Question
Description of Programming Tasks Task 1 - Empty Classroom Seating Chart Write a program that prints a seating chart (similar to that from the Project
Description of Programming Tasks
Task 1 - Empty Classroom Seating Chart Write a program that prints a seating chart (similar to that from the Project 1a Description) for an empty classroom of size specified by the user. Have the user enter in both the number of rows and the number of seats per row. Make sure the user input is reasonable, by checking that the number of rows is 26 or less, that the number of seats per row not greater than 30, and that both are at least 1. Then, print to screen a carefully formatted seating chart where each row is represented with a letter, and every seat in each row is uniquely identified with a letter and a number (see the sample output section below for examples). Some important considerations: The format of your seating chart must match the sample output precisely (including whitespaces) in order to pass the test cases Capital letters. Two digits for all numbers (e.g. A05, K14, W24, etc.). Single space after each seat (including the last seat per row, before the new line character " "), which means each seat uses a total of 4 characters. The first row is at the bottom of the seating chart, and thus must be printed last. To achieve this task for general input from the user, a nested loop must be used. However, arrays and/or structs must not be used as they are beyond the scope of the course to this point.
Task 2 - Every-Other Seating Chart Extend your program to also print out a seating chart where every other seat is taken by a student. Represent a taken seat as an "X". To keep the formatting neat, make sure to still reserve 4 total characters for each seat, i.e. if seat B12 is taken, replace it with an "X" that has one space before the "X" and two spaces after (again, see the sample output section below for examples). Additionally, report the number of students that can be seated using this arrangement. One important consideration: The first seat, A01, is taken, in addition to every other seat in row A. Then, the taken seats in row B are offset from row A, and the pattern repeats for all the rows.
Task 3 - More-Complicated Seating Chart Extend your program to also print out a seating chart that matches the pattern from the Project 1a filled seating chart. Use the logic you developed for your Project 1a program, wrap it into appropriate loop(s), and modify the functionality for general user input for number of rows and seats per row (again, see the sample output section below for examples). Additionally, report the number of students that can be seated using this arrangement. Sample Input/Output The following are sample executions of the program with mixed input and output. Recall: the program should only take in two integer inputs for the number of rows and number of seats per row.
Enter number of rows: 11
Enter number of seats per row: 16
Empty Classroom Seating Chart: K01 K02 K03 K04 K05 K06 K07 K08 K09 K10 K11 K12 K13 K14 K15 K16 J01 J02 J03 J04 J05 J06 J07 J08 J09 J10 J11 J12 J13 J14 J15 J16 I01 I02 I03 I04 I05 I06 I07 I08 I09 I10 I11 I12 I13 I14 I15 I16 H01 H02 H03 H04 H05 H06 H07 H08 H09 H10 H11 H12 H13 H14 H15 H16 G01 G02 G03 G04 G05 G06 G07 G08 G09 G10 G11 G12 G13 G14 G15 G16 F01 F02 F03 F04 F05 F06 F07 F08 F09 F10 F11 F12 F13 F14 F15 F16 E01 E02 E03 E04 E05 E06 E07 E08 E09 E10 E11 E12 E13 E14 E15 E16 D01 D02 D03 D04 D05 D06 D07 D08 D09 D10 D11 D12 D13 D14 D15 D16 C01 C02 C03 C04 C05 C06 C07 C08 C09 C10 C11 C12 C13 C14 C15 C16 B01 B02 B03 B04 B05 B06 B07 B08 B09 B10 B11 B12 B13 B14 B15 B16 A01 A02 A03 A04 A05 A06 A07 A08 A09 A10 A11 A12 A13 A14 A15 A16
Every-Other Seating Chart:
X K02 X K04 X K06 X K08 X K10 X K12 X K14 X K16 J01 X J03 X J05 X J07 X J09 X J11 X J13 X J15 X X I02 X I04 X I06 X I08 X I10 X I12 X I14 X I16 H01 X H03 X H05 X H07 X H09 X H11 X H13 X H15 X X G02 X G04 X G06 X G08 X G10 X G12 X G14 X G16 F01 X F03 X F05 X F07 X F09 X F11 X F13 X F15 X X E02 X E04 X E06 X E08 X E10 X E12 X E14 X E16 D01 X D03 X D05 X D07 X D09 X D11 X D13 X D15 X X C02 X C04 X C06 X C08 X C10 X C12 X C14 X C16 B01 X B03 X B05 X B07 X B09 X B11 X B13 X B15 X X A02 X A04 X A06 X A08 X A10 X A12 X A14 X A16 *88 students have seats in this arrangement* More-Complicated Seating Chart: X X K03 X X K06 X X K09 X X K12 X X K15 X J01 X J03 X J05 X J07 X J09 X J11 X J13 X J15 X I01 X X I04 I05 X X I08 I09 X X I12 I13 X X I16 X X H03 X X H06 X X H09 X X H12 X X H15 X G01 X G03 X G05 X G07 X G09 X G11 X G13 X G15 X F01 X X F04 F05 X X F08 F09 X X F12 F13 X X F16 X X E03 X X E06 X X E09 X X E12 X X E15 X D01 X D03 X D05 X D07 X D09 X D11 X D13 X D15 X C01 X X C04 C05 X X C08 C09 X X C12 C13 X X C16 X X B03 X X B06 X X B09 X X B12 X X B15 X A01 X A03 X A05 X A07 X A09 X A11 X A13 X A15 X
*100 students have seats in this arrangement*
Enter number of rows: 5
Enter number of seats per row: 7
Empty Classroom Seating Chart:
E01 E02 E03 E04 E05 E06 E07 D01 D02 D03 D04 D05 D06 D07 C01 C02 C03 C04 C05 C06 C07 B01 B02 B03 B04 B05 B06 B07 A01 A02 A03 A04 A05 A06 A07 Every-Other Seating Chart: X E02 X E04 X E06 X D01 X D03 X D05 X D07 X C02 X C04 X C06 X B01 X B03 X B05 X B07 X A02 X A04 X A06 X *
18 students have seats in this arrangement*
More-Complicated Seating Chart:
X X E03 X X E06 X D01 X D03 X D05 X D07 C01 X X C04 C05 X X X X B03 X X B06 X A01 X A03 X A05 X A07
*20 students have seats in this arrangement*
Enter number of rows: 30 Too many rows! Max. number of rows is 26. Enter number of rows: 0 Number of rows must be at least 1. Enter number of rows: 15 Enter number of seats per row: 50 Too many seats per rows! Max. number of seats per row is 30. Enter number of rows: 11 Enter number of seats per row: 0 Number of seats per rows must be at least 1.
In C Programming!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
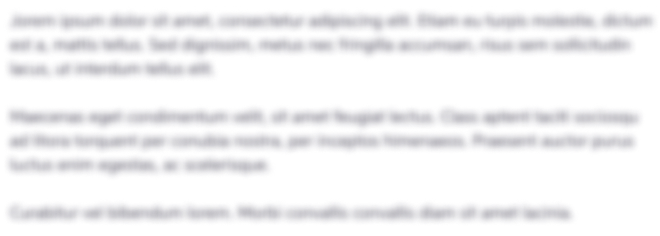
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started