Question
(Done) Part 1: Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields:
(Done) Part 1: Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields: description. The description field references a String object that holds a brief description of the item. unitsOnHand. The unitsOnHand field is an int variable that holds the numbers of units currently in inventory. price. The price filed is a double that holds the item's retail price. Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that returns the values in these fields. (having Issues with) After this, write a method in the RetailItem class called purchaseItem. This method will be used to simulate an item(s) purchase. It will accept as a parameter the variable totalItemsToPurchase representing a number of units to buy (e.g. 10). The method should check if its unitsOnHand is enough to satisfy the purchase. If it is not, notify the user. If it is enough, update unitsOnHand with the new value (unitsOnHand totalItemsToPurchase) and return to the user the total cost of the purchase (itemsToPurchase * price). my code:
package Assignment5;
import java.io.*;
import java.util.Scanner;
//class declaration public class RetailItem {
// variable declaration
private String description; private int units; private double price; private int buyItem;
// default constructor
public RetailItem(){ } //parameterized constructor
public RetailItem(String x, int y, double z){ description = x; units = y; price = z; }
//mutator method implementation public void setDescription(String x){ description = x; }
public void setPrice(double z) { price = z; }
void setUnits(int y) { units = y; }
//accessor methods
public int getUnits(){ return units;
}
public String getDescription(){ return description; }
public double getPrice(){ return price; }
public static void main(String[] args){
String str = "Pants";
RetailItem r1 = new RetailItem("Jacket", 12, 59.95);
RetailItem r2 = new RetailItem("Shoes", 40, 34.95);
RetailItem r3 = new RetailItem();
r3.setDescription(str);
r3.setUnits(-1);
r3.setPrice(24.95);
//print the output
System.out.println("______________________________________");
System.out.println("Description\tUnits on Hand\tPrice\t");
System.out.println("______________________________________");
//call the get methods and diplay it
System.out.println("Item #1\t" + r1.getDescription() + "\t\t" + r1.getUnits() + " \t" + r1.getPrice());
System.out.println("Item #2\t" + r2.getDescription() + "\t\t" + r2.getUnits() + " \t" + r2.getPrice());
System.out.println("Item #3\t" + r3.getDescription() + "\t\t" + r3.getUnits() + " \t" + r3.getPrice());
System.exit(0);
} }
/////Outputs // //__________________________________ //Description Units on Hand Price //__________________________________ //Item #1 Jacket 12 59.95 //Item #2 Designer Jeans 40 34.95 //Item #3 Shirt 20 24.95 //
Step by Step Solution
There are 3 Steps involved in it
Step: 1
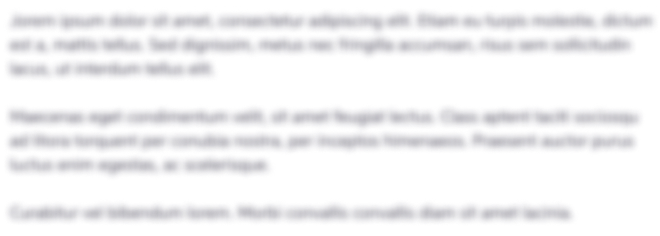
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started