Question
Develop a C program to read and process an input text file, and retrieve the following information and save to file. number of lines number
Develop a C program to read and process an input text file, and retrieve the following information and save to file.
number of lines
number of totoal words
number of distinct words
distinct words and their frequencies
Specifically, write C programs myword.h , containing the following structure definitions and function headers, and myword.c , containing the implementations of the functions. It is required to use your str_trim() and lower_case() functions from Q1, and use some other string library functions such as strtok(), strcmp() and strcpy() in the implementation.
structure definitions:
1. typedef struct word { char word[MAX_WORD]; int frequency; } WORD; typedef struct words { int line_count; int total_word_count; int distinct_word_count; } WORDSTATS;
2. int process_word(char *filename, WORD *words, WORDSTATS *wordstats)opens and reads text file of name passed by filename line by line. For each line, it gets each word, check if it is already in WORD array words, if yes, increases its frequency by 1, otherwise inserts it to the end of the WORD array words and set its frequency 1, and update total_word_count and distinct_word_count. It returns 0 if operation is successful, 1 otherwise.
3. int save_file(char *filename, WORD *words, WORDSTATS *wordstats) saves the word processed data pass words and wordstats to file of filename.
Use the provided main program myword_main.c to test your program with this input text file textdata.txt. The output file and format should be like word_report.txt, the screen output is like the following.
What's Given:
myword_main.c:
/* -------------------------------------------------- Project: cp264-a3q2 File: myword_main.c, a public test driver Author: HBF Version: 2023-01-26 -------------------------------------------------- */ #include
void display_wordstats_words(WORDSTATS *wordstats, WORD *words); void read_display_file(char *filename);
int main(int argc, char *args[]) { char infilename[40] = "textdata.txt"; //default input file name char outfilename[40] = "word_report.txt"; //default output file name
if (argc > 1) { if (argc >= 2) strcpy(infilename, args[1]); if (argc >= 3) strcpy(outfilename, args[2]); }
WORD words[MAX_WORDS] = {0}; WORDSTATS wordstats = {0}; process_word(infilename, words, &wordstats); display_wordstats_words(&wordstats, words);
save_file(outfilename, words, &wordstats);
read_display_file(outfilename);
return 0; }
void display_wordstats_words(WORDSTATS *wordstats, WORD *words) { printf(" %s:%s ","word stats", "value"); printf("%s:%d ", "line count", wordstats->line_count); printf("%s:%d ", "total word count", wordstats->total_word_count); printf("%s:%d ", "distinct word count", wordstats->distinct_word_count);
printf(" %s:%s ","distinct words", "frequency"); int i; for (i = 0; i < wordstats->distinct_word_count; i++) { printf("%s:%d ", words[i].word, words[i].frequency); } }
void read_display_file(char *filename) { FILE *fp = fopen(filename, "r"); if (fp == NULL) printf(" no file "); else printf(" file contents "); char line[1000]; while (fgets(line, 1000, fp) != NULL) { printf("%s", line); } fclose(fp); }
textdata.txt:
THIS IS THE FIRST TEST. This is the second test. CP264 Data Structures
word_report.txt
word stats:value line count:3 total word count:13 distinct word count:9 distinct words:frequency this:2 is:2 the:2 first:1 test:2 second:1 cp264:1 data:1 structures:1
Public Test:
word stats:value line count:3 total word count:13 distinct word count:9 distinct words:frequency this:2 is:2 the:2 first:1 test:2 second:1 cp264:1 data:1 structures:1 file contents word stats:value line count:3 total word count:13 distinct word count:9 distinct words:frequency this:2 is:2 the:2 first:1 test:2 second:1 cp264:1 data:1 structures:1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
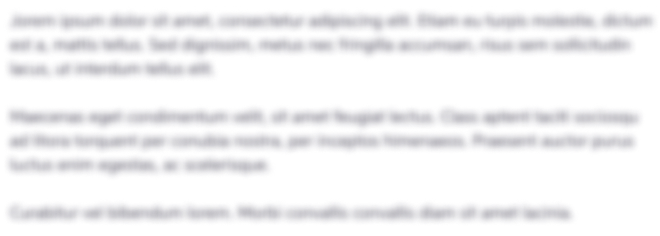
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started