Question
Enhance this approach by creating a BlackJack game between two virtual players. The cards have the values given in Program9-1 and Program9-1B, with the following
Enhance this approach by creating a BlackJack game between two virtual players. The cards have the values given in Program9-1 and Program9-1B, with the following caveat: oAces will take the value of 11 as long as the sum total of the cards in a persons hand does not exceed 21. oIf the sum total does exceed 21, the Ace will take the value of 1.
HERE IS THE PROGRAM:
import random def main():# Create a deck of cards. deck = create_deck() hand = {} # Deal the cards. print(Here are your first two cards: ) deal_cards(deck, 2, hand) lose = False hit = input('Do you want a hit (Y or N): ') while (hit == 'Y' or hit == 'y') and not lose: lose = deal_cards(deck, 1, hand) if not lose: hit = input('Do you want another hit (Y or N): ') else: print('You went over. You lose!') exit = input('')
def create_deck(): # Create a dictionary with each card and its value # stored as key-value pairs. deck = {'Ace of Spades':1, '2 of Spades':2, '3 of Spades':3, '4 of Spades':4, '5 of Spades':5, '6 of Spades':6, '7 of Spades':7, '8 of Spades':8, '9 of Spades':9, '10 of Spades':10, 'Jack of Spades':10, 'Queen of Spades':10, 'King of Spades': 10, 'Ace of Hearts':1, '2 of Hearts':2, '3 of Hearts':3, '4 of Hearts':4, '5 of Hearts':5, '6 of Hearts':6, '7 of Hearts':7, '8 of Hearts':8, '9 of Hearts':9, '10 of Hearts':10, 'Jack of Hearts':10, 'Queen of Hearts':10, 'King of Hearts': 10, 'Ace of Clubs':1, '2 of Clubs':2, '3 of Clubs':3, '4 of Clubs':4, '5 of Clubs':5, '6 of Clubs':6, '7 of Clubs':7, '8 of Clubs':8, '9 of Clubs':9, '10 of Clubs':10, 'Jack of Clubs':10, 'Queen of Clubs':10, 'King of Clubs': 10, 'Ace of Diamonds':1, '2 of Diamonds':2, '3 of Diamonds':3, '4 of Diamonds':4, '5 of Diamonds':5, '6 of Diamonds':6, '7 of Diamonds':7, '8 of Diamonds':8, '9 of Diamonds':9, '10 of Diamonds':10, 'Jack of Diamonds':10, 'Queen of Diamonds':10, 'King of Diamonds': 10} # Return the deck. return deck
def deal_cards(deck, number, hand): # Initialize an accumulator for the hand value. hand_value = 0 over = False # Make sure the number of cards to deal is not # greater than the number of cards in the deck. if number > len(deck): number = len(deck) # Deal the cards and accumulate their values. for count in range(number): card = random.choice(list(deck.keys())) # Use these two lines instead of: value = deck.pop(card) # card, value = deck.popitem() hand[card] = value print(hand) for val in hand.values(): if val == 1: val = int(input('What value do you want to give the Ace(11 or 1)? ')) hand_value += val # Display the value of the hand. print('Value of this hand:', hand_value) if hand_value > 21: over = True return over # Call the main function. main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
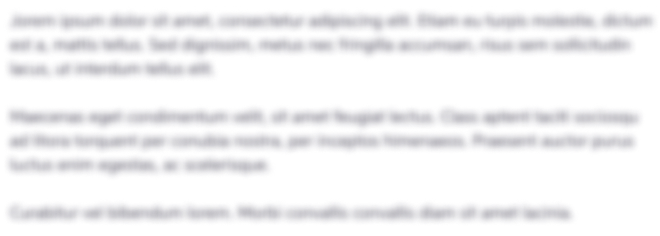
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started