Question
Develop an algorithm using the Java programming language that implements a basic stack data structure. Your stack must have the ability to push entries on
Develop an algorithm using the Java programming language that implements a basic stack data structure.
Your stack must have the ability to push entries on the stack. Your stack must also have the ability to pop entries off of the stack.
By the end of week on you must post your work to the Unit 3 Stack Development Assignment workshop link and solicit help or feedback from your peers.
For this assignment, assume that you are developing a system for a manufacturing assembly line that builds automobiles. There are three stations in the manufacturing line where an inspector will visually inspect the vehicle. You program must keep track of these inspections as they occur. You decided to develop your program using a stack data structure. As your vehicle begins the line you will push the number 0, which indicates that that an inspection has not yet occurred, onto the stack three subsequent times.
At each station in the line you will pop one of the items off of the stack. Each time your algorithm pops an item from the stack you must print it out to the console using the System.out.println function. Your algorithm should perform the functions of the stack as illustrated in the above diagram.
You will develop your algorithm using Java code, and by using the Jeliot tool as a development environment. Jeliot can be executed directly from the following website (http://cs.joensuu.fi/jeliot/javaws/jeliot.jnlp). Instructions for using Jeliot can be found at http://cs.joensuu.fi/jeliot/index.php. You can use the example files provided with the course as a reference for developing a simple algorithm and for examples on implementing a structure as either an array or link implementation.
You can select to implement your stack as either an array or linked list implementation. When you post your algorithm, you should compare the efficiency of your algorithm with those of your peers to determine what approach provides the most efficient and elegant solution to the problem. As part of your assignment you must submit both a description of the assignment and how your algorithm works including an Asymptotic analysis of your algorithm. Your analysis must include the efficiency of your algorithm expressed in Big Oh notation.
As part of your assignment, you must include the code of your algorithm. All of these elements of this assignment must be completed and posted to the assignment for Unit 3 by the end of the week for unit 3. The following week, you must review the work of at least three of your peers. As part of your review, you must copy and paste your peers code into the Jeliot tool and execute it. If the code does not function properly you should make suggestions on how to correct the problems (if possible). If the efficiency of the algorithm can be improved you should make these suggestions. You should coordinate with your fellow student and follow up on any corrections they make by re-running the code and providing additional feedback. As you review your own algorithm and those of your peers, you should be looking for the following characteristics that our text outlined as being requirements of a good algorithm.
- It must provide the correct output based upon the input
- It must be composed of concrete steps
- There can be NO ambiguity of the flow of the algorithm
- The algorithm must have a finite number of steps that is determinable
- The algorithm must terminate or complete
If either your algorithm or the algorithms of your peers that you are reviewing do not exhibit these characteristics, you should collaboratively determine why the algorithm does not exhibit these characteristics and offer suggestions to correct the algorithm such that it does exhibit these characteristics.
Addendum: Linked List Example If you need an example of the code that can be used to implement a linked list, you can look at the following and perhaps should animate it in the jeliot environment. The following implements a simple linked list and then prints the list out after it has been created. The data structure implemented in this example is a LIFO (last in first out) structure, which we know from this units learning, is known as a stack. We could have implemented the code to add items to the list as a method in this example, but did not to simplify the code.
import Prog1Tools.IOTools; class Node { Node ptr; int value; public Node(int value) { this.value = value; } } public class CreateLinkedList { public static void main(String[] args) { Node link, plink; // root will be the beginning of the linked list Node root = new Node(5); // each additional node will link to preceeding one link = new Node(1); link.ptr = root; plink = link; link = new Node(8); link.ptr = plink; plink = link; link = new Node(6); link.ptr = plink; plink = link; link = new Node(3); link.ptr = plink; plink = link; // Move through the list and print out each value printList(link); } public static void printList(Node node) { if (node != null) { System.out.println(" Value: " + node.value); printList(node.ptr); } } }
0 2 0 2 2 2 Call push routine 3 timas Call pcp routine Call pep routine Call pop rouine 0 2 0 2 2 2 Call push routine 3 timas Call pcp routine Call pep routine Call pop rouineStep by Step Solution
There are 3 Steps involved in it
Step: 1
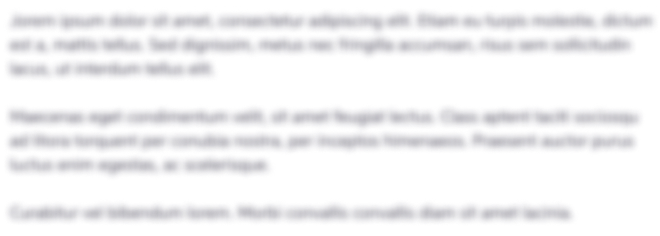
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started