Question
Develop a C++ program that implements a binary tree #pragma once // include this library to use NULL, otherwise use nullptr instead #include #include #include
Develop a C++ program that implements a binary tree
#pragma once
// include this library to use NULL, otherwise use nullptr instead
#include
#include
#include "node.hpp"
template
class BST{
public:
// Constructor for the BST class, creates an empty tree
BST(void);
// Destructor for the BST class, destroys the tree
~BST(void);
// Inserts data into the tree
// param: The data to be inserted into the tree
void insert(T);
// Removes data from the tree
// param: The data to be removed from the tree
void remove(T);
// Performs an inorder traversal
// returns: pointer to a vector containing the tree traversal
std::vector
// Performs an postorder traversal
// returns: pointer to a vector containing the tree traversal
std::vector
// Performs an preorder traversal
// returns: pointer to a vector containing the tree traversal
std::vector
// Searches the tree for a given value
// param: the data to search for
// returns: a pointer to the node containing the data or NULL if the data
// was not found
Node
// Gets the current number of nodes in the tree
// returns: the number of nodes in the tree
int get_size(void);
private:
// the root node of the tree
Node
// the number of nodes in the tree
int node_count;
};
template
BST
{
root = NULL;
node_count = 0;
}
template
BST
{
root = NULL;
while(root != NULL)
{
remove(root->get_data());
}
}
template
std::vector
{
std::vector
return vec;
}
template
std::vector
{
std::vector
return vec;
}
template
std::vector
{
std::vector
return vec;
}
template
void BST
{
}
template
Node
{
}
template
void BST
{
}
template
int BST
{
}
node.hpp
#pragma once
#include
template
class Node{
private:
T data;
Node
Node
public:
Node(void);
Node(T &data);
void set_data(T &new_data);
void set_left(Node
void set_right(Node
T get_data(void);
Node
Node
};
template
Node
{
data = 0;
left = NULL;
right = NULL;
}
template
Node
{
data = new_data;
left = NULL;
right = NULL;
}
template
void Node
{
data = new_data;
}
template
T Node
{
return data;
}
template
void Node
{
left = left_node_ptr;
}
template
void Node
{
right = right_node_ptr;
}
template
Node
{
return left;
}
template
Node
{
return right;
}
main.cpp
#include
#include
#include
#include
#include
#include "bst.hpp" // The header file for our custom linked list class
using namespace std;
int main(int argc, char** argv)
{
BST
Node
ifstream input;
int cmd, argument, ret, i;
vector
if(argc < 2)
{
cout << "useage: ./a1.out
return 0;
}
input.open(argv[1]);
// while there is something to read from the file, read
while (input >> cmd)
{
// switch on the command we read from the file
switch (cmd)
{
// if the cmd requires a parameter, read it from the file and call the
// associated function
case 1:
input >> argument;
bst.insert(argument);
cout << "Inserted " << argument << endl;
break;
case 2:
input >> argument;
bst.remove(argument);
cout << "Removed " << argument << endl;
break;
case 3:
traversal_data = bst.inorder();
if(traversal_data != NULL)
{
cout << "inorder traveral: ";
for (std::vector
i != traversal_data->end();
++i)
{
std::cout << *i << ' ';
}
cout << endl;
}
else cout << "tree appears to be empty ";
break;
case 4:
traversal_data = bst.postorder();
if(traversal_data != NULL)
{
cout << "postorder traveral: ";
for (std::vector
i != traversal_data->end();
++i)
{
std::cout << *i << ' ';
}
cout << endl;
}
else cout << "tree appears to be empty ";
break;
case 5:
traversal_data = bst.preorder();
if(traversal_data != NULL)
{
cout << "preorder traveral: ";
for (std::vector
i != traversal_data->end();
++i)
{
std::cout << *i << ' ';
}
cout << endl;
}
else cout << "tree appears to be empty ";
break;
case 6:
input >> argument;
searchResult = bst.search(argument);
if(searchResult == NULL)
{
cout << "Did not find " << argument << endl;
}
else
{
cout << "Found " << searchResult->get_data() << endl;
}
break;
case 7:
i = bst.get_size();
cout << "The tree has " << i << "nodes ";
break;
}
}
input.close();
return 0;
} cmd.txt
1 75
1 40
1 89
1 128
1 12
1 9
1 1
1 2
1 7
1 3
1 99
1 35
1 36
1 4
1 27
1 66
1 55
3
4
5
2 3
3
4
5
2 5
3
4
5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
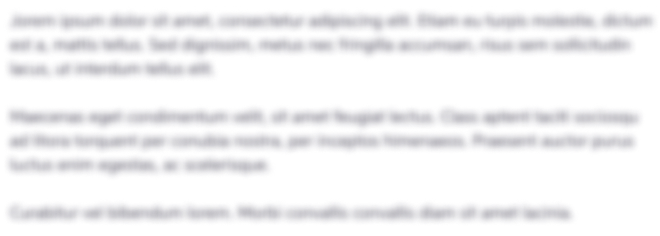
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started