Question
Description: In this homework, you will replicate the GUI you made for homework #3 utilizing FXML and CSS style sheets.You should use the Maven JavaFX
Description:
In this homework, you will replicate the GUI you made for homework #3 utilizing FXML and CSS style sheets.You should use the Maven JavaFX project found in the sample code section on our BB course page. Remember to change the artifact ID in the POM file to HW3Spring23.
You must have at least one of each: a controller class, a .fxml file and a .css file. The user interface must render only using this approach. The appropriate GUI elements must be clickable and have methods that are called when the user interacts with it. These methods must be defined in a controller class and attached to the widgets in the .fxml files.
If you submit a working version of homework #3 that creates and manages the GUI with fxml instead of programmatically, you will receive extra credit on this homework. If you do not want to submit a working version of homework #3 using fxml, when widgets are clicked you can simply have the methods attached print something to the terminal. We will only concentrate on the successful creation of a user interface using fxml and css.
Below, is the write up of homework #3 for your reference....
Implementation Details (this is the description from homework #3):
You will use the Maven JavaFX Project provided in the sample code section of our BB course page. You will need to change the in the pom file to fxmlhw. You will also need to use the documentation for JavaFX BorderPane, VBox, TextField and Button. It can be found here: https://docs.oracle.com/javase/8/javafx/api/index.html?help-doc.html
First, change the title of the app to "(your first and last name) Homework 3". Your GUI should have two buttons (use the JavaFX class Button) with the labels "button 1" and "button 2" as well as two text fields (use the JavaFX class TextField). Utilizing a BorderPane (a class in JavaFX), set one TextField to the center and the other TexField to the right in the BorderPane. Set the buttons in an VBox to the left inside the BorderPane. The TextField in the center should display prompt text letting the user know what to do with it; the prompt should say "enter text here then press button 1". You will need to look at the documentation for TextField to find the proper method.
The TextField on the right should not be editable (user can not type in it). It should start out displaying the message "final string goes here". This string should NOT be a prompt. Consult the documentation to find the correct methods.
Attach an EventHandler to "button 1" utilizing an anonymous class. When clicked:
you will get the text from the text field in the center,
concatenate that text with the string " : from the center text field!"
set the text field to the right with the new string disable button 1 so it can not be pressed
change the string displayed on button one to "pressed" (instead of "button 1").
Attach an EventHandler to "button 2" utilizing a lambda expression. When clicked:
you will clear the text from both TextFields,
replace the string "final string goes here" in the TextField to the right
enable the first button so it can be pressed again
replace the string displayed on that button with "button one".
You will notice when you complete the above, that your user interface does not look very good. Look into the documentation for BorderPane and figure out how to improve the look of your app and implement it.
BELOW WAS MY CODE FOR HOMEWORK 3:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.scene.control.Button; import javafx.scene.control.TextField; import javafx.scene.layout.BorderPane; import javafx.scene.text.Font; import javafx.scene.text.Text;
public class JavaFXTemplate extends Application {
public static void main(String[] args) { launch(args); } //feel free to remove the starter code from this method @Override public void start(Stage primaryStage) throws Exception { //set window title primaryStage.setTitle("Sriya Alla Homework 3"); // create the buttons Button button1 = new Button("Button 1"); Button button2 = new Button("Button 2"); // create the text fields TextField textField1 = new TextField(); textField1.setPromptText("Enter text here then press Button 1"); // set the prompt text TextField textField2 = new TextField("final string goes here"); // set the initial text textField2.setEditable(false); // make the text field non-editable // create the VBox to hold the buttons VBox buttonBox = new VBox(); buttonBox.getChildren().addAll(button1, button2); // create the BorderPane and set the VBox to the left, and the text fields to the center and right BorderPane borderPane = new BorderPane(); borderPane.setLeft(buttonBox); borderPane.setCenter(textField1); borderPane.setRight(textField2); borderPane.setStyle("-fx-background-color: #FFB6C1;"); textField1.setFont(new Font("Impact", 20)); // change the font to Impact, size 20 textField2.setFont(new Font("Comic Sans MS", 20)); // change the font to Comic Sans MS, size 20 // create the scene and set it on the stage and show the window Scene scene = new Scene(borderPane, 700,700); primaryStage.setScene(scene); primaryStage.show(); // EventHandler to "button 1" utilizing an anonymous class button1.setOnAction(event -> { String text = textField1.getText(); // get the text from the center TextField String newString = text + " : from the center text field!"; // concatenate with new string textField2.setText(newString); // set the text field on the right to the new string button1.setDisable(true); // disable button 1 button1.setText("pressed"); // change the label of button 1 to "pressed" }); // Attach event handler to button 2 using a lambda expression button2.setOnAction(event -> { textField1.setText(""); textField2.setText("new final string"); // Replace string "final string goes here" in the TextField to the right button1.setDisable(false); // Enable button 1 so it can be pressed again button1.setText("Button 1"); // Replace string displayed on button 1 with "button one" }); }
}
Please give me a good explanation and thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
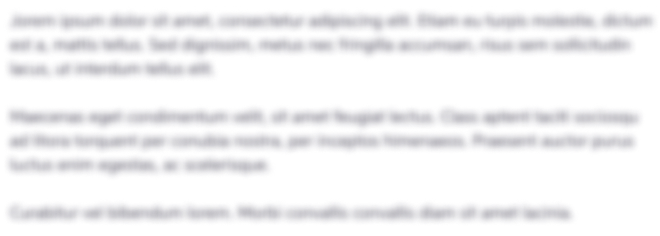
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started