Question
develop a function called bubbleSort that accepts a list as a parameter and sorts that list using the bubble sort algorithm . A bubble sort
develop a function called bubbleSort that accepts a list as a parameter and sorts that list using the bubble sort algorithm. A bubble sort is the simplest, and least efficient, sorting algorithm that works by repeatedly swapping the adjacent elements if they are in wrong order. In effect, the algorithm bubbles larger values to the end of the collection.
Because the algorithm requires referencing and comparing individual elements, an index is used to reference the elements of the list. The algorithm also requires a nested loop.
Do the following in the function:
- Using i as the loop variable, iterate over the range 0 through the length of the list:
- Using j as the loop variable, iterate over the range 0 through (length of the list - i - 1):
- if the list element referenced by j is greater than the list element reference by j + 1:
- Swap the elements
Note, your code should work with the list parameter passed to the function, i.e., do not attempt to create a new list. As evidenced by the above algorithm, the position of the list elements are changing as the algorithm executes. The expression length of list - i - 1 reduces the upper range since with each successive pass the larger values are being moved to the end of the list. You may want to use print statements to print i and j to observe how the algorithm works.
Given:
animals = ['elephant', 'cat', 'moose', 'antelope', 'elk', 'rabbit', 'zebra', 'yak', 'salamander', 'deer',\ 'otter', 'minx', 'giraffe', 'goat', 'cow', 'tiger', 'bear'] bubbleSort(animals) print(animals)
expected output:
['antelope', 'bear', 'cat', 'cow', 'deer', 'elephant', 'elk', 'giraffe', 'goat', 'minx', 'moose', 'otter', 'rabbit', 'salamander', 'tiger', 'yak', 'zebra']
Step by Step Solution
There are 3 Steps involved in it
Step: 1
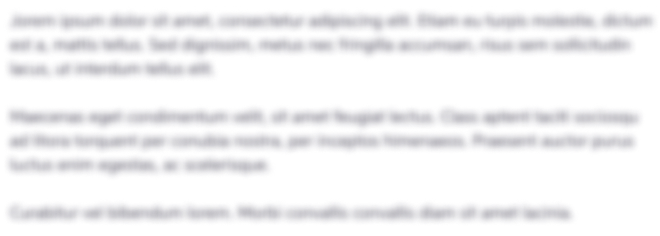
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started