Question
Description: This program will manage cars that go through a car rental lot Instructions: Move your class to separate CPP and h files. Create a
Description: This program will manage cars that go through a car rental lot
Instructions:
Move your class to separate CPP and h files.
Create a full constructor for your class.
Create a toString member function for objects of your class.
Create an equals member function based on the purpose of your class.
Check to make sure all identifying comments, class comments, and function comments are correct.
In the main source file prevent the user from entering a car that is already in the vector using the equals method to test.
It must provide a means for the user to add, edit, and delete cars.
Make sure the main code is in top-down format with work broken into separate functions, each function prototyped before main and defined after main.
Class Car should contain:
private:
int vin; string make; string model; double rate; int year; int numCars;
public:
Car(); Car(int vin, string make, string model, double rate, int year, int numCars); int getVin() const; void setVin(int vin); string getMake() const; void setMake(string make); string getModel() const; void setModel(string model); int getYear() const; void setYear(int year); double getRate() const; void setRate(double rate); void decrementNumCars();
What I have so far:
source.cpp:
#define MAX_CARS 20 #include
using namespace std;
int mainMenu(); void rentCar(vector
int choiceLI = 0; do { choiceLI = mainMenu(); //if choice is more than 3 or less than 1, reset user while (choiceLI > 3 || choiceLI < 0) { cout << " " << endl; cout << "Invalid Option, Please try again." << endl; cout << " " << endl; main(); } //options menu functions switch (choiceLI) { case 1: rentCar(numCars); case 2: viewCar(numCars); case 3: } } //while choice isn't 0 while (choiceLI != 0); cout << " " << endl; cout << "Thank you, Goodbye!" << endl; exit(0); }
/*********************************************** * Function: mainMenu * Description: Menu for user to decide what they would like to do * Return choice gets passed to main. * Input: int choice - reads user choice from menu ***********************************************/ int mainMenu() { int choice; cout << "||======================= Luxury Rent-a-Car =====================||" << endl; //list of options cout << "||===============================================================||" << endl; cout << "|| 1 - Rent a Car ||" << endl; cout << "|| 2 - Manage Car Lot ||" << endl; cout << "|| 4 - Manage Transactions ||" << endl; cout << "|| 0 - Exit ||" << endl; cout << "||===============================================================||" << endl; cout << "Please Enter Choice: "; //reads user choice from menu cin >> choice; return choice; }
void rentCar(vector
}
void viewCar(const vector
if (cInfo.size() == 0) { cout << " " << endl; cout << "No Cars in list, Returning to main Menu..." << endl; cout << " " << endl; main(); } else { cout << " " << endl; cout << "Available cars in Playlist: " << endl; for (int i = 0; i < cInfo.size(); i++) { cout << "\t" << i << ". " << cInfo.at(i).getModel() << endl; } } }
void viewCarInfo(const vector
void deleteCar(vector
Car.cpp:
#include "Car.h"
using namespace std;
Car::Car() { int vin = 0; string make = ""; string model = ""; double rate = 0; int year = 0; int numCars = 0; }
Car::Car(int vin, string make, string model, double rate, int year, int numCars) { setVin(vin); setMake(make); setModel(model); setRate(rate); setYear(year); }
int Car::getVin() const { return vin; }
void Car::setVin(int vin) { this->vin = vin; }
string Car::getMake() const { return make; }
void Car::setMake(string make) { this->make = make; }
string Car::getModel() const { return model; }
void Car::setModel(string model) { this->model = model; }
int Car::getYear() const { return year; }
void Car::setYear(int year) { if (year >= 2020 || year <= 2010) { throw out_of_range("Car Must be less than decade old"); } this->year = year; }
double Car::getRate() const { return rate; }
void Car::setRate(double rate) { this->rate = rate; }
bool Car::equals(const Car& c) { return(this->vin == c.getVin()); } string Car::toString() const { string str = ""; str += "\tVehicle Identification Number: " + vin; str += " \tName of Brand: " + make; str += " \tName of Model: " + model; str += " \tYear of Car: " + to_string(year) + " "; str += " \t Rate of Car: " + to_string(rate) + " "; return str; }
Car.h:
class Car { private: //Private data memebers int vin; string make; string model; double rate; int year; int numCars; public: //Public fuctions in class Car(); Car(int vin, string make, string model, double rate, int year, int numCars); int getVin() const; void setVin(int vin); string getMake() const; void setMake(string make); string getModel() const; void setModel(string model); int getYear() const; void setYear(int year); double getRate() const; void setRate(double rate); void decrementNumCars(); bool equals(const Car& c); string toString() const; }; #endif
Please help, Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
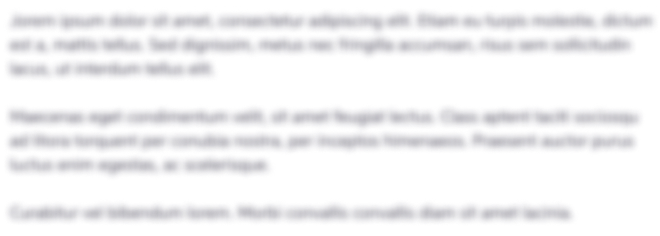
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started