Question
EDIT THE FOLLOWING CODE IN JAVA TO INCLUDE THE FOLLOWING PART A: Add a method void animSort() that sorts the animals in the animals array
EDIT THE FOLLOWING CODE IN JAVA TO INCLUDE THE FOLLOWING
PART A: Add a method void animSort() that sorts the animals in the animals array based on their energy. You must use the Arrays.sort method in your implementation. For example, suppose that
animals = [Cow1, Chicken1, Llama1, null, null, ..., null] and assume that the order based on the energy is Llama1 < Chicken1 < Cow1. Then, animSort would change animals to:
animals = [Llama1, Chicken1, Cow1, null, null, ..., null]
PART B: Implement any changes outside the Farm class in order for animSort() to work properly.
PART C: Add a method boolean addClone(Animal anim) that clones the given animal, anim, and adds it to the animals array. For example, if animals has 4 animals and we call myFarm.addClone(animals[2]), a clone of animals[2] would be created and added to animals at the next available spot. The method addClone returns true if the animal is added successfully to animals and false if the farm is full.
PART D: Implement any changes outside the Farm class in order for addClone to work properly.
BELOW IS THE ORIGINAL CODE:
public class Animal { private String name; private double energy, mealAmount, x, y, speedX=1, speedY=1; private boolean alive; public Animal() { setEnergy(100); } public void speak(String msg){ if (isAlive()) System.out.println(getName() + " says: " + msg); } public double eat(){ if (isAlive()) { //get amount needed and round to 2 decimals double amount = Math.round((100-getEnergy())*100)/100.0; if (amount >= mealAmount) { System.out.println(getName() + " ate " + mealAmount + " units"); setEnergy(getEnergy() + mealAmount); return mealAmount; } else if (amount > 0) { System.out.println(getName() + " ate " + amount + " units. Now it is full!"); setEnergy(100); return amount; } else { System.out.println(getName() + " didn't eat. It is full!"); return 0; } } else { System.out.println(getName() + " is dead!"); return 0; } } public void move() { if(isAlive()){ x += speedX; y += speedY; setEnergy(getEnergy() - 0.1); }else System.out.println(getName() + "can't move. It is dead!"); } public void sound(){if(isAlive()) System.out.println("Unknown sound!");} //setters, getters, toString public String getName() { return name; } public double getEnergy() { return energy; } public void setName(String name) { this.name = name; } public void setEnergy(double energy) { if(energy>0 && energy <=100) this.energy = energy; if(this.energy <= 17 ) System.out.println(getName() + " says: I'm STARVING"); else if(this.energy <= 50) System.out.println(getName() + " says: I'm hungry"); this.alive = (energy > 0); } public double getMealAmount() { return mealAmount; } public void setMealAmount(double mealAmount) { if(mealAmount>0 && mealAmount<100) this.mealAmount = mealAmount; } public double getX() { return x; } public void setX(double x) { this.x = x; } public double getY() { return y; } public void setY(double y) { this.y = y; } public double getSpeedX() { return speedX; } public void setSpeedX(double speedX) { this.speedX = speedX; } public double getSpeedY() { return speedY; } public void setSpeedY(double speedY) { this.speedY = speedY; } public boolean isAlive() { return alive; } public String toString(){ //return String.format("Alive:%b Name:%-10sEnergy:%-7.1fLocation:(%-2.1f,%-2.1f)", isAlive(), name, energy,x,y); return String.format("%-8s: %-5s at (%-2.1f,%-2.1f) Energy=%-7.1f", name, isAlive()?"alive":"dead",x,y,energy); } }
public class Chicken extends Animal{ private static int id; public Chicken() { setName("Chicken" + ++id); setMealAmount(5); } public void sound(){ if(isAlive()) System.out.println("Cluck!"); } public void swim(){ if(isAlive()) System.out.println("Chicken can swim as following:...!"); } }
public class Cow extends Animal{ private static int id; public Cow() { setName("Cow" + ++id); setMealAmount(20); } public void sound(){ if(isAlive()) System.out.println("Moo!"); } public void milk(){ if(isAlive()) System.out.println("We can milk cows as following:...!"); } }
public class Farm { private double availableFood; private Animal[] animals; public Farm() { setAvailableFood(1000); animals = new Animal[4]; animals[0] = new Chicken(); animals[1] = new Cow(); animals[2] = new Llama(); animals[3] = new Llama(); } public void makeNoise(){ // all animals make their sound (Moo, Cluck, etc) for(Animal animal: animals) animal.sound(); } public void feedAnimals(){ // restore energy of all animals and deduct amount eaten from availableFood for(Animal animal : animals) if(availableFood >= Math.min(animal.getMealAmount(), (100-animal.getEnergy()))) // no penalty if student uses: if(availableFood >= animal.getMealAmount()) availableFood -= animal.eat(); else System.out.println("Not enough food for your animals! You need to collect more food items."); } public double getAvailableFood() { return availableFood; } public void setAvailableFood(double availableFood) { if(availableFood>=0 && availableFood<=1000) this.availableFood = availableFood; } public Animal[] getAnimals() { return animals; } }
public class Llama extends Animal{ private static int id; public Llama() { setName("Llama" + ++id); setMealAmount(9); } public void sound(){ if(isAlive()) System.out.println("Hmmm!"); } public void jump(){ if(isAlive()) System.out.println("Llamas can jump as following:...!"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
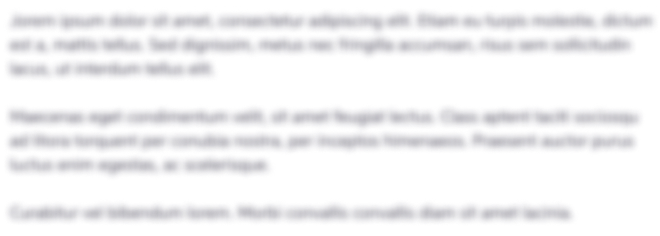
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started