Question
Does anyone know how to fix the Hitboxes in my current code for these characters in Pygame? I don't know how to fix it! I'd
Does anyone know how to fix the Hitboxes in my current code for these characters in Pygame? I don't know how to fix it! I'd like the main character, my christmas tree I created out of shapes, to be able to move using the arrow keys but for some reason it won't work. For the Santa, I just use a santa clipart image from google. Also does anyone know if there is a way to make some sort of score board to show when the santa hits the christmas tree. The christmas tree would be moving trying to avoid the Santa. Any helpjQuery22404215418967491358_1590950779485? Thanks
Here is my current code:
import pygame, sys, random, math
from pygame.locals import *
pygame.init()
SCREENWIDTH = 600
SCREENHEIGHT = 400
WHITE = (255, 255, 255)
SKY_BLUE = (0, 174, 255)
BLUE = ( 0, 0, 255)
BLACK = ( 0, 0,0)
YELLOW = (255, 255, 0)
BROWN = (84, 49, 49)
GREEN = (9, 46, 18)
RED = (255, 0, 0)
PURPLE = (230, 0, 255)
ORANGE = (255, 132, 0)
PINK = (255, 0, 153)
TEAL = (0, 128, 128)
MOON = (230, 211, 168)
def game():
#game variables
global screen
global snow1X, snow1Y, snow2X, snow2Y, snow3X, snow3Y, snow4X, snow4Y, snow5X, snow5Y
snow1X = 250
snow1Y = 0
snow2X = 300
snow2Y = 10
snow3X = 350
snow3Y = 20
snow4X = 200
snow4Y = 50
snow5X = 390
snow5Y = 0
global snow2Speed, snow3Speed
snow2Speed = random.randint(1,3)
snow3Speed = random.randint(1,3)
global treeX, treeY, treeHitBox
treeX = int(SCREENWIDTH/2)
treeY = int(SCREENHEIGHT * .6)
treeHitBox = pygame.rect.Rect(treeX, treeY, 30, 30)
global birdImage, birdX, birdY, birdHitBox
birdImage = pygame.image.load("santaclausclipart.png")
birdX = 10
birdY = int(SCREENHEIGHT/2)
birdHitBox = pygame.Rect(treeX, treeY, 30, 30)
screen = pygame.display.set_mode((SCREENWIDTH, SCREENHEIGHT))
clock = pygame.time.Clock()
fps = 60
#start of the game loop
while True:
#HANDLE ALL THE EVENTS
handleKeyboardEvents()
#UPDATE GAME STATE
moveSnow()
moveBird()
collisions()
#DRAW SCREEN
drawScene()
drawSnow()
drawTree()
drawBird()
pygame.display.update()
clock.tick(fps)
#end of the game loop
def handleKeyboardEvents():
global treeX, treeY
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
key = pygame.key.get_pressed()
if key[pygame.K_UP] and treeY>1:
treeX -= 5
if key[pygame.K_DOWN] and treeY < SCREENHEIGHT:
treeY += 5
if key[pygame.K_LEFT] and treeX>1:
treeX -= 5
if key[pygame.K_RIGHT] and treeX < SCREENWIDTH:
treeX += 5
def collisions():
global treeHitBox, birdHitBox, birdX, birdY
#print (treeHitBox, birdHitBox, treeHitBox.colliderect(birdHitBox))
if treeHitBox.colliderect(birdHitBox):
birdX = -400
birdY = random.randint(0,SCREENHEIGHT)
#move santa claus
def moveBird():
global birdImage, birdX, birdY
birdX = birdX + 5
if birdX > SCREENWIDTH:
birdX = 0
birdY = random.randint (0, SCREENHEIGHT)
#draws penguin image from file
def drawBird():
global birdImage, birdX, birdY
screen.blit(birdImage, (birdX, birdY))
birdHitBox = pygame.rect.Rect(birdX, birdY, 175, 150)
#ths is just for testing to see the hitbox, comment out afterwords
pygame.draw.rect(screen, BLACK, birdHitBox, 1)
#draw the christmas tree
def drawTree():
global screen, treeX, treeY
#draw tree stump
pygame.draw.rect(screen, BROWN, (275, 283, 40, 65),5)
screen.fill(BROWN, rect = (275, 283, 40, 65))
#draw the body of the christmas tree
pygame.draw.polygon(screen, GREEN, ((400, 283), (190, 283), (275, 230), (205, 230), (275, 177), (220, 177), (295, 124), (370, 177), (315, 177), (385, 230), (315, 230), (400, 283)))
#draw the ornaments on tree
pygame.draw.circle(screen, YELLOW, (245, 265), 6, 5)
pygame.draw.circle(screen, BLUE, (295, 255), 6, 5)
pygame.draw.circle(screen, RED, (340, 265), 6, 5)
pygame.draw.circle(screen, PURPLE, (255, 212), 6, 5)
pygame.draw.circle(screen, WHITE, (295, 202), 6, 5)
pygame.draw.circle(screen, ORANGE, (330, 212), 6, 5)
pygame.draw.circle(screen, PINK, (270, 159), 6, 5)
pygame.draw.circle(screen, TEAL, (315, 159), 6, 5)
pygame.draw.circle(screen, YELLOW, (293, 124), 10, 5)
#christmas tree hit box
treeHitBox = pygame.rect.Rect(treeX, treeY, 200, 400)
pygame.draw.rect(screen, BLACK, treeHitBox, 1)
#drawing the scene, snow on the bottom, moon, and a present under the tree
def drawScene():
global screen
screen.fill(SKY_BLUE)
pygame.draw.rect(screen, WHITE, (0, 350, 600, 150),0)
pygame.draw.circle(screen, MOON, (60, 60), 50, 0)
pygame.draw.polygon(screen, PURPLE, ((195, 348), (195, 298), (245, 298), (245, 348)))
pygame.draw.polygon(screen, RED, ((212, 348), (212, 298), (227, 298), (227, 348)))
pygame.draw.polygon(screen, RED, ((195, 330), (195, 315), (245, 315), (245, 330)))
pygame.draw.line(screen, RED, (220, 298), (210, 288), 5)
pygame.draw.line(screen, RED, (220, 298), (230, 288), 5)
#draws snow
def drawSnow():
global screen, snow1X, snow1Y, snow2X, snow2Y, snow3x, snow3Y, snow4X, snow4Y, snow5X, snow5Y
pygame.draw.circle(screen, WHITE, (snow1X, snow1Y), 5, 0)
pygame.draw.circle(screen, WHITE, (snow2X, snow2Y), 5, 0)
pygame.draw.circle(screen, WHITE, (snow3X, snow3Y), 5, 0)
pygame.draw.circle(screen, WHITE, (snow4X, snow4Y), 5, 0)
pygame.draw.circle(screen, WHITE, (snow5X, snow5Y), 5, 0)
#moves snow
def moveSnow():
global screen, snow1X, snow1Y, snow2X, snow2Y, snow3x, snow3Y, snow4X, snow4Y, snow5X, snow5Y
global snow2Speed, snow3Speed
snow1Y = snow1Y + 1
snow2Y = snow2Y + snow2Speed
snow3Y = snow3Y + snow3Speed
snow4Y = snow4Y + 3
snow5Y = snow5Y + 2
if snow1Y > SCREENHEIGHT:
snow1Y = 0
if snow2Y > SCREENHEIGHT:
snow2Y = 0
if snow3Y > SCREENHEIGHT:
snow3Y = 0
snow3Speed = random.randint(1,3)
if snow4Y > SCREENHEIGHT:
snow4Y = 0
if snow5Y > SCREENHEIGHT:
snow5Y = 0
game()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
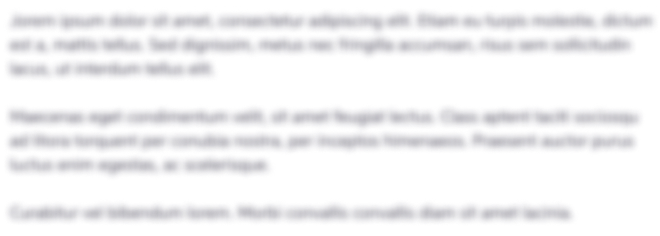
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started