Question
Done quite a bit of it but need help with the last few TODOS for this .H file. Done in C++ TODOS BOLDED #ifndef VECTOR_H
Done quite a bit of it but need help with the last few TODOS for this .H file. Done in C++
TODOS BOLDED
#ifndef VECTOR_H
#define VECTOR_H
#include
#include
#include
#include
template
class Vector {
public:
class iterator;
private:
T* array;
size_t _capacity, _size;
// You may want to write a function that grows the vector
void grow() {
_capacity = (_capacity == 0) ? 1 : 2 * _capacity;
T* temp = new T[_capacity];
std::copy(array, array + _size, temp);
delete[] array;
array = temp;
}
public:
Vector() noexcept : array(nullptr), _capacity(0), _size(0) {}
Vector(size_t count, const T& value): array(new T[count]), _capacity(count), _size(count) {std::fill(array, array + count, value);}
explicit Vector(size_t count) : array(new T[count]), _capacity(count), _size(count) {}
Vector(const Vector& other) : array(new T[other._size]), _capacity(other._size), _size(other._size) { std::copy(other.array, other.array + other._size, array); }
Vector(Vector&& other) noexcept : array(other.array),capacity(other._capacity), _size(other._size) {other.array = nullptr; other._capacity = other._size = 0;}
~Vector() {delete[] array;}
Vector& operator=(const Vector& other) {
if (this != &other) {
T*temp = new T[other._size]; std::copy(other.array, other.array + other._size, temp); delete[] array; array = temp;
_capacity = other._capacity;
_size = other._size;
}
return *this;
}
Vector& operator=(Vector&& other) noexcept {
if (this != &other) {
delete[] array; array = other.array; _capacity = other._capacity; _size = other._size; other.array = nullptr; other._capacity = other._size = 0;
}
return *this;
}
iterator begin() noexcept {return iterator(array); }
iterator end() noexcept { return iterator(array + _size); }
[[nodiscard]] bool empty() const noexcept { return _size == 0;}
size_t size() const noexcept { return _size; }
size_t capacity() const noexcept { return _capacity; }
T& at(size_t pos) {
if (pos >= _size) throw std::out_of_range("out of range");{
return array[pos];
}
}
const T& at(size_t pos) const { /* TODO */ }
T& operator[](size_t pos) { /* TODO */ }
const T& operator[](size_t pos) const { /* TODO */ }
T& front() { /* TODO */ }
const T& front() const { /* TODO */ }
T& back() { /* TODO */ }
const T& back() const { /* TODO */ }
void push_back(const T& value) { /* TODO */ }
void push_back(T&& value) { /* TODO */ }
void pop_back() { /* TODO */ }
iterator insert(iterator pos, const T& value) { /* TODO */ }
iterator insert(iterator pos, T&& value) { /* TODO */ }
iterator insert(iterator pos, size_t count, const T& value) { /* TODO */ }
iterator erase(iterator pos) { /* TODO */ }
iterator erase(iterator first, iterator last) { /* TODO */ }
class iterator {
public:
using iterator_category = std::random_access_iterator_tag;
using value_type = T;
using difference_type = ptrdiff_t;
using pointer = T*;
using reference = T&;
private:
// Add your own data members here
// HINT: For random_access_iterator, the data member is a pointer 99.9% of the time
public:
iterator() { /* TODO */ }
// Add any constructors that you may need
// This assignment operator is done for you, please do not add more
iterator& operator=(const iterator&) noexcept = default;
[[nodiscard]] reference operator*() const noexcept { /* TODO */ }
[[nodiscard]] pointer operator->() const noexcept { /* TODO */ }
// Prefix Increment: ++a
iterator& operator++() noexcept { /* TODO */ }
// Postfix Increment: a++
iterator operator++(int) noexcept { /* TODO */ }
// Prefix Decrement: --a
iterator& operator--() noexcept { /* TODO */ }
// Postfix Decrement: a--
iterator operator--(int) noexcept { /* TODO */ }
iterator& operator+=(difference_type offset) noexcept { /* TODO */ }
[[nodiscard]] iterator operator+(difference_type offset) const noexcept { /* TODO */ }
iterator& operator-=(difference_type offset) noexcept { /* TODO */ }
[[nodiscard]] iterator operator-(difference_type offset) const noexcept { /* TODO */ }
[[nodiscard]] difference_type operator-(const iterator& rhs) const noexcept { /* TODO */ }
[[nodiscard]] reference operator[](difference_type offset) const noexcept { /* TODO */ }
[[nodiscard]] bool operator==(const iterator& rhs) const noexcept {return ptr == rhs.ptr;}
[[nodiscard]] bool operator!=(const iterator& rhs) const noexcept {return ptr != rhs.ptr;}
[[nodiscard]] bool operator<(const iterator& rhs) const noexcept {return ptr < rhs.ptr;}
[[nodiscard]] bool operator>(const iterator& rhs) const noexcept { return ptr > rhs.ptr;}
[[nodiscard]] bool operator<=(const iterator& rhs) const noexcept {return ptr <= rhs.ptr; }
[[nodiscard]] bool operator>=(const iterator& rhs) const noexcept {return ptr <= rhs.ptr; }
};
void clear() noexcept { /* TODO */ }
};
// This ensures at compile time that the deduced argument _Iterator is a Vector
// There is no way we know of to back-substitute template
// because it leads to a non-deduced context
namespace {
template
using is_vector_iterator = std::is_same
}
template
[[nodiscard]] _Iterator operator+(typename _Iterator::difference_type offset, _Iterator const& iterator) noexcept { /* TODO */ }
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
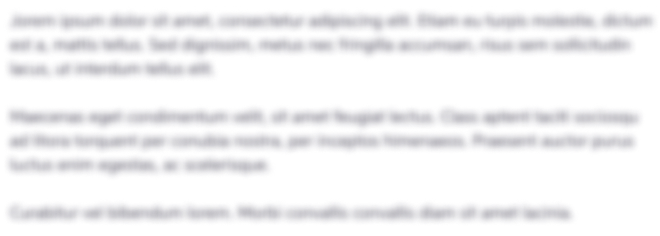
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started