Question
# DO NOT change the name of the MIPS source code. # DO NOT change the name of the MIPS source code. # DO NOT
# DO NOT change the name of the MIPS source code. # DO NOT change the name of the MIPS source code. # DO NOT change the name of the MIPS source code. # Complete the MIPS translation task according the instruction inside the source code file. # DO NOT change the name of the MIPS source code. # DO NOT change the name of the MIPS source code. # DO NOT change the name of the MIPS source code.
#You must translate the update() function, Write the function exactly as described in this handout. #Do not implement the program using other algorithms or tricks. #Do not even switch the order of the arguments #in function calls; you must follow the order specified in the C++ code.
#Your functions should be properly commented. #Each function must have its own header block, including the prototype of the function, #the locations of all arguments and return values, descriptions of the arguments and how they are passed, #and a description of what the function does.
#You should try to make your code efficient. #For example, your loops should follow the efficient form presented in class. #Points will be deducted for obvious inefficiencies.
#Correct register use conventions include having your arguments in $a?, return values in $v?, correct stack handling etc. #Refer to the function specifications in this handout, and Chapter 4 slides.
# You may use the output from the C++ Code to check your work: # cycle 0 # red particle (x,y,xVel,yVel): 4 4 1 1 # green particle (x,y,xVel,yVel): 4 4 -1 -1
# cycle 1 # red particle (x,y,xVel,yVel): 5 5 1 1 # green particle (x,y,xVel,yVel): 3 3 -1 -1
# cycle 2 # red particle (x,y,xVel,yVel): 6 6 1 1 # green particle (x,y,xVel,yVel): 2 2 -1 -1
# cycle 3 # red particle (x,y,xVel,yVel): 7 7 1 1 # green particle (x,y,xVel,yVel): 1 1 -1 -1
# cycle 4 # red particle (x,y,xVel,yVel): 8 8 1 1 # green particle (x,y,xVel,yVel): 0 0 -1 -1
# cycle 5 # red particle (x,y,xVel,yVel): 9 9 1 1 # green particle (x,y,xVel,yVel): 1 1 1 1
# cycle 6 # red particle (x,y,xVel,yVel): 10 10 1 1 # green particle (x,y,xVel,yVel): 2 2 1 1
# cycle 7 # red particle (x,y,xVel,yVel): 9 9 -1 -1 # green particle (x,y,xVel,yVel): 3 3 1 1
# cycle 8 # red particle (x,y,xVel,yVel): 8 8 -1 -1 # green particle (x,y,xVel,yVel): 4 4 1 1
# cycle 9 # red particle (x,y,xVel,yVel): 7 7 -1 -1 # green particle (x,y,xVel,yVel): 5 5 1 1
# Collison: oops, end of simulation! # red particle (x,y,xVel,yVel): 6 6 -1 -1 # green particle (x,y,xVel,yVel): 6 6 1 1
.data redData: .word 0:4 greenData: .word 0:4 prmpt1: .asciiz "Enter x-coordinate for red particle (0 to 10):" prmpt2: .asciiz "Enter y-coordinate for red particle (0 to 10):" prmpt3: .asciiz "Enter x-coordinate for green particle (0 to 10):" prmpt4: .asciiz "Enter y-coordinate for green particle (0 to 10):" prmpt5: .asciiz "cycle " prmpt6: .asciiz "red particle (x,y,xVel,yVel): " prmpt7: .asciiz "green particle (x,y,xVel,yVel): " prmpt8: .asciiz "Collison: oops, end of simulation! " space: .asciiz " " endl: .asciiz " "
.text # void updatePoint(int *arg) # # $a0 arg # $s0 copy of arg # $v0 distance (return value of findDistance())
# Description: # updatePoint() updates the position of a particle in a # 10x10 grid, based on its velocity # particle velocity will change direction on collision with # boundary of grid # arg[0-3]: (x,y) position and (x,y) velocity of a particle
updatePoint: ### ********* ADD CODE BELOW THIS LINE ************ #########
### ********* DO NOT ADD CODE BELOW THIS LINE ************ #########
# int findDistance(int arg0, int arg1, int arg2, int arg3) # # $a0 arg0 # $a1 arg1 # $a2 arg2 # $a3 arg3 # $v0 result # $t0 distX # $t1 distY
# Description: # findDistance() finds the Manhattan distance between 2 points # (arg0, arg1) is (x, y) position of one point # (arg2, arg3) is (x, y) position of other point
findDistance: sub $t0,$a0,$a2 # distX = arg0 - arg2 ; bgez $t0,fin1 # if (distX < 0) mul $t0,$t0,-1 # distX = - distX ; fin1: sub $t1,$a1,$a3 # distY = arg1 - arg3 ; bgez $t1,fin2 # if (distY < 0) mul $t1,$t1,-1 # distY = - distY ; fin2: bltz $a0,retY # if ((arg0 < 0) || (arg2 < 0)) bgez $a2,else retY: move $v0,$t1 # return distY ; j endfind else: bltz $a1,retX # else if ((arg1 < 0) || (arg3 < 0)) bgez $a3,else1 retX: move $v0,$t0 # return distX ; j endfind else1: add $v0,$t0,$t1 # else # return distX + distY ; endfind: jr $31 #} # i $s0 # cycle $s1 = 0 # dist $s2 main: li $s1,0
la $s3,redData # redData[2] = 1 ; li $s4,1 li $t0, 4 sw $t0, 0($s3) sw $t0, 4($s3) sw $s4, 8($s3) sw $s4, 12($s3) # redData[3] = 1 ; la $s3,greenData # greenData[2] = -1 ; li $s4,-1 sw $s4,8($s3) sw $s4,12($s3) # greenData[3] = -1 ; li $t0, 4 sw $t0,($s3) sw $t0,4($s3) loop: # do { la $a0,prmpt5 # cout << "cycle " << cycle << endl ; li $v0,4 syscall move $a0,$s1 li $v0,1 syscall la $a0,endl li $v0,4 syscall la $a0,prmpt6 # cout << "red particle (x,y,xVel,yVel): " li $v0,4 syscall la $s3, redData lw $a0,($s3) # << redData[0] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,4($s3) # << redData[1] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,8($s3) # << redData[2] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,12($s3) # << redData[3] li $v0,1 syscall la $a0,endl # << endl ; li $v0,4 syscall la $a0,prmpt7 # cout << "green particle (x,y,xVel,yVel): " li $v0,4 syscall la $s3, greenData lw $a0,($s3) # << greenData[0] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,4($s3) # << greenData[1] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,8($s3) # << greenData[2] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,12($s3) # << greenData[3] li $v0,1 syscall la $a0,endl # << endl ; li $v0,4 syscall la $a0,endl # << endl ; li $v0,4 syscall
la $a0,redData # updatePoint(redData) ; jal updatePoint la $a0,greenData # updatePoint(greenData) ; jal updatePoint la $s3,redData # dist = findDistance(redData[0], lw $a0,($s3) # redData[1], greenData[0], lw $a1,4($s3) # greenData[1]) ; la $s4,greenData lw $a2,($s4) lw $a3,4($s4) jal findDistance move $s2,$v0 add $s1,$s1,1 # cycle++ ; ble $s2,2,exit # } while ((dist > 2) && (cycle < 10)) ; blt $s1,10,loop exit: bgt $s2,2,end # if (dist <= 2) { la $a0,prmpt8 # cout << prmpt8 ; li $v0,4 syscall la $a0,prmpt6 # cout << "red particle (x,y,xVel,yVel): " li $v0,4 syscall la $s3, redData lw $a0,($s3) # << redData[0] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,4($s3) # << redData[1] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,8($s3) # << redData[2] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,12($s3) # << redData[3] li $v0,1 syscall la $a0,endl # << endl ; li $v0,4 syscall la $a0,prmpt7 # cout << "green particle (x,y,xVel,yVel): " li $v0,4 syscall la $s3, greenData lw $a0,($s3) # << greenData[0] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,4($s3) # << greenData[1] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,8($s3) # << greenData[2] li $v0,1 syscall la $a0,space # << " " li $v0,4 syscall lw $a0,12($s3) # << greenData[3] li $v0,1 syscall la $a0,endl # << endl ; li $v0,4 syscall la $a0,endl # << endl ; li $v0,4 syscall end: li $v0,10 # } syscall #}
C++ CODE that is to be translated
// There is a red particle and a green particle in a box. // The box has (x,y) coordinates 0 <= x <= 10, 0 <= y <= 10. // The user enters initial (x,y) coordinates for the two // particles. The velocity of a particle is given as // an (x,y) pair, where each coordinate is the distance // the particle moves in that direction, in one cycle. // Initially, the red particle starts with velocity (1,1), // and the green particle starts with velocity (-1,-1). // // The program simulates the movement of the two particles. // When a particle reaches a wall, it will bounce // off the wall. When the two particles are too close together, // they will collide and the simulation will end. // // Each particle is represented by an array of four ints. // Element 0 and 1 are the (x,y) coordinates of the particle. // Element 2 and 3 are the (x,y) velocity components of the // particle.
#include using std::cin; using std::cout; using std::endl;
void updatePoint(int *); int findDistance(int, int, int, int);
int main() { int redData[4], greenData[4]; int i,cycle=0, dist;
// set initial conditions
redData[0] = 4; redData[1] = 4; redData[2] = 1; redData[3] = 1; greenData[0] = 4; greenData[1] = 4; greenData[2] = -1; greenData[3] = -1;
do { // display state of particles cout << "cycle " << cycle << endl; cout << "red particle (x,y,xVel,yVel): " << redData[0] << " " << redData[1] << " " << redData[2] << " " << redData[3] << endl; cout << "green particle (x,y,xVel,yVel): " << greenData[0] << " " << greenData[1] << " " << greenData[2] << " " << greenData[3] << endl << endl;
// update particle positions and velocities updatePoint(redData); updatePoint(greenData);
// check distance between particles dist = findDistance(redData[0], redData[1], greenData[0], greenData[1]); cycle++; } while ((dist > 2) && (cycle < 10));
if (dist <= 2) { cout << "Collison: oops, end of simulation! "; cout << "red particle (x,y,xVel,yVel): " << redData[0] << " " << redData[1] << " " << redData[2] << " " << redData[3] << endl; cout << "green particle (x,y,xVel,yVel): " << greenData[0] << " " << greenData[1] << " " << greenData[2] << " " << greenData[3] << endl << endl; }
}
// update position and velocity of particle // arg is array of data for particle
void updatePoint(int *arg) { int distance;
distance = findDistance(arg[0],arg[1],0,-1); if ((distance < 1) && (arg[2] < 0)) arg[2] = - arg[2]; distance = findDistance(arg[0],arg[1],10,-1); if ((distance < 1) && (arg[2] > 0)) arg[2] = - arg[2]; distance = findDistance(arg[0],arg[1],-1,0); if ((distance < 1) && (arg[3] < 0)) arg[3] = - arg[3]; distance = findDistance(arg[0],arg[1],-1,10); if ((distance < 1) && (arg[3] > 0)) arg[3] = - arg[3]; arg[0] = arg[0] + arg[2]; arg[1] = arg[1] + arg[3]; return; }
// find Manhattan distance between two particles, or between // a particle and a wall // (arg0,arg1) are the (x,y) coordinates for first particle/wall // (arg2,arg3) are the (x,y) coordinates for second particle/wall
int findDistance(int arg0, int arg1, int arg2, int arg3) { int distX, distY;
distX = arg0 - arg2; if (distX < 0) distX = - distX; distY = arg1 - arg3; if (distY < 0) distY = - distY; if ((arg0 < 0) || (arg2 < 0)) return distY; else if ((arg1 < 0) || (arg3 < 0)) return distX; else return distX + distY; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
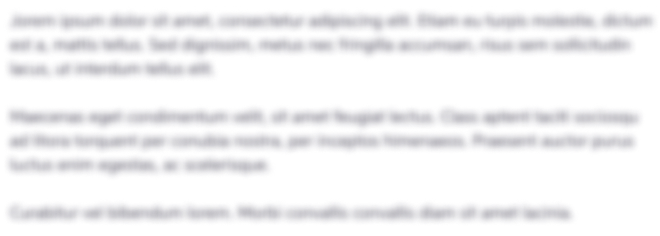
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started