Question
DESIGN THIS FIRST --- Design Hierarchy chart, pseudocode for main, module specs for EACH function FOR COMMENT: Write both C++ and pseudocode separately! Write a
DESIGN THIS FIRST --- Design Hierarchy chart, pseudocode for main, module specs for EACH function
FOR COMMENT: Write both C++ and pseudocode separately!
Write a program in C++ to allow the user to play a one-user simplified Keno game. The program will contain the following:
- A one-dim. array of ints of size 20 for the official Keno game numbers (like a lottery game)
- A one-dim. array of ints of size 15 for the user's numbers
- (OPTIONAL: A one-dim. array of ints of size 15 for the matched numbers) You must allow the user to play more than one game using a loop. It's up to you how to ask the user to play again and how to repeat if you try this option. In main:
Open the input file and output file in main to allow reading more than one game from the input file (even if you don't use the option to play more than one game). DO NOT CHANGE ANYTHING GIVEN BELOW
CALL the openInputFile function:
If the file doesn't open, display an error message and return -1 from main:
If the file doesn't open, display an error message and return -2 from main:
Then in main, call each of the following (EACH of the following paragraphs MUST be a SEPARATE FUNCTION, except the selectionSort function is the same one CALLED 2 TIMES)
o **Call a function (that you write) to read from the input into the Keno game array (pass as an argument) (see details in A. below)
o If the function (A) returns true, continue into the loop...
o Call a function (that you write) to read into the user's guess array and find how many the user guessed (see details in B. below)
o Sort the Keno game array by calling the SELECTION SORT function (given below).
void selectionSort(int array[], int size)
{
int startScan, minIndex, minValue;
for (startScan = 0; startScan
{
minIndex = startScan;
minValue = array[startScan];
for(int index = startScan + 1; index
{
if (array[index]
{
minValue = array[index];
minIndex = index;
}
}
array[minIndex] = array[startScan];
array[startScan] = minValue;
}
}
o Sort the user's array by calling the SELECTION SORT function
o Call a function (that you write, see C. below) that finds how many numbers the user's guess array matched the Keno game array, and assign its return value to a main int variable (I'll call numMatched)
o Call a function (that you write, see D. below) that writes both arrays and how many matched (must use the format shown in the test runs!)
o Ask the user if he/she wants to try another, and repeat to ** if the user wants to repeat (up to you to determine how)
A. Read from the input file 20 integers into the Keno game array (parameter). However, when reading in the loop, check if the index/counter is inside the array bounds AND YOU MUST STOP READING IF THERE ARE NO MORE NUMBERS IN THE FILE. If the index or counter is 0, return false, otherwise return true.
B. Ask the user and read how many numbers the user wants to guess (minimum 1 and maximum 15), but YOU MUST CALL THE getInteger FUNCTION GIVEN IN BELOW (pass "Enter how many numbers to choose: ", MIN_GUESSES, MAX_GUESSES)! Then read that many numbers from the user into the user's onedim. array of ints ALSO USING THE getInteger FUNCTION GIVEN BELOW for reading one number at a time passing "Enter a number: ", 1 and 80 to the getInteger function, then checking for duplicate numbers (CALL the LINEAR SEARCH function given in the textbook which MUST BE CALLED HERE, not imbedded in the function) before assigning to an array element! Assign how many the user chose to a reference parameter for it OR return it in a return statement. (That means that how many the user chose MUST be a variable in main!)
int getInteger(string prompt,
int minInt,
int maxInt)
{
int inputInt;
cout
cin >> inputInt;
while( inputInt maxInt )
{
cout = "
cout
cin >> inputInt;
}
return inputInt;
} // end getInteger
C. For each element in the user's number array, search the Keno game array (call the LINEAR SEARCH function given in BELOW which MUST BE CALLED HERE, not imbedded in the function, and NOT using your own search). If it's found, add 1 to the matched counter (optional: put the number into the matched array parameter). The user's numbers for loop MUST use the variable read in the first function for how many numbers the user guessed (so it must be a parameter). RETURN IN A RETURN STATEMENT, how many numbers in the user's array are in the Keno game array.
int searchList(int list[], int numElems, int value)
{
int index = 0; // Used as a subscript to search array
int position = -1; // To record position of search value
bool found = false; // Flag to indicate if the value was found
while (index
{
if (list[index] == value) // If the value is found
{
found = true; // Set the flag
position = index; // Record the value's subscript
}
index++; // Go to the next element
}
return position; // Return the position, or -1
}
D. Display to the screen AND write to the output file (reference parameter) the Keno game numbers, the user's numbers, and the number of matches with a label (see test output file for format). The user's numbers for loop MUST use the variable read in the first function for how many numbers the user guessed. Optional: write the numbers that matched in the matched array.
EACH OF THE ABOVE PARAGRAPHS (A. THROUGH F.) MUST BE A FUNCTION! When calling function A., you MUST assign the return value (or reference parameter) for the how many the user chose to a main variable. In functions D., E., and F., the main variable for how many the user chose MUST be passed as an argument to those functions!
Each of the numbers in bold above MUST be an external const! DO NOT USE ANY EXTERNAL (global) VARIABLES (variables declared outside of main or outside of any function)!! (external const declarations are OK.)
Include in your program:
-Arrays (one-dim. arrays as specified above) (NEVER ALLOW THE PROG. TO OUTSIDE THE ARRAY or POINTS WILL BE DEDUCTED)
- for loops for traversing the array(s), WHICH MUST ITERATE ONLY FOR HOW MANY ELEMENTS ARE USED IN THE ARRAY (so how many used, NOT necessarily the physical size, MUST be a parameter in the functions that traverse the array)
- function that returns a value (indicated above) AND functions (with array parameters as spec. above)
- Error checking specified above - input text file (ALWAYS CHECK IF IT OPENS OK BEFORE READING FROM IT) - output text file (ALWAYS CHECK IF IT OPENS OK BEFORE WRITING TO IT)
- Comments for program header, and variables, as usual
- Comment each function in the source file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
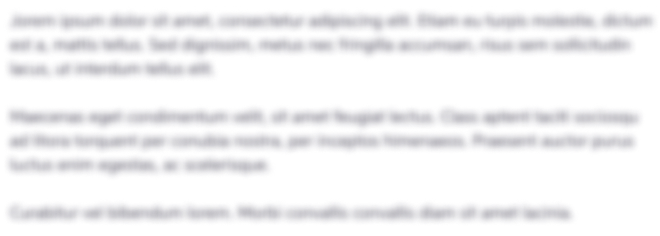
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started