Question
Develop a dynamic programming solution to the Crazy-8 game in java. Input: a sequence of cards c[0], c[1] c[n-1] Example: 7S 7H KD KC 8H
Develop a dynamic programming solution to the Crazy-8 game in java.
Input: a sequence of cards c[0], c[1] c[n-1]
Example: 7S 7H KD KC 8H (7 of Spades, 7 of Hearts, King of Diamonds, King of Clubs, 8 of Hearts)
Output: the longest trick subsequence c[i1] c[i2] c[ik], where i1 < i2 . . .< ik
Definition of a Trick Subsequence: For it to be a trick subsequence, it must be that "j, c[ij] and c[ij+1] match that is
they either have the same rank
or they are from the same suit
or one of them is an 8 For the above example (card sequence: 7S 7H KD KC 8H),
the longest trick subsequence is: KD KC 8H i = 1 i = 2 i = 3 i = 4 i = 5 C[i] 7S 7H KD KC 8H
Max Score[i] 1 2 1 2 3 Sub Pointer 0 1 0 3 4 For the above example (card sequence: 7C 7H KC KS 8D), the longest trick subsequence is: 7C KC KS 8D i = 1 i = 2 i = 3 i = 4 i = 5 C[i] 7C 7H KC KS 8D
Max Score[i] 1 2 2 3 4 Sub Pointer 0 1 1 3 4 The following main program will be used to create a random card sequence, and your program will print the trick sequence. The card sequence is a sequence of two characters a single character rank followed by a single character for suit. The rank of 10 is omitted. Main Program: package cse361;
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] ranks = {"1", "2", "3", "4", "5", "6", "7", "8", "9", "J", "Q", "K", "A"};
String[] suits = {"C", "S", "H", "D"};
int seqLength = Integer.parseInt(args[0]);
String[] cardSeq = new String[seqLength];
int cntCards = ranks.length;
int cntSuite = suits.length;
for (int i = 0; i < seqLength; i++) {
// generate the first random number
int rand1 = (int)(Math.random()*cntCards);
int rand2 = (int)(Math.random()*cntSuite);
cardSeq[i] = ranks[rand1] + suits[rand2]; }
// Print card cardSeq
for (int i = 0; i < cardSeq.length; i++)
System.out.println (cardSeq[i]);
// Create Card Sequence
Crazy8 crazy8Seq = new Crazy8 (cardSeq);
crazy8Seq.findTrickSequence();
crazy8Seq.printTrickSeqeunce(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
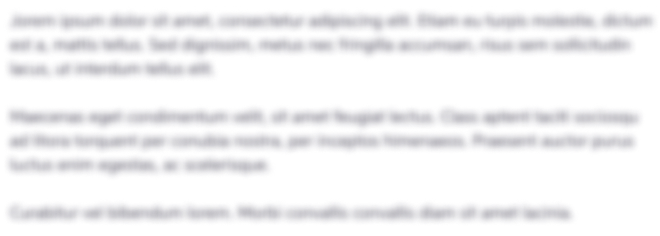
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started