Question
Develop an application to assist customers of the Zvis car rental company in processing their orders.The following is the ordering information for Zvis: Choice Of
Develop an application to assist customers of the Zvis car rental company in processing their orders.The following is the ordering information for Zvis:
Choice Of Car Car CodeDaily Cost Weekly Cost
Compact1$15$90
Mid Size2$20$120
Full Size3$30$180
SUV4$35$200
Daily and weekly rates are increased by 10% for cars equipped with the luxury package option.
A flat fee of $25 is assessed if the customer requires the car to be equipped with GPS.
If insurance is requested, the following rates apply:
Age Of DriverDaily CoverageWeekly Coverage
18 to 25$7$40
26 to 40$5$30
Over 40$3$15
There is a $10 fee to drop off the car at another location.
Add $5 for late return protection.This allows the customer to drop off the car up to 3 hours late and not be charged a full day's rate.Without this coverage, the customer is charged a full day's rate if the car is dropped off more than 1 hour late.
Veterans and active members of the armed services receive a 10% discount on the total gross cost of the order.
AARP members receive a 5% discount on the total gross cost of the order.
The program must prompt the user to enter the customer's name, age and period for which the car is required either in days or weeks(asking the customer to enter the pickup date and drop off date and then calculating the rental period will involve complex code that we are not prepared to undertake).The program must display all the available options, accept the customer's responses and then process the customer's order and display the preliminary bill.
The format of the preliminary bill is as follows:
Zvis Car Rental Company
Preliminary Bill
Customer____________________Age_______
Requested Car________________Period:Weeks ___Days ___
Gross Charges .................................. ______
Add:
Insurance ................................ ______
Luxury Package ...................... ______
GPS .......................................... ______
Drop Off Option ..................... ______
Late Return Protection ......... ______
Total Gross Charges .......______
Less:
Military Discount ................... ______
AARP Discount ....................... ______
Net Preliminary Charges ...... ______
For additional charges and discounts that are not applicable, display a zero alongside the item.Display the actual car requested not the car code.Also fully display the rental period.
Validation Requirements
1.The customer's name cannot be blank
2.The customer's age cannot be less than 18 or greater than 95.
3.The rental period cannot exceed 180 days.
4.The car code must be 1, 2, 3 or 4.
5.The insurance, luxury, GPS, drop off, late. Military and AARP responses must be either yes or no or y
or n.
Processing Specifications
1.The rental period must be displayed in weeks and days.In prompting the user to enter the rental period, the user should be able to enter the number of weeks as well as the number of days.If the user enter a rental period of 13 days, charges should be based on a period of 1 week and 7 days.
2.Gross charges = daily and/or weekly rate multiplied by number of rental days and/or number of rental weeks (luxury package charges are not included in the gross charges)
3.Total gross charges = gross charges plus insurance, luxury package, GPS, drop off and late return charges.
4.Net preliminary charges = total gross charges minus military and AARP discounts.
5.The requested car must display the car model not the car model code.
6.Your program should display options information before asking the user to respond.
7.Your program solution requires modular design.
If the user requests a rental period exceeding 7 days, the program must determine the charges for the number of weeks and number of days in the period.A request for 12 days will create charges for 1 week and 5 days.
You are encouraged to perform manual calculations to verify that your program is running correctly.
Collate the program listing along with the preliminary bills in the order as indicated below.
For submission, prepare bills for the following customers:
Customer AgePeriodCarInsuranceLuxuryGPSDrop OffLateMilitaryAARP
Michael Jones251 (w)1yesnoyesnoyesyesno
Mary Contrary655 (d)3noyesnoyesnonoyes
Brian Kirkland302 (w)4yesyesyesyesyesyesno
Jenny Bombay3410 (d)4nononoyesyesnono
David Garcia503 (w)2yesyesyesnonoyesno
Help with Major 1
# function to validate and return correct yes no responses
# prompt message is a parameter variable that will receive the message string
# supplied in the function call and will be used when prompting the user to respond yes or no
def get_yes_no_response(prompt_message):
yes_no_response_is_invalid = True
while yes_no_response_is_invalid:
yes_no_response = input(prompt_message)
print()
if yes_no_response == "yes" or yes_no_response == "no":
yes_no_response_is_invalid = False
else:
print ("Invalid response; please answer yes or no")
# once a valid response is keyed and the loop terminates, the response is returned to the
# variable on the left of the equal sign in the line of code that call the function
return yes_no_response
def car_rental():
# get input data
# show car selection table and get car requested
print()
print ("CarsCar CodeDaily CostWeekly Cost")
print ("---------------------------------------------")
print ("Compact1$15$ 90")
print ("Mid Size2$20$120")
print ("Full Size3$30$180")
print ("SUV4$35$200")
car_selection_is_invalid = True
while car_selection_is_invalid:
print()
car = input("Enter the car code of the car you want to rent: ")
if car == "1" or car == "2" or car == "3" or car == "4":
car_selection_is_invalid = False
else:
print()
print ("Invalid car selection; please re-enter your car choice")
# get number of weeks requested
print ()
print ("The rental period cannot exceed 6 months / 24 weeks / 180 days")
weeks_requested_is_invalid = True
while weeks_requested_is_invalid:
try:
print()
weeks = int(input("Enter the number of weeks requested: "))
print()
if weeks < 0 or weeks > 24:
print ("Weeks requested is invalid; re-enter weeks requested: ")
else:
weeks_requested_is_invalid = False
except:
print ("Weeks requested must be numeric; re-enter weeks requested")
# get number of days requested
print ("The number of days requested cannot exceed 6")
#
#
# continue to get other input data for the rental
#
#
#
#
# get insurance request response
print()
print ("The following are the insurance rates:")
print("Age Of DriverDaily CoverageWeekly Coverage")
print("18 to 25$7$40")
print("26 to 40$5$30")
print("Over 40$3$15")
print()
# this line calls the get_yes_no_response function and passes the insurance request message to it
# the function will return the valid response of yes or no and store it in insurance_response
insurance_response = get_yes_no_response("Are you requesting insurance coverage (yes/no)? ")
luxury_response = get_yes_no_response("Are you requesting the luxury package (yes/no) ?")
GPS_response = get_yes_no_response("Do you want the car to be equipped with GPS (yes/no)?")
# same for the other options
# begin processing operations once all the inputs have been supplied
# etc
# etc
Step by Step Solution
There are 3 Steps involved in it
Step: 1
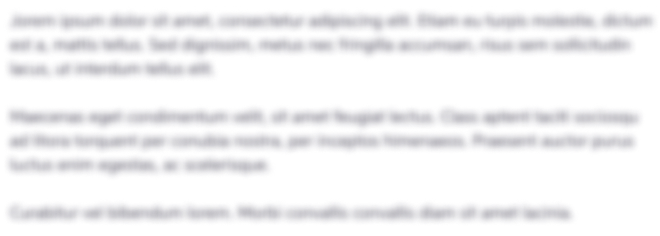
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started