Question
Description The goal of the project is to design and implement a basic media library system in which administrators (you) can inquire the current inventory,
Description The goal of the project is to design and implement a basic media library system in which administrators (you) can inquire the current inventory, and add or remove items. These items are identified either as books or movies. The media library is organized as follows. Each entry in the library can be identified by its media type (Movie or Book), its Title, its Reference, and its Price. A sample media library comprised of 9 items is given in Table 1.
Reference Media Title Price TU2RL012 Movie 2001: A Space Odyssey $11.99 GV5N32M9 Book A Brief History of Time $10.17 1DB6HK3L Movie North by Northwest $8.99 PO5T7Y89 Movie The Good, The Bad and The Ugly $9.99 TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 2FG6B3N9 Movie Gone with the Wind $4.99 6Y9OPL87 Book Gone with the Wind $7.99 Table 1: A sample media library. In addition, a book media item includes information about by its Author, while a movie media item includes information about its Director and Lead Actor. These additional information are provided in Table 2. Reference Author- Book Director- Movie Main Actor- Movie TU2RL012 Stanley Kubrick Keir Dullea GV5N32M9 Stephen Hawking 1DB6HK3L Alfred Hitchcock Cary Grant PO5T7Y89 Sergio Leone Clint Eastwood TR3FL0EW Paulo Coelho F2O9PIE9 Friedrich Nietzsche R399CED1 Richard Bach 2FG6B3N9 Victor Fleming Vivien Leigh 6Y9OPL87 Margarett Mitchell Table 2: Additional information for the sample media library.
1 The project must include three files:
1. MediaItem.py file/module that contains the user-defined type (object data) MediaItem.
2. inventory.py file/module that contains all the necessary functions to operate the inventory.
3. media-store.py file containing the main program.
At the first execution of the program media-store.py, the output includes a menu containing 9 options:
Welcome to BestMedia ====================
Menu ====
1-List Inventory
2-Info Inventory
3-List of All Books
4-List of All Movies
5-Item Description
6-Remove Item
7-Add Item
8-Set Maximum Price
0-Exit
Enter Command:
Once option 0 (for Exit) is selected, the program stops. All the options will be reviewed below. The project is designed to be incremental, you can then debug, test and run your code after each new task/option is implemented. After Task 1 done all the other Tasks/options can be completed in any order. Do not forget to comment your code. Make sure you obtain the exact same output for the exact same input for the examples (this includes syntax, blank spaces, and skipping blank lines). Your program will also be tested with different inputs by the graders.
Option-1- [30pts] Let us first see what is happening when option 0 is selected. Welcome to BestMedia ==================== Menu ==== 1-List Inventory 2-Info Inventory 2 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 0 Goodbye! Let us then see what is happening when option 1 is selected. Enter Command: 1 Reference/Media/Title/Price (max=$100.0) --------------------------- TU2RL012 Movie 2001: A Space Odyssey $11.99 GV5N32M9 Book A Brief History of Time $10.17 1DB6HK3L Movie North by Northwest $8.99 PO5T7Y89 Movie The Good, The Bad and The Ugly $9.99 TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 2FG6B3N9 Movie Gone with the Wind $4.99 6Y9OPL87 Book Gone with the Wind $7.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: Here an inventory of the media store is displayed. We can see the references, media type, title and price of each items. The maximum price is set to $100 meaning that all the items with a price below $100 will be listed in the inventory. At the end the menu selection is printed again and the program is waiting for you to make another choice. What you need to implement: 1. In the media store.py file: (i) The welcoming message, (2) a call to a function initialize that will return a list of items (data objects of type MediaItem...explained further below), (3) a variable that keeps track of the maximum price (initialize to $100 at first), (4) a while loop that keeps printing the menu selection and asking the user to enter a command choice; this while loop will exit if the entry is 0 and print a Goodbye! message; the function to print the menu, display menu, is already provided to you in the file inventory.py; (5) the option 1 that contains a call to a function display (you can use as arguments the list of items and the maximum price). We note that the header of the file already 3 contains the instruction import inventory which will allow you to call the functions in the inventory file using the dot operator. 2. In the MediaItem.py file: A class that define the type data object MediaItem that includes the constructor (i.e. function init ). You will consider the following attributes: media, title, price, price, ref, director, lead actor, author. All the attributes can be initialized to None by default. 3. In the Inventory.py file: (1) the initialize function that creates and returns a list of MediaItem data objects. Ideally we would like to read all the inventory from a file (so we could easily consider 1000s of items if needed) but we will do that later in the semester. Here, you will need to fill up by hand (hard coded) all the attributes of the objects for our selected 9 items presented in Tables 1 and 2 (a bit long but you can cc-paste title, etc. from this pdf file). (2) The function display that displays the items as presented in the output example (you can use \t to separate each field of the items). We note that the header of the file contains the instruction from MediaItem import MediaItem which will allow you to use the MediaItem data type.
Option-2- [15pts]
Let us now see what is happening when option 2 is selected. Enter Command: 2 Inventory is worth $75.89 Most expensive item at $11.99 There are 5 Book(s), and 4 Movie(s) Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: The program is displaying some info about the inventory, the total value of all the media items, the price of the most expensive items, and the total number of books and movies found in the whole inventory. What you need to implement: 1. In the media store.py file: the option 2 that contains a call to a function info (you can use as arguments the list of items) 2. In the Inventory.py file: the function info that displays the items as presented in the output example. You will probably needs a for loop that scans through all members of the list of media items. To find the maximum price you could implement the linear search algorithm seen in class.
Option-3 and 4- [10pts]
Let us now see what is happening when option 3 and then option 4 are selected. 4 Enter Command: 3 Reference/Media/Title/Price (max=$100.0) --------------------------- GV5N32M9 Book A Brief History of Time $10.17 TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 6Y9OPL87 Book Gone with the Wind $7.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 4 Reference/Media/Title/Price (max=$100.0) --------------------------- TU2RL012 Movie 2001: A Space Odyssey $11.99 1DB6HK3L Movie North by Northwest $8.99 PO5T7Y89 Movie The Good, The Bad and The Ugly $9.99 2FG6B3N9 Movie Gone with the Wind $4.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: This is very similar to option 1 but either the media Book items or Movie items are displayed. In Option 3, and 4 you could use the function display that would need to be modified to include an additional argument about the nature of the media.
Option-5- [10pts]
Let us now see what is happening when option 5 is selected (here we do it three times). Enter Command: 5 5 Enter item reference: 1DB6HK3L Title: North by Northwest (Ref: 1DB6HK3L, Price: $8.99); Movie Director: Alfred Hitchcock; Lead Actor: Cary Grant Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 5 Enter item reference: R399CED1 Title: Jonathan Livingston Seagull (Ref: R399CED1, Price: $6.97); Author: Richard Bach Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 5 Enter item reference: ECE122ECE No such item found! Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: The program is asking the user to enter a particular reference and it will display all information about this item (or returned No such item found! if the item is not in the inventory). Book item will also include info about the author while Movie item will include info about the director and lead actor. What you need to implement: 1. In the media store.py file: the option 5 that could include a call to a function search item that returns the object media item if found (could return None if not found). Another function display item 6 that displays the information for the selected item as presented in the output example. 2. In the Inventory.py file: the two functions above.
Option-6- [10pts] Let us now see what is happening when option 6 is selected. Here we select option 6, follows by option 1, option 3 and option 2. Enter Command: 6 Enter item reference: GV5N32M9 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 1 Reference/Media/Title/Price (max=$100.0) --------------------------- TU2RL012 Movie 2001: A Space Odyssey $11.99 1DB6HK3L Movie North by Northwest $8.99 PO5T7Y89 Movie The Good, The Bad and The Ugly $9.99 TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 2FG6B3N9 Movie Gone with the Wind $4.99 6Y9OPL87 Book Gone with the Wind $7.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 3 Reference/Media/Title/Price (max=$100.0) --------------------------- TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 6Y9OPL87 Book Gone with the Wind $7.99 7 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 2 Inventory is worth $65.72 Most expensive item at $11.99 There are 4 Book(s), and 4 Movie(s) Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: The program is asking the user to enter a particular reference and the corresponding items will be removed from the inventory as seen in the example when you select option 1 the book A Brief History of Timeis not there anymore. It also disappears from the list of books (since it is a book). Option 2 can return the new info about the new inventory that now only contains 8 items. What you need to implement: 1. In the media store.py file: the option 6 that could include a call to a function search index item to return the index of the item to remove from the list. We have seen in class how the built-in del function can be used to then remove an item with a given index from a list (and left shift all the other items with higher indexes). 2. In the Inventory.py file: the method above.
Option-7- [10pts] Let us now see what is happening when option 7 is selected (we use it three times here, follows by option 1 and then 2) Enter Command: 7 Book or Movie? Book Enter Book Title: Hamlet Enter Book Reference: J45K99EE Enter Book Price: 4.59 8 Enter Author Name: William Shakespeare Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 7 Book or Movie? Movie Enter Movie Title: The Great Escape Enter Movie Reference: ER89QW43 Enter Movie Price: 7.99 Enter Director Name: John Sturges Enter Lead Actor Name: Steve McQueen Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 7 Book or Movie? Novel Wrong input! Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 1 Reference/Media/Title/Price (max=$100.0) --------------------------- TU2RL012 Movie 2001: A Space Odyssey $11.99 1DB6HK3L Movie North by Northwest $8.99 PO5T7Y89 Movie The Good, The Bad and The Ugly $9.99 TR3FL0EW Book The Alchemist $6.99 9 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 2FG6B3N9 Movie Gone with the Wind $4.99 6Y9OPL87 Book Gone with the Wind $7.99 J45K99EE Book Hamlet $4.59 ER89QW43 Movie The Great Escape $7.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 2 Inventory is worth $78.3 Most expensive item at $11.99 There are 5 Book(s), and 5 Movie(s) Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: For option 7, once you ask the user to enter which media to select (Book or Movie), you will need a new function create item that returns a new data object MediaItem and append it to the list (creating then a new inventory)
Option-8- [5pts]
Finally, let us now see what is happening when option 8 is selected (follow by option 1 here) Enter Command: 8 Enter maximum price (current=$100.0): 8.0 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 10 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: 1 Reference/Media/Title/Price (max=$8.0) --------------------------- TR3FL0EW Book The Alchemist $6.99 F2O9PIE9 Book Thus Spoke Zarathustra $7.81 R399CED1 Book Jonathan Livingston Seagull $6.97 2FG6B3N9 Movie Gone with the Wind $4.99 6Y9OPL87 Book Gone with the Wind $7.99 J45K99EE Book Hamlet $4.59 ER89QW43 Movie The Great Escape $7.99 Menu ==== 1-List Inventory 2-Info Inventory 3-List of All Books 4-List of All Movies 5-Item Description 6-Remove Item 7-Add Item 8-Set Maximum Price 0-Exit Enter Command: The user is asked to enter a new maximum price. Using option 1, the inventory will not display the items that have a higher price (items are not removed, just hidden, so it will not affect option 2, but will affect options 3 and 4).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
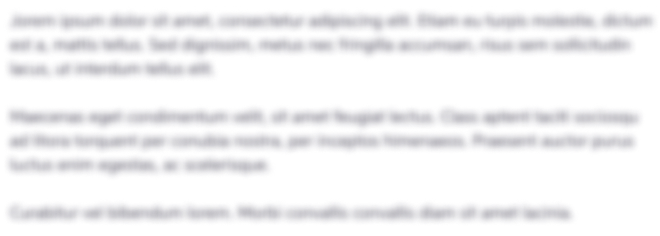
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started