Question
Develop a toString method for the Deck class. It should return a single string that represents the cards in the deck. When it's printed, this
Develop a toString method for the Deck class. It should return a single string that represents the cards in the deck. When it's printed, this string should display the same results as the print method in Section 13.1.
Hint: You can use the + operator to concatenate strings, but it is not very efcient. Consider using java.lang.StringBuilder instead; see Section 10.11.
Code:
import java.util.Arrays;
import java.util.Random;
/**
* A deck of playing cards (of fixed length).
*/
public class Deck {
private Card[] cards;
/**
* Constructs a standard deck of 52 cards.
*/
public Deck() {
this.cards = new Card[52];
int index = 0;
for (int suit = 0; suit <= 3; suit++) {
for (int rank = 1; rank <= 13; rank++) {
this.cards[index] = new Card(rank, suit);
index++;
}
}
}
/**
* Constructs a deck of n cards (all null).
*/
public Deck(int n) {
this.cards = new Card[n];
}
/**
* Gets the internal cards array.
*/
public Card[] getCards() {
return this.cards;
}
/**
* Displays each of the cards in the deck.
*/
public void print() {
for (Card card : this.cards) {
System.out.println(card);
}
}
/**
* Returns a string representation of the deck.
*/
public String toString() {
return Arrays.toString(this.cards);
}
/**
* Randomly permutes the array of cards.
*/
public void shuffle() {
}
/**
* Chooses a random number between low and high, including both.
*/
private static int randomInt(int low, int high) {
return 0;
}
/**
* Swaps the cards at indexes i and j.
*/
private void swapCards(int i, int j) {
}
/**
* Sorts the cards (in place) using selection sort.
*/
public void selectionSort() {
}
/**
* Finds the index of the lowest card
* between low and high inclusive.
*/
private int indexLowest(int low, int high) {
return 0;
}
/**
* Returns a subset of the cards in the deck.
*/
public Deck subdeck(int low, int high) {
Deck sub = new Deck(high - low + 1);
for (int i = 0; i < sub.cards.length; i++) {
sub.cards[i] = this.cards[low + i];
}
return sub;
}
/**
* Combines two previously sorted subdecks.
*/
private static Deck merge(Deck d1, Deck d2) {
return null;
}
/**
* Returns a sorted copy of the deck using selection sort.
*/
public Deck almostMergeSort() {
return this;
}
/**
* Returns a sorted copy of the deck using merge sort.
*/
public Deck mergeSort() {
return this;
}
/**
* Reorders the cards (in place) using insertion sort.
*/
public void insertionSort() {
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
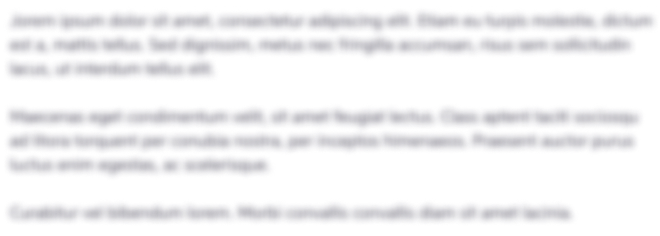
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started