Question
Design, implement, and test a reusable Money class. Are money objects values or references? You should be able to represent at least five different currencies:
Design, implement, and test a reusable Money class.
Are money objects values or references?
You should be able to represent at least five different currencies:
USD, EUR, INR (Indian Rupees), MXP (Mexican Pesos), JPY (Japanese Yen)
Include operations for adding money amounts.
Implement Javas Comparable interface. The compareTo method is called by List.sort.
Implement Javas Serializable interface. This allows Money objects to be saved to and read from files.
We should be able to add and compare money objects even if they are different currencies. To do this, create a currency converter interface with a mock implementation.
CurrencyConverter.java
How will you add and compare money of different currencies? For example:
3.14 USD + 2.78 EUR = ?
3.14 USD < 2.78 EUR = ?
How do we define classes of comparable objects in Java?
Provide an interface to a currency conversion service. How could this interface be implemented?
To be useful, Money objects should also be serializable so they can be saved to and read from files.
Turn in:
- A class diagram showing all classes and interfaces to be implemented (together with fields and operations).
- Implementation of all classes and interfaces from the diagram.
Here is a test harness for your implementation:
TestMoney.java
Heres the output I get:
deposits = [3.25 USD, 5.0 EUR, 129.0 MXP, 200.0 INR, 100.0 JPY]
deposits = [100.0 JPY, 200.0 INR, 3.25 USD, 5.0 EUR, 129.0 MXP]
m2 + m3 = 11.45 EUR
m2 + m4 = 7.592592592592593 EUR
m2 + m5 = 5.842592592592593 EUR
m2 + m1 = 8.00925925925926 EUR
IMPLEMENTATION EXAMPLE:
package money; import java.util.*; public class TestMoney { public static void main(String[] args) { Money m1 = new Money(3.25, Currency.USD); Money m2 = new Money(5.0, Currency.EUR); Money m3 = new Money(129.0, Currency.MXP); Money m4 = new Money(200.0, Currency.INR); Money m5 = new Money(100.0, Currency.JPY); Listdeposits = new ArrayList (); deposits.add(m1); deposits.add(m2); deposits.add(m3); deposits.add(m4); deposits.add(m5); System.out.println("deposits = " + deposits); deposits.sort(null); System.out.println("deposits = " + deposits); System.out.println("m2 + m3 = " + m2.add(m3)); System.out.println("m2 + m4 = " + m2.add(m4)); System.out.println("m2 + m5 = " + m2.add(m5)); System.out.println("m2 + m1 = " + m2.add(m1)); } }
package money; //Currency Converter public interface CurrencyConverter { public static CurrencyConverter calculator = new MockConverter(); // mock converter uses 2/18 rates public double exchangeRate(Currency from, Currency to); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
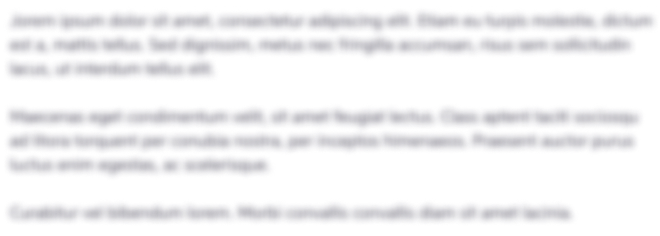
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started