Question
DS Malik Data Structures in C++ 2nd edition Chapter 5 Programming Exercise 14 (Programming Example Video Store) a. Complete the design and implementation of the
DS Malik Data Structures in C++ 2nd edition
Chapter 5 Programming Exercise 14
(Programming Example Video Store)
a. Complete the design and implementation of the class customerType defined in the Programming Example Video Store.
b. Design and implement the class customerListType to create and maintain a list of customers for the video store.
Code to start with:
#include
#include
using namespace std;
class videoType { friend ostream& operator<< (ostream&, const videoType&);
public: void setVideoInfo(string title, string star1, string star2, string producer, string director, string productionCo, int setInStock);
//Function to set the details of a video.
//The private member variables are set according to the
//parameters.
//Postcondition: videoTitle = title; movieStar1 = star1;
// movieStar2 = star2;
movieProducer = producer;
// movieDirector = director;
// movieProductionCo = productionCo;
// copiesInStock = setInStock; int getNoOfCopiesInStock() const;
//Function to check the number of copies in stock.
//Postcondition: The value of copiesInStock is returned. void checkOut();
//Function to rent a video. //Postcondition: The number of copies in stock is
// decremented by one. void checkIn(); //Function to check in a video.
//Postcondition: The number of copies in stock is
// incremented by one.
void printTitle() const;
//Function to print the title of a movie.
void printInfo() const;
//Function to print the details of a video.
//Postcondition: The title of the movie, stars, director,
// and so on are displayed on the screen.
bool checkTitle(string title);
//Function to check whether the title is the same as the
//title of the video.
//Postcondition: Returns the value true if the title is the
// same as the title of the video; false otherwise.
void updateInStock(int num);
//Function to increment the number of copies in stock by
//adding the value of the parameter num.
//Postcondition:
copiesInStock = copiesInStock + num;
void setCopiesInStock(int num);
//Function to set the number of copies in stock.
//Postcondition: copiesInStock = num;
string getTitle() const;
//Function to return the title of the video.
//Postcondition: The title of the video is returned.
videoType(string title = "", string star1 = "", string star2 = "", string producer = "", string director = "", string productionCo = "", int setInStock = 0);
//constructor
//The member variables are set according to the
//incoming parameters. If no values are specified, the
//default values are assigned.
//Postcondition:
videoTitle = title; movieStar1 = star1;
// movieStar2 = star2;
movieProducer = producer;
// movieDirector = director;
// movieProductionCo = productionCo;
// copiesInStock = setInStock;
//Overload the relational operators.
bool operator==(const videoType&) const;
bool operator!=(const videoType&) const; private: string videoTitle;
//variable to store the name of the movie string movieStar1;
//variable to store the name of the star string movieStar2;
//variable to store the name of the star string movieProducer;
//variable to store the name of the
//producer string movieDirector;
//variable to store the name of the
//director string movieProductionCo;
//variable to store the name
//of the production company int copiesInStock;
//variable to store the number of
//copies in stock };
The definitions of these functions, as shown here, are quite straightforward and easy to follow:
void videoType::setVideoInfo(string title, string star1, string star2, string producer, string director, string productionCo, int setInStock)
{ videoTitle = title; movieStar1 = star1; movieStar2 = star2; movieProducer = producer; movieDirector = director; movieProductionCo = productionCo; copiesInStock = setInStock; }
string videoType::getTitle() const { return videoTitle; }
videoType::videoType(string title, string star1, string star2, string producer, string director, string productionCo, int setInStock)
{ setVideoInfo(title, star1, star2, producer, director, productionCo, setInStock);
}
bool videoType::operator==(const videoType& other)
const { return (videoTitle == other.videoTitle);
}
bool videoType::operator!=(const videoType& other)
const { return (videoTitle != other.videoTitle);
}
ostream& operator<< (ostream& osObject, const videoType& video)
{ osObject << endl;
osObject << "Video Title: " << video.videoTitle << endl;
osObject << "Stars: " << video.movieStar1 << " and " << video.movieStar2 << endl;
osObject << "Producer: " << video.movieProducer << endl;
osObject << "Director: " << video.movieDirector << endl;
osObject << "Production Company: " << video.movieProductionCo << endl;
osObject << "Copies in stock: " << video.copiesInStock << endl; osObject << "__________" << endl;
return osObject;
}
The definition of the class videoListType is as follows: //*****************************************************************
// Author: D.S. Malik // // class videoListType // This class specifies the members to implement a list of videos. //*****************************************************************
#include
#include "unorderedLinkedList.h"
#include "videoType.h"
using namespace std;
class videoListType:
public unorderedLinkedList
{ public: bool videoSearch(string title) const;
//Function to search the list to see whether a
//particular title, specified by the parameter title,
//is in the store.
//Postcondition: Returns true if the title is found, and
// false otherwise. bool isVideoAvailable(string title) const;
//Function to determine whether a copy of a particular
//video is in the store.
//Postcondition: Returns true if at least one copy of the
// video specified by title is in the store, and false
// otherwise.
void videoCheckOut(string title);
//Function to check out a video, that is, rent a video.
//Postcondition: copiesInStock is decremented by one. void videoCheckIn(string title);
//Function to check in a video returned by a customer.
//Postcondition: copiesInStock is incremented by one. bool videoCheckTitle(string title) const;
//Function to determine whether a particular video is in
//the store.
//Postcondition: Returns true if the videos title is the
// same as title, and false otherwise.
void videoUpdateInStock(string title, int num);
//Function to update the number of copies of a video
//by adding the value of the parameter num. The
//parameter title specifies the name of the video for
//which the number of copies is to be updated.
//Postcondition:
copiesInStock = copiesInStock + num;
void videoSetCopiesInStock(string title, int num);
//Function to reset the number of copies of a video.
//The parameter title specifies the name of the video
//for which the number of copies is to be reset, and the
//parameter num specifies the number of copies.
//Postcondition:
copiesInStock = num;
void videoPrintTitle() const;
//Function to print the titles of all the videos in the store.
private: void searchVideoList(string title, bool& found, nodeType
//This function searches the video list for a particular
//video, specified by the parameter title.
//Postcondition: If the video is found, the parameter found is
// set to true, otherwise it is set to false. The parameter
// current points to the node containing the video.
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
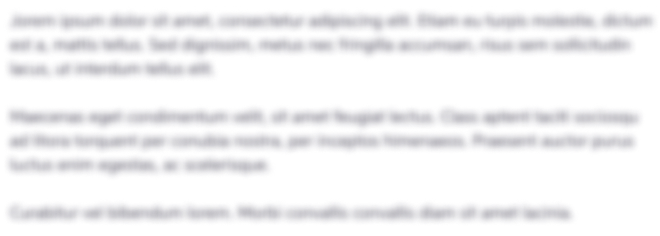
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started