size is chosen so the array of integers may be viewed as a 1024 by 1024 array of bits): give code to retrieve a bit from a given position.
A company has fired one of its senior staff after an incident that they claimed was an accident, but where the directors suspected malice. The company's auditors have suggested a review of the company's systems to mitigate 'the insider threat', and your advice has been sought. The directors want to be able to assure the shareholders that they are taking all reasonable steps to limit the damage that staff could do to affect the company's share price, whether accidentally or otherwise. (a) Describe a multilevel security policy briefly, explaining what sort of harms it seeks to prevent, and how. [5 marks] (b) Describe a separation-of-duty security policy briefly, explaining what sort of harms it seeks to prevent, and how. [5 marks] (c) Describe one approach to minimising the risk of user error that is based primarily on psychology. [5 marks] (d) Introducing architectural changes to the company's information systems will take time, while changes to user interfaces and workflows are often simple to implement. Which psychological approaches might be adopted more quickly, and what might you expect to go wrong? [5 marks]
Consider the following grammar: ce, cats} V {saw, grinned} (a) The grammar can be used to generate the following sentences: (i) Alice saw cats (ii) Cats Alice saw grinned Draw derivation trees for both of these sentences. [2 marks] (b) What is the longest sentence that can be generated by the grammar? Describe this sentence. [2 marks] (c) Is the language generated by the grammar a regular language? Provide a proof for your answer. [8 marks] (d) A psycho-linguistic experiment shows that, by the 2nd word in the sentence, Part (a)(ii) is harder to process than the sentence Part (a)(i). give hypothesized that a speaker's short-term memory functions as a stack. Explain how this hypothesis might account for the experimental results by drawing the stack arising from a top-down parse of the two sentences. [4 marks] (e) How might the sentence in Part (a)(ii) be altered so that it has the same meaning but is easier to process? Explain your reasoning.
A Boolean satisfiability problem has four variab x3) (x2 x3) (x1 x2 x4). (1) The aim is to find assignments to the variables such that f is true under the usual rules for Boolean operations. This question addresses the use of more general constraint satisfaction to solve this problem. (a) Give a general description of a constraint satisfaction problem (CSP). [3 marks] (b) Explain how a Boolean satisfiability problem in CNF form and with n variables can be converted to a CSP, also having n variables and having a suitable constraint for each clause. Illustrate your answer using the 4-variable formula f in (1). [3 marks] (c) Explain, again using a constraint corresponding to a clause from (1), how general constraints can be converted to binary constraints. Provide a graph illustrating the problem from (1) after it has been converted to a CSP with only binary constraints. [4 marks] (d) Explain, how forward checking works in the context of a general CSP. How does this benefit a CSP solver? [3 marks] (e) Using the CSP equivalent you developed for (1), with only binary constraints, demonstrate how forward checking works using the sequence of assignments x1 = F, x2 = F, x4 = T. [5 marks] (f ) How would you expect the solution obtained when applying forward checking to be affected if constraints were allowed to propagate more widely? [2 marks] Computer Graphics and Image Processing
(a) Describe an algorithm to draw a straight line using only integer arithmetic.
You may assume that the line is in the fifirst octant, that the line starts and
ends at integer co-ordinates, and that the function setpixel(x, y) turns on the
pixel at location (x, y). [8 marks]
(b) Describe Douglas and Pucker's algorithm for removing superflfluous points from
a line chain. [10 marks]
(c) Under what circumstances would it be sensible to employ Douglas and Pucker's
algorithm?
(a) Brieflfly explain the difffferences between combinational and sequential logic.
[2 marks]
(b) With the aid of appropriate diagrams, brieflfly explain the operation of Moore
and Mealy fifinite state machines and highlight their difffferences. [6 marks]
(c) The state sequence for a binary counter is as follows:
The British Computer Society Code of Conduct has four sections. What kind
of professional conduct does each section cover, and how does each of these
kinds of conduct benefifit the profession and its members? [8 marks]
(b) True or False questions:
(i) A User can provide occasional use of the University computer system for
a friend who is a temporary visitor.
(ii) Circumstances that mitigate minor infractions of the rules promulgated
by the Information Technology Syndicate include, among other things,
inebriation.
(iii) Appropriate use of the Cambridge University Data Network (CUDN)
means bona fifide academic activity plus a low level for private purposes.
(iv) Small amounts of commercial activity are acceptable as long as the User
is acting in a private capacity.
[4 marks]
(c) The IT industry is increasingly aware of its own environmental impact.
Describe at least one environmental problem to which the industry contributes
and how, as an IT professional, you can help to solve this problem. [4 marks]
(d) "Social engineering is a greater threat to computer security than computer
cracking software." What is social engineering and what measures can be
taken to guard against it? [2 marks]
(e) What is copyleft and how is it used to protect free, open-source, software?
3File Input & Data Processing Reading data from a file is often done in order to process and aggregate it to get ad- ditional results. In this activity you will read in data from a file containing win/loss data from the 2011 Major League Baseball season. Specifically, the file data/mlb_nl_2011.txt contains data about each National League team. Each line contains a team name fol- lowed by the number of wins and number of losses during the 2011 season. You will open this file and process the information to output a list of teams followed by their win percentage (number of wins divided by the total number of games) from highest to lowest. Instructions
Instructions 1. Open the mlb.c sOurce files. Much of the program has already been provided for you, including a convenience function to sort the lists of teams and their win percentages as well as a function to output them. 2. Add code to open the data file and read in the team names, wins and losses and populate the teams and winPercentages[]
winPercentages arrays with the appropriate data 3. Call the sort and output functions to sort and display your results 4. Answer the questions on your worksheet and demonstrate your working program to a lab instructor mlb nl_2011.txt file Braves 89 73 Phillies 102 60 Nationals 80 81 Mets 77 85 Marlins 72 90 Brewers 96 66 Cardinals 90 72 Reds 79 83 Pirates 72 90 Cubs 7191
mlb.c #include #include #include void sortMLB(char *teams, double *winPerc, int numTeams); void printMLB(char **teams, double *win Perc, int numTeams); int maingint argc, char **argv) { int const maxSize = 50 int const numTeams = 16; char filePath[] = "data/mib nl_2011.bxt"; char tempBuffer[ 100); int i; char **teams = (char *)malloc(sizeof(char ) * numTeams); double win Percentages = (double "malloc(sizeof(double) * numTeams); for (i = 0; i< numTeams; i++) { teamsi] = (char ")malloc(sizeof(char) * maxSize);
Write Java program to find the factorial of number. Input number should be given by user. ) Write Java program to read a file content line by line.
Simple natural language words are very basic index keys for retrieval systems. Since the topics for which users seek to retrieve documents are often complex, it is frequently claimed that simply looking for matches on any subset of a set of submitted single-word keys is not a discriminating enough approach to retrieval. Describe three different ways in which the relations between words can be defined so as to form compound index terms. You should describe in detail how each is implemented and what its advantages and disadvantages are by comparison with (a) single terms, and (b) the other two types of compound. [6 marks for each method] Which would you implement in a general-purpose system, and why? [2 marks] 7 Artificial Intelligence This question considers the monkey-and-bananas problem, in which there is a monkey in a room with some bananas hanging out of reach from the ceiling, but a box is available that will enable the monkey to reach the bananas if he climbs onto it. Initially the monkey is at location A, the bananas at B and the box at C. The monkey and box have height x, but if the monkey climbs onto the box he will have height y, the same as the bananas. The actions available to the monkey include Go from one place to another, Push an object from one place to another, Climb onto an object, and Grasp an object. Grasping results in holding the object if the monkey and object are in the same place at the same height. (a) Write the initial state description using a representation of your choice. [4 marks] (b) Write definitions of the four actions, providing at least some obvious preconditions, additions and deletions. [10 marks] (c) Suppose the monkey wants to fool the observers, who have gone to lunch, by grabbing the bananas but leaving the box in its original place. Write this as a goal (but not assuming the box is necessarily at location C) in the language of situation calculus. [3 marks] (d) If the box is filled with bricks, its position will remain the same when the Push operator is applied. Is this an example of the frame problem or the circumscription problem? Justify your answer. [3 marks] 8 Neural Computing (a) (i) Define generalization in neural networks that learn from training data, and then are tested on new data. Why should not all the available data be used in the training set? [4 marks] (ii) Draw a simple connectivity diagram that illustrates the idea of lateral inhibition in a competitive neural network. [2 marks] (iii) With another diagram showing plots for input and output, illustrate how lateral inhibition in such a competitive network sharpens any input signal by effectively amplifying its first derivative. [2 marks] (iv) What class of multi-layer neural network can be used to overcome the mathematical difficulties caused by intrinsic non-orthogonality of representation in many sensory and control systems? (b) The study of neurological trauma to the brain gives clues about its modular organisation and specialisations of function, which may reveal some computational principles. (i) What two fundamental principles of brain function did Karl Lashley's neurological research seem to reveal? [2 marks] (ii) What are generally the differences between recovery prospects after a sudden brain trauma (in which all the damage is done at once), versus the same damage done more gradually (e.g. by a growing tumour)?You have designed a digital water-level display installed on the River Cam. A sensor measures the current height of a small floating ball once every minute. In order to reduce the fluctuations that small waves would otherwise cause in the displayed value, you implemented a digital filter yi = 0.8yi1 + 0.2xi , where the xi are the measured and the yi are the displayed water levels.
A program is required to draw an arc from (0, 1) to (1, 0) of the circle centred t the origin with unit radius. (a) One approach would be to draw a segment of the cubic Overhauser curve defined by (1, 0), (0, 1), (1, 0) and (0, 1). (i) Explain how a segment of an Overhauser curve in general can be represented as an Hermite cubic and so as a Bezier cubic. [4 marks] (ii) Derive the formula for the resulting Bezier curve, P(t). [3 marks] (iii) Calculate the coordinates of P( 1 2 ). How large is the error? [Hint: 2 1.414.] [3 marks] (b) Calculate revised control points for the Bezier curve so that it models the circular arc more accurately. [4 marks] (c) Describe in outline an alternative way of efficiently drawing the arc by calculating the pixels that lie on it directly.
(a) State what is meant by a directed graph and a strongly connected component.
Illustrate your description by giving an example of such a graph with 8 vertices
and 12 edges that has three strongly connected components. [5 marks]
(b) Describe, in detail, an algorithm to perform a depth-fifirst search over such a
graph. Your algorithm should attach the discovery and fifinishing times to each
vertex and leave a representation of the depth-fifirst spanning tree embedded
within the graph. [5 marks]
Step by Step Solution
3.45 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
Lets break down and answer each part of the question a Access Regimes in Java In Java there are four access modifiers that can be applied to fields an...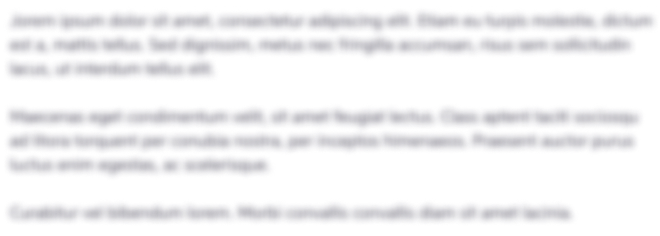
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started