Question
Develop a programMonthDetails.javathat will collect a numeric month and year from a user and then display how many days there are in that month. The
Develop a programMonthDetails.javathat will collect a numeric month and year from a user and then display how many days there are in that month. The month that is displayed to the user should be the name of the month, not the number (ie March instead of 3).
Keep in mind that the number of days in February changes based on whether or not it is a leap year. If you would like to keep it simple, you can consider any year divisible by 4 to be considered a leap year and use that for your program. However, calculating a leap year is not always that simple. The following formula can be used to accurately calculate a leap year:
if the year modulo 400 == 0, it is a leap year
or
if the year modulo 100 != 0 AND year modulo 4 == 0, it is a leap year
Fortunately we have some operators at our disposal that can make this calculation work. We can use an if statement containing both of those conditions to determine a leap year. The if statement looks like this:
if ( (year % 100 != 0 && year % 4 == 0) || (year % 400 == 0) ) {
(do something when it is a leap year)
}
else {
(do something when it is NOT a leap year)
}
Use parallel arrays (one String and one int) to keep track of both the number of days in each month as well as the names of each month. If a leap year is encountered, you shouldchange the value for February that is stored in the array containing the number of days in each month. Then use that value to display the output to the user.
Before starting to write the actual code in Java, develop a pseudocode document planning out the steps the program will take. Also be sure to add your name, date, course number, and project title to the top of the java file.
When the program ends, display a message telling the user how awesome he or she is, and then end the program. Test the program to verify that it operates properly with both leap years and non-leap years. In the screenshot(s) you submit for the assignment, test the three values given to verify that the program functions per the requirements given.
You will find the three test cases below. It is OK torun the programthreeseparate timesto accomplish this. Or you can try to put the program in a loop to run at least three times, but that is optional:
Test case 1 | Find how many days are in each month! Please enter the numeric month:2 Please enter the year:1997 There are 28 days in the month of February, 1997. Thank you for being amazing! Have a great day! |
Test case 2 | Find how many days are in each month! Please enter the numeric month:5 Please enter the year:2005 There are 31 days in the month of May, 2005. Thank you for being amazing! Have a great day! |
Test case 3 | Find how many days are in each month! Please enter the numeric month:2 Please enter the year:2016 There are 29 days in the month of February, 2016. Thank you for being amazing! Have a great day! |
Tips for completing this project:
- No loop is required in this program. You can run it three separate times.
- Recall what an index number does and how you use it to access items stored in an array.
- If done correctly, you only need one if/else statement to calculate a leap year.
- Don't try to solve the leap year issue until the program is working successfully with a valid month and year entered.
- If you're having trouble starting, begin by collecting the numeric month from the user and try to display the String equivalent.
Sol100:
// Program: MonthDetails.java
// Author: [Your Name]
// Date: [Date]
// Course: [Course Number]
// Project Title: Month Details
// Declare arrays for month names and days in each month
String[] monthNames = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};
int[] daysInMonth = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// Prompt user for month and year
Display "Find how many days are in each month!"
Display "Please enter the numeric month: "
Input month
Display "Please enter the year: "
Input year
// Check if it is a leap year and update February if necessary
if ((year % 100 != 0 && year % 4 == 0) || (year % 400 == 0)) {
daysInMonth[1] = 29;
} else {
daysInMonth[1] = 28;
}
// Display number of days in the entered month and year
Display "There are " + daysInMonth[month - 1] + " days in the month of " + monthNames[month - 1] + ", " + year + "."
Display "Thank you for being amazing! Have a great day!"
Note: This is just a sample pseudocode and you may need to modify it to suit your needs and programming style.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
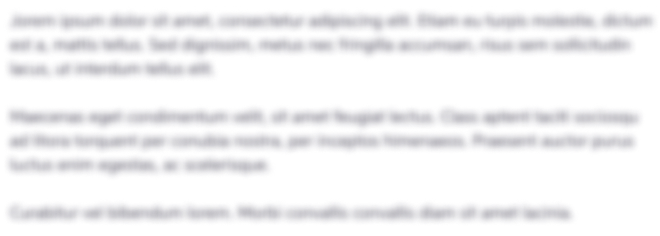
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started