develop a system that can track employee information for two Organizations (such as Google and Microsoft). The Employee information you must track is as follows:
develop a system that can track employee information for two Organizations (such as Google and Microsoft). The Employee information you must track is as follows:
Name
Gender
Job Title
Organization they work for
Birthday
As for the Organization that the Employee works for, you must also track this information:
Organization Name
Number of Employees
The system must be able to properly compare any two employees against each other to determine if they are the same Employee. This means that if you compared two Employees with the same Name, Gender, Birthday and Organization, the system should think that they are equal to one another. If any of these properties are different, then the two Employees are not the same Employee.
The same rules apply to comparing Organizations to one another. Organizations with the same Organization name are to be thought of as equal, different names means different organizations.
Use the charts on the next page to create your classes.
Employee |
private String name; private String gender; private String jobTitle; private Organization organization; private String birthDate; |
Employee (String theName, String theGender, String title, Organization org, String theBirthDate) |
<<accessors>> String getName () String getGender () String getJobTitle () Organization getOrganization () String getBirthDate () String toString () boolean equals (Employee other) <<mutators>> void changeJobTitle (String newTitle) void changeName (String newName) void changeOrganization (Organization org) |
Organization |
private String organizationName; private int numEmployees; |
Organization (String name, int employees) |
<<accessors>> String getOrganizationName () int getNumEmployees () String toString () boolean equals (Organization other) <<mutators>> void changeOrganizationName (String newName) |
*****NOTE: All constructors and methods should be public. *****
* Note : There is a zip file listed that has a starts for each of the classes that are required. They are located in the source file and you will also find a test file for the program.
STARTER CODE - EMPLOYEE
public class Employee
{
}
STARTER CODE - ORGANIZATION
/**
* Organization.java
*
* @author - Jane Doe
* @author - Period n
* @author - Id nnnnnnn
*
* @author - I received help from ...
*
*/
public class Organization
{
}
TESTER CODE
/**
* This class tests the Employee and Organization classes.
*/
public class Tester
{
public static void main(String[] args)
{
Employee emp1 = new Employee("Sally Brown", "Female", "Vice President",
new Organization("Google", 5500), "11-11-1969");
System.out.println(emp1);
System.out.println();
System.out.println("Name should be Sally Brown and is " + emp1.getName());
System.out.println("Gender should be Female and is " + emp1.getGender());
System.out.println("Job title should be Vice President and is " + emp1.getJobTitle());
System.out.println("Birth date should be 11-11-1969 and is " + emp1.getBirthDate());
System.out.println("Organization should be Google and is "
+ emp1.getOrganization().getOrganizationName());
System.out.println("Number of employees in organization should be 5500 and is "
+ emp1.getOrganization().getNumEmployees());
System.out.println();
Employee emp2 = new Employee("Sally Brown", "Female", "Vice President",
new Organization("Google", 5500), "11-11-1969");
System.out.println(emp2);
System.out.println();
System.out.println("Name should be Sally Brown and is " + emp2.getName());
System.out.println("Gender should be Female and is " + emp2.getGender());
System.out.println("Job title should be Vice President and is " + emp2.getJobTitle());
System.out.println("Birth date should be 11-11-1969 and is " + emp2.getBirthDate());
System.out.println("Organization should be Google and is "
+ emp2.getOrganization().getOrganizationName());
System.out.println("Number of employees in organization should be 5500 and is "
+ emp2.getOrganization().getNumEmployees());
System.out.println();
Employee emp3 = new Employee("Charlie Brown", "Male", "President",
new Organization("Microsoft", 15500), "9-15-1965");
System.out.println(emp3);
System.out.println();
System.out.println("Name should be Charlie Brown and is " + emp3.getName());
System.out.println("Gender should be Male and is " + emp3.getGender());
System.out.println("Job title should be President and is " + emp3.getJobTitle());
System.out.println("Birth date should be 9-14-1965 and is " + emp3.getBirthDate());
System.out.println("Organization should be Microsoft and is "
+ emp3.getOrganization().getOrganizationName());
System.out.println("Number of employees in organization should be 15500 and is "
+ emp3.getOrganization().getNumEmployees());
System.out.println();
Employee emp4 = new Employee("Charlie Brown", "Male", "President",
new Organization("Google", 5500), "9-15-1965");
System.out.println(emp4);
System.out.println();
System.out.println("Name should be Charlie Brown and is " + emp4.getName());
System.out.println("Gender should be Male and is " + emp4.getGender());
System.out.println("Job title should be President and is " + emp4.getJobTitle());
System.out.println("Birth date should be 9-14-1965 and is " + emp4.getBirthDate());
System.out.println("Organization should be Google and is "
+ emp4.getOrganization().getOrganizationName());
System.out.println("Number of employees in organization should be 5500 and is "
+ emp4.getOrganization().getNumEmployees());
System.out.println();
System.out.println("emp1.equals(emp2) should be true and is " + emp1.equals(emp2));
System.out.println("emp1.equals(emp3) should be false and is " + emp1.equals(emp3));
System.out.println("emp1.equals(emp4) should be false and is " + emp1.equals(emp4));
System.out.println("emp2.equals(emp3) should be false and is " + emp2.equals(emp3));
System.out.println("emp2.equals(emp4) should be false and is " + emp2.equals(emp4));
System.out.println("emp3.equals(emp4) should be false and is " + emp3.equals(emp4));
}
}
Step by Step Solution
3.47 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
Coding public class Employee private String name private String gender private String jobTitle private Organization organization private String birthDate param name param gender param jobTitle param o...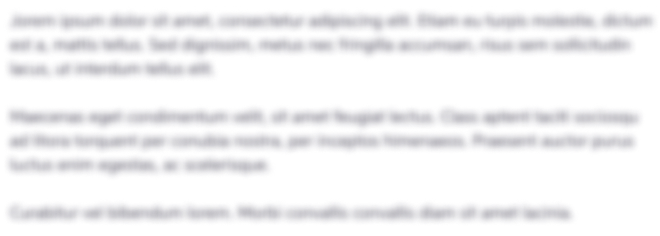
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started