Question
Easy C Problem (STACK2 is located below) Just add the three member functions to the already completed STACK2. Once complete, take screenshot of the compiled
Easy C Problem (STACK2 is located below)
Just add the three member functions to the already completed STACK2. Once complete, take screenshot of the compiled output.
(1) Add OutputSTACK() as a new member function to the STACK2 abstract data type.
//--------------------------------------------------
void OutputSTACK(const STACK *stack,FILE *OUT)
//--------------------------------------------------
{
fprintf(OUT,"[%d:%d] ",GetSizeSTACK(stack),GetCapacitySTACK(stack));
if ( IsEmptySTACK(stack) )
printf("(empty)");
else
{
int offset;
int UB = GetSizeSTACK(stack)-1;
for (offset = 0; offset <= UB; offset++)
fprintf(OUT,"%d%c",PeekSTACK(stack,offset),((offset == UB) ? '.' : ' '));
}
}
(2) Add CopySTACK() as a new member function to STACK2.
//--------------------------------------------------
void CopySTACK(STACK *LHS,const STACK *RHS)
//--------------------------------------------------
{
if ( LHS != RHS )
{
int offset;
DestructSTACK(LHS);
ConstructSTACK(LHS,RHS->capacity);
Student provides missing code to traverse *RHS, PeekSTACK()-ing all elements
contained in *RHS, PushSTACK()-ing each element, in turn, on to *LHS.
}
}
(3) Add EQSTACK() as a new member function to STACK2.
//--------------------------------------------------
bool EQSTACK(const STACK *LHS,const STACK *RHS)
//--------------------------------------------------
{
bool r;
int offset;
if ( LHS->size != RHS->size ) return( false );
Student provides missing code to traverse *LHS and *RHS, PeekSTACK()-ing
corresponding elements to ensure every pair of corresponding elements are
equal; that is, compute r with the following universal quantified proposition
r "offset [0,size-1] ( PeekSTACK(LHS,offset) = PeekSTACK(RHS,offset) )
return( r );
}
(4) Take screenshot of compiled output
//----------------------------------------------------
// STACK2.h
//----------------------------------------------------
#ifndef STACK2_H
#define STACK2_H
#include
//==============================================================
// Data model definitions
//==============================================================
typedef struct STACK
{
int size;
int capacity;
int *elements;
} STACK;
//==============================================================
// Public member function prototypes
//==============================================================
void ConstructSTACK(STACK *stack,const int capacity);
void DestructSTACK(STACK *stack);
void PushSTACK(STACK *stack,const int element);
void PopSTACK(STACK *stack);
int PeekSTACK(const STACK *stack,const int offset);
int GetSizeSTACK(const STACK *stack);
int GetCapacitySTACK(const STACK *stack);
bool IsFullSTACK(const STACK *stack);
bool IsEmptySTACK(const STACK *stack);
int PeekSTACK2(const STACK *stack,const int offset,bool *exception);
int PeekSTACK3(const STACK *stack,const int offset);
//==============================================================
// Private utility member function prototypes
//==============================================================
// (none)
#endif
//--------------------------------------------------
// STACK2.c
//--------------------------------------------------
#include
#include
#include
#include ".\Stack2.h"
#include "..\ADTExceptions.h"
//--------------------------------------------------
void ConstructSTACK(STACK *stack,const int capacity)
//--------------------------------------------------
{
if ( capacity <= 0 ) RaiseADTException(STACK_CAPACITY_ERROR);
stack->size = 0;
stack->capacity = capacity;
stack->elements = (int *) malloc(sizeof(int)*capacity);
if ( stack->elements == NULL ) RaiseADTException(MALLOC_ERROR);
}
//--------------------------------------------------
void DestructSTACK(STACK *stack)
//--------------------------------------------------
{
free(stack->elements);
}
//--------------------------------------------------
void PushSTACK(STACK *stack,const int element)
//--------------------------------------------------
{
if ( IsFullSTACK(stack) ) RaiseADTException(STACK_OVERFLOW);
stack->elements[stack->size] = element;
++stack->size;
}
//--------------------------------------------------
void PopSTACK(STACK *stack)
//--------------------------------------------------
{
if ( IsEmptySTACK(stack) ) RaiseADTException(STACK_UNDERFLOW);
stack->size--;
}
//--------------------------------------------------
int PeekSTACK(const STACK *stack,const int offset)
//--------------------------------------------------
{
if ( !((0 <= offset) && (offset <= GetSizeSTACK(stack)-1)) ) RaiseADTException(STACK_OFFSET_ERROR);
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------
int GetSizeSTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->size );
}
//--------------------------------------------------
int GetCapacitySTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->capacity );
}
//--------------------------------------------------
bool IsFullSTACK(const STACK *stack)
//--------------------------------------------------
{
return( (stack->size == stack->capacity) ? true : false );
}
//--------------------------------------------------
bool IsEmptySTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->size == 0 );
}
//--------------------------------------------------
int PeekSTACK2(const STACK *stack,const int offset,bool *exception)
//--------------------------------------------------
{
if ( !((0 <= offset) && (offset <= GetSizeSTACK(stack)-1)) )
{
*exception = true;
return( 0 );
}
*exception = false;
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------
int PeekSTACK3(const STACK *stack,const int offset)
//--------------------------------------------------
{
if ( !((0 <= offset) && (offset <= GetSizeSTACK(stack)-1)) )
{
fprintf(stderr,"\a Exception \"%s\" ",STACK_OFFSET_ERROR);
system("PAUSE");
exit( 1 );
}
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------------------
// ADTExceptions.h
//--------------------------------------------------------------
#ifndef ADTEXCEPTIONS_H
#define ADTEXCEPTIONS_H
// ADT exception definitions
#define MALLOC_ERROR "malloc() error"
#define DATE_ERROR "DATE error"
#define STACK_CAPACITY_ERROR "STACK capacity error"
#define STACK_UNDERFLOW "STACK underflow"
#define STACK_OVERFLOW "STACK overflow"
#define STACK_OFFSET_ERROR "STACK offset error"
// ADT exception-handler prototype
void RaiseADTException(char exception[]);
#endif
//--------------------------------------------------------------
// ADTExceptions.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\ADTExceptions.h"
//--------------------------------------------------------------
void RaiseADTException(char exception[])
//--------------------------------------------------------------
{
fprintf(stderr,"\a Exception \"%s\" ",exception);
system("PAUSE");
exit( 1 );
}
//--------------------------------------------------------------
// STACK ADT Problem #2
// Problem2.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\STACK2.h"
#include "..\ADTExceptions.h"
//--------------------------------------------------------------
int main()
//--------------------------------------------------------------
{
int capacity;
printf("capacity? ");
while ( scanf("%d",&capacity) != EOF )
{
STACK stack;
int i,pushN,popN,peekN;
ConstructSTACK(&stack,capacity);
printf("pushN? "); scanf("%d",&pushN);
printf("peekN? "); scanf("%d",&peekN);
printf(" popN? "); scanf("%d",&popN);
for (i = 1; i <= pushN; i++)
PushSTACK(&stack,i);
/*
Method (1) Allow ADT to RaiseADTException() which provides a uniform
exception-handling approach for *ALL* ADT
*/
for (i = 1; i <= peekN; i++)
printf("%d ",PeekSTACK(&stack,i-1));
printf(" ");
/*
Method (2) ADT returns exception status (true or false) then client code
checks status after *EVERY* ADT exception-producing operation call
for (i = 1; i <= peekN; i++)
{
bool exception;
int x = PeekSTACK2(&stack,i-1,&exception);
if ( exception )
{
fprintf(stderr,"\a Exception \"%s\" ",STACK_OFFSET_ERROR);
system("PAUSE");
exit( 1 );
}
printf("% d",x);
}
printf(" ");
*/
/*
Method (3) ADT handles the exception (identical to Method (1)
from clients point-of-view)
for (i = 1; i <= peekN; i++)
printf("%d ",PeekSTACK3(&stack,i-1));
printf(" ");
*/
for (i = 1; i <= popN; i++)
PopSTACK(&stack);
DestructSTACK(&stack);
printf(" capacity? ");
}
system("PAUSE");
return( 0 );
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
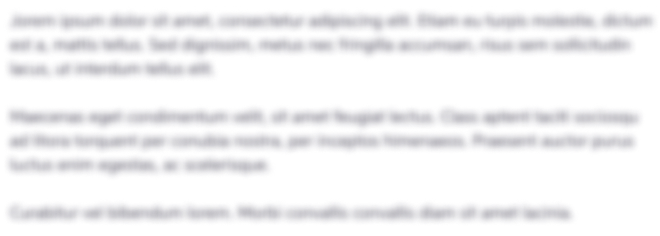
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started