Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Directions, PART II: Threaded Summation Name your program threaded _ sum.c and name the generated executable threaded _ sum. All code should be written in
Directions, PART II: Threaded Summation
Name your program threadedsum.c and name the generated executable threadedsum. All code should be written in c Write a program to calculate the sum of numbers found in a file using threading. The code and makefile for this part should be saved to a directory in your repo named threadedsum. To build a program with pthread support to enable threading, you need to provide the following flags to gcc: pthread.
You will need the following struct declaration in your code it can be stored in threadedsum.c :
typedef struct threaddatat
const int data; pointer to array of data read from file ALL
int startInd; starting index of threads slice
int endInd; ending index of threads slice
long long int totalSum; pointer to the total sum variable in main
pthreadmutext lock; critical region lock
threaddatat;
You will need to write a minimum of the following functions you may implement additional functions as you see fit:
main
Input Params: int argc, char argv
Output: int
Functionality: This funciton will parse your command line arguments threadedsum data.txt and check that arguments have been provided executable number of threads to use, filename to read data from, whether to use locking or not If arguments aren't provided, main should tell the user that there aren't enough parameters, and then return to signal that an error occured at execution.
Otherwise, main should first call readFile which will read in all data from the file that corresponds to the commandlineprovided filename.
Then it should check whether the number of Threads requested and make sure that it is less than the amount of values read, otherwise output Too many threads requested and return
Create a long long int totalSum variable and initialize it to ; it will hold the total array sum. Store the current time using gettimeofday; this is the time that the entire Threaded implementation of summation initiates at The program should now create and initialize a pthreadmutext pointer variable. If the user chooses not to use locking by setting the fourth command line argument to be set the variable to be pointer to NULL. Otherwise, the mutex pointer variable will be used by any created Threads to implement required locking, so initialize it with pthreadmutexinit.
At this point, the program should construct an array of threaddatat objects, as large as the number of Threads requested by the user. Then it should loop through the array of threaddatat and set the pointer to the data array containing the previously read values, the startIndex, and endIndex of the slice of the array you'd like a Thread to process ie to sum through and the lock to point to the previously created pthreadmutext Note: There are several ways to calculate the start and end indices, this is left up to your implementation.
The program should now make an array of pthreadt objects as large as the number of Threads requested by the user Store the time that threading begins using gettimeofday. Then run a loop that iterates over all pthreadt objects and call pthreadcreate within the loop while passing it the corresponding pthreadt object in the array, the routine to invoke arraySum and the corresponding threaddatat object in the array created and initialized in the previous step the one that contains perThread arguments such as the start and end index that the specific Thread is responsible for
Next, the program should perform pthreadjoin on all the pthreadt objects in order to ensure that the main Thread waits until all children are all finished with their work before proceeding to the next step.
Finally, store the time that the program reached this point in another gettimeofday. calculate the total time of execution. Dont forget to convert to ms Print out the final sum, and the total execution time and exit from the main Thread to terminate. The output should look something like this:
Threaded execution outputs
readFile
Input Params: char int
Output: int
Functionality: readFile in part II is identical to readFile in part I. You may reuse the same code.
arraySum
Input Params: void
Output: void
Functionality: This function is executed by each threaddatat object, which is what is passed as a parameter. Since the input type is void to adhere by the pthread API, you have to typecast the input pointer into the appropriate pointer type to reinterpret the data.
Sum the threaddatatdata array from threaddatatstartIndex to threaddatatendIndex the calling thread's current slice of the original array into a locally defined long long int threadSum variable. If the command line arguments indicated that the user doesnt want to use a lock, the threaddatatlock variable will be NULL since you set it in main This indicates you should NOT use the lock and
Step by Step Solution
There are 3 Steps involved in it
Step: 1
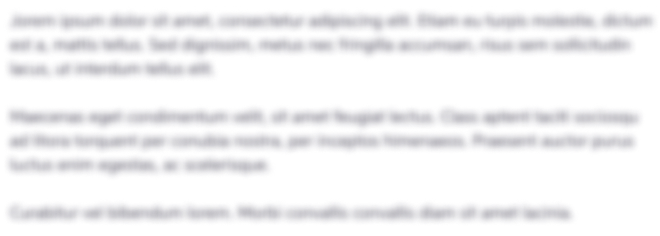
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started