Question
Implement the 12 classes, 5 enums, and one interface shown in the diagram above and then answer the questions at the end of the document.
Implement the 12 classes, 5 enums, and one interface shown in the diagram above and then answer the questions at the end of the document.
Class Design:
Zoo – A zoo contains a collection of animals.
Animal – An animal is a living creature. Each animal has a name, species, & weight. An animal may be able to fly, swim, or walk. An animal can breathe either through lungs or through gills. A mother animal can deliver babies either though laying eggs or through live birth. Animals are either cold or warm blooded. Their skin may be covered with fur. Animal in this project is an interface.
AbstractAnimal – An abstract class to implement the Animal interface. An abstract class cannot be instantiated. Many of the methods are defined as abstract, leaving it for one of the eight subclasses to define.
Bird, Amphibian, Mammal, Reptile, Fish, FlightlessBird, SwimmingMammal, DuckBilledPlatypus – subclasses of AbstractAnimal, any of which can be instantiated into an object. Defines the methods left as abstract in AbstractAnimal. All of these subclasses have a similar argument signature.
AnimalFactory – a class with a single static method, getInstance(), which, depending on the species of the animal, will create the appropriate subclass of AbstractAnimal. You are not meant to create Animals using the constructors for the subclasses of Abstract.
Depending on the species of the animal. There are two ways to do this:
1. Use a giant if block to examine the animal’s species and return an object of the correct subtype of AbstractAnimal, or
2. Implement a hash map where the key is the animal species, and the value is an object of the appropriate subtype of AbstractAnimal. Use the map get() method to return the subtype of Animal given the species of the animal.
ZooKeeper – The zookeeper admits animals into the zoo and keeps track of the animals in it. It is the only class in this project with a main() method, and the only method in this class is main.
Tasks:
1. Build the 5 enumerations:
a. Birth,
b. BloodTemp,
c. Movement,
d. Respiration,
e. Skin Covering
2. Implement the Animal interface, the AbstractAnimal class and all the subclasses of AbstractAnimal. Put these classes in the package edu.bergen.zoo
3. Implement the AnimalFactory class. Its getInstance() method should return one of:
a. Bird
b. Mammal
c. Reptile
d. Amphibian
e. Fish
f. FlightlessBird
g. SwimmingMammal,
h. DuckBilledPlatypus
based on the value of species provided in the argument list.
Hint#1 – Use an if block, checking on the value of species, and return an object of the appropriate subclass of AbstractAnimal, or
Hint#2 = create a HashMap, where the key is the name of the species, and the value is an object of the appropriate subclass of AbstractAnimal
4. Implement the Zoo class. Put this class in the package edu.zoo
5. Build a ZooKeeper class. In its main() method, do the following tasks:
a. Construct a Zoo object.
b. Create the following ten Animals using AnimalFactory.getInstance(…), and use the Zoo.addAnimal() method in put them in the Zoo. The ten animals are:
i. Eli the Elephant (3000 lbs)
ii. Betty the Boa (20 lbs)
iii. Katerina the Kangeroo (600 lbs)
iv. Voldomir the Vampire (10 lbs)
v. Oksana the Ostrich (300 lbs)
vi. Sophia the Salamander (2 lbs)
vii. Gianluca the Giraffe (800 lbs)
viii. Lisa the Lion (700 lbs)
ix. Samuel the Shark (300 lbs)
x. Harry the Hawk (10 lbs)
c. Print the name and species of all the animals in the zoo that lay eggs.
d. Print the name and species of all the animals in the zoo that are cold blooded
e. Print the name and species of all the animals in the zoo that breathe through their gills
f. Print the name and species of all the animals in the zoo that lay eggs.
g. Print the toString() for the animal named Lisa
h. How many animals are in the zoo?
Step by Step Solution
3.53 Rating (143 Votes )
There are 3 Steps involved in it
Step: 1
Ill provide an outline of how to implement the classes enums and interface described in the question Ill focus on the overall structure and key points ...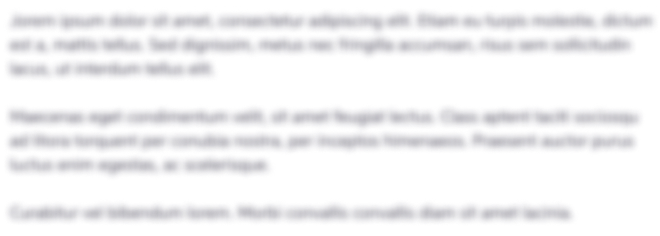
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started