Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Develop Two Functions: 1 . void deleteTree ( BSTnode * root ) Delete an entire Binary Search Tree. 2 . void deleteNode ( BSTnode *
Develop Two Functions:
void deleteTreeBSTnode root
Delete an entire Binary Search Tree.
void deleteNodeBSTnode root int value
Delete a Binary Search Tree node with the given while maintaining the BST Each node in the Binary Search Tree will have a unique value. If the value is not in the BST print: "There is no node in the Binary Search Tree with that value." This is to be added to our code definition of BSTs in class, featured below:
#include
#include
#include
using namespace std;
struct BSTnode
int data;
BSTnode left;
BSTnode right;
;
BSTnode addNodeBSTnode root int value
if root nullptr
root new BSTnode;
rootleft nullptr;
rootright nullptr;
rootdata value;
return root;
else if value rootdata
rootleft addNoderootleft, value;
return root;
else if value rootdata
rootright addNoderootright, value;
return root;
return root;
bool containsValueBSTnode root int value
if rootdata value
return true;
else if rootdata value
if rootleft nullptr
return false;
else
return containsValuerootleft,value;
else if root data value
if root right nullptr
return false;
else
return containsValuerootright, value;
return false;
BSTnode nodeSearch BSTnode root int value
if root data value
return root;
else if root data value
return nodeSearchrootleft,value;
else if root data value
return nodeSearchrootright, value;
return nullptr;
void displayBSTnode root
if root nullptr
return;
else
displayrootleft;
cout rootdata ;
displayrootright;
int main
BSTnode root nullptr;
root addNoderoot;
root addNoderoot;
root addNoderoot;
root addNoderoot;
root addNoderoot;
root addNoderoot;
root addNoderoot;
cout containsValueroot endl;
cout containsValueroot endl;
deleteNoderoot;
deleteNoderoot;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
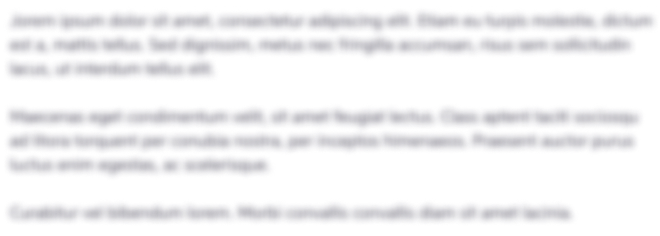
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started