Question
employee.h ---------- #include #include #include class Employee { private: int employeeNum; std::string name; std::string address; std::string phoneNum; double hrWage, hrWorked; public: Employee(int en, std::string n,
employee.h ----------
#include
class Employee { private: int employeeNum; std::string name; std::string address; std::string phoneNum; double hrWage, hrWorked;
public: Employee(int en, std::string n, std::string a, std::string pn, double hw, double hwo); std::string getName(); void setName(std::string n); int getENum(); std::string getAdd(); void setAdd(std::string a); std::string getPhone(); void setPhone(std::string p); double getWage(); void setWage(double w); double getHours(); void setHours(double h); double calcPay(double a, double b); static Employee read(std::ifstream& in); void write(std::ofstream& out); };
employee.cpp ---------- //employee.cpp
#include "employee.h" #include
Employee::Employee(int en, std::string n, std::string a, std::string pn, double hw, double hwo) { this->employeeNum = en; this->name = n; this->address = a; this->phoneNum = pn; this->hrWage = hw; this->hrWorked = hwo;
}
string Employee::getName() { return name; }
void Employee::setName(string n) { this->name = n; }
int Employee::getENum() { return employeeNum; }
string Employee::getAdd() { return address; }
void Employee::setAdd(string a) { this->address = a; }
string Employee::getPhone() { return phoneNum; }
void Employee::setPhone(string p) { this->phoneNum = p; }
double Employee::getWage() { return hrWage; }
void Employee::setWage(double w) { this->hrWage = w; }
double Employee::getHours() { return hrWorked; }
void Employee::setHours(double h) { this->hrWorked = h; }
double Employee::calcPay(double a, double b) { const double x = 40.00; double gross; if(b > 40) { b -= x; gross = (a * x) + ((a + (a / 2)) * b); } else { gross = a * b; } double net = gross - (gross * .2) - (gross * .075); return net; }
Employee Employee::read(ifstream &in){ string name, addr, phone; double wage, hours; int id;
in >> id; if(in.fail()) throw runtime_error("end of file");
in.ignore(); //get rid of newline before using getline() getline(in, name); getline(in, addr); getline(in, phone); in >> wage >> hours;
if(in.fail()) throw runtime_error("end of file");
return Employee(id, name, addr, phone, wage, hours); }
void Employee::write(ofstream &out) { out
main.cpp ========
#include "employee.h" #include
void PrintCalc(Employee a) {
cout
void createFile() { string filename; Employee joe(37, "Joe Brown", "123 Main St.", "123-6788", 45.00, 10.00); Employee sam(21, "Sam Jones", "45 East State", "661-9000", 30.00, 12.00); Employee mary(15, "Mary Smith", "12 High Street", "401-8900", 40.00, 15.00);
cout > filename;
ofstream out(filename.c_str()); joe.write(out); sam.write(out); mary.write(out);
out.close(); cout
}
void readFile() { string filename; cout > filename;
ifstream in(filename.c_str()); if(in.fail()) throw runtime_error("Couldn't open file for input"); else { for(int i = 0; i
} int main(int argc, const char * argv[]) { int choice = 0;
cout > choice;
try { if(choice == 1) createFile(); else if(choice == 2) readFile(); else cout
return 0; }
I put in this code and I keep getting these errors need to check to see what I am putting in wrong
1: Constructor and Getters 0/5 Tests the parameterized constrictor and "Getter functions Compilation failed main.cpp: In function 'bool testPassed (std::ofstream&) main.cpp: 70:10: error: class Employee'has no member named 'getEmploye if (emp.getEmployeeNumber )!-123) main.cpp:82:10: error: 'class Employee' has no member named 'getAddr' if (emp.getAddr-"Cohasset, MA") Compilation failed main.cpp:94:10: error: class Employee' has no member named 'getHourlyW if (emp.getHourlyWage ) 1000000.00) main.cpp:100:10: error 'class Employee' has no member named 'getHoursW if (emp.getHoursWorked)12.0) 2: Setters 0/5 Tests all Setters of Employee class Compilation failed main.cpp: In function 'bool testPassed (std::ofstream&) main.cpp: 71:6: error: class Employee has no member named 'setAddr' d emp.setAddr ("Braintree, MA" main.cpp: 73:6: error: class Employee has no member named 'setHourlyWa emp.setHourlyWage (0.99); main n.cpp: emp.setHoursWorked (5000.0) :74:6: error: class Employee' has no member named setHoursWorl Compilation failed main.cpp: 83:10: error: 'class Employee' has no member named 'getAddr if (emp.getAddr - "Braintree, MA") main.cpp: 95:10: error:'class Employee' has no member named getHourlyW if (emp.getHourlyWage() 0.99) 101:10: error class Employee' has no member named 'getHoursWo if (emp.getHoursWorked) -5000.0) 3: calcPay 075 Test calcPay for both regular and overtime hours Compilation failed main.cpp: In function 'bool testPassed (std::ofstream&) main.cpp: 71:18: error: no matching function for call to 'Employee::calcl if (emp.calcPay)!8700.0) In file included from main.cpp:2:0 employee.h:26:8: note: candidate: double Employee::calcPay (double, doub double calcPay (double a, double b) ; emplovee.h:26:8: note:candidate expects 2 arguments, 0 provided error class Employee' has no member named 'setHoursWorl Compilation failed ain.cpp: 78:6: emp.setHoursWorked (43.5); main.cpp: 79:18: error: no matching function for call to 'Employee::calcl if (emp.calcPay)!32806.25) In file included from main.cpp:2:0 employee.h:26:8: note: candidate: double Employee::calcPay (double, doub double calcPay (double a, double b) ; emplovee.h:26:8: note:candidate expects 2 arguments, 0 provided 4: read0 and write0 0/10 Tests the write0 and static read functions Compilation failed main.cpp: In function 'bool testPassed (std::ofstream&) : 82:10: error: 'class Employee' has no member named 'getEmploye in.cpp if (emp.getEmployeeNumber (123) main.cpp: 94:10: error: 'class Employee' has no member named 'getAddr if (emp.getAddr-"Cohasset MA") Compilation failed 106:10: error class Employee' has no member named 'getHourly if (emp.getHourlyWage()1000.00) :112:10: error class Employee' has no member named 'getHoursWo if (emp.getHoursWorked) !- 12.0)Step by Step Solution
There are 3 Steps involved in it
Step: 1
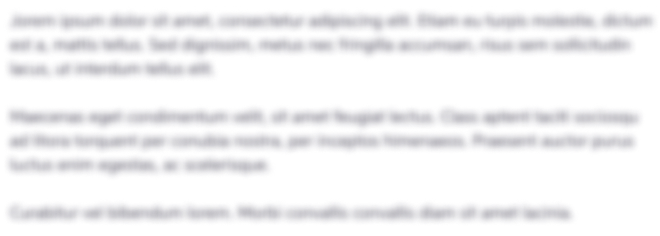
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started