Question
Exception Handling and Design You will be designing and implementing a very simple version of the game Sudoku for a single 3x3 square. Specifically, you
Exception Handling and Design
You will be designing and implementing a very simple version of the game Sudoku for a single 3x3 square. Specifically, you will design and implement a SudokuGame support class that stores all actual information about the state of the game, and detects and throws exceptions when problems occur. You will also modify a command-line application that uses that support class to handle those exceptions.
Basic Rules of Simple Sudoku
Our simplified version of Sudoku uses a single 3x3 board (unlike the real thing which contains 9 of them). The goal is to place the numbers 1 through 9 in the squares, so that:
Each number appears exactly once. Each of the three rows add up to 15. Each of the three columns add up to 15.
Numbers are prompted for and added one at a time:
Current board:
+------+
| |
| |
| |
+------+
Enter row (0 - 2): 1
Enter column (0 - 2): 0
Enter number to place there: 3
Current board:
+------+
| |
|3 |
| |
+------+
Enter row (0 - 2): 0
Enter column (0 - 2): 2
Enter number to place there: 6
Current board:
+------+
| 6 |
|3 |
| |
+------+
Enter row (0 - 2): 1
Enter column (0 - 2): 1
Enter number to place there: 5
Current board:
+------+
| 6 |
|3 5 |
| |
+------+
Enter row (0 - 2): 2
Enter column (0 - 2): 2
Enter number to place there: 7
Current board:
+------+
| 6 |
|3 5 |
| 7 |
+------+
Enter row (0 - 2): 2
Enter column (0 - 2): 0
Enter number to place there: 4
Numbers can also be removed. If the user specifies a row and column that already contains a number, that number is removed from the board:
Enter row (0 - 2): 2
Enter column (0 - 2): 2
Current board:
+------+
| 6 |
|3 5 |
|4 |
+------+
An error message should be displayed instead if placing a number would violate any of these rules. Specifically:
Adding the third number to a row should not give a total either less than or greater than 15.
Adding the third number to a column should not give a total either less than or greater than 15.
A number must not be added if it is already on the board.
Enter row (0 - 2): 0
Enter column (0 - 2): 0
Enter number to place there: 7
That would give an illegal total for that column!
Current board:
+------+
| 6 |
|3 5 |
|4 |
+------+
Enter row (0 - 2): 1
Enter column (0 - 2): 2
Enter number to place there: 9
That would give an illegal total for that row!
Current board:
+------+
| 6 |
|3 5 |
|4 |
+------+
Enter row (0 - 2): 2
Enter column (0 - 2): 2
Enter number to place there: 3
You have already used that number!
The SudokuGame Support Class This class represents the current state of a 3x3 simple Sudoku board. The simplest was to do this is a two dimensional array.
Constructing such an array is similar to a one dimensional array:
type[][] arrayname = new type[3][3];
You should think about what type you would store in each element of the array (there are a couple of good choices.
You should also think about how you would represent an empty square.
Required Constructors and Methods
Your SudokuGame support class is required to have the following constructors and methods:
public SudokuGame() This constructor should create a new game with an empty board. public String getValue(int row, int column)
This inspector method returns the value stored at [row][column]. Note that it is to do so as a String. Also note that it should return a space (that is, ) if the square is empty.
public void remove(int row, int column)
This modifier method should remove the number at the location [row][column], replacing it with a space.
public void place(int row, int column, int number)
This modifier method should place the value number at the location [row][column]. It should also do validation on the board, throwing one of the following exceptions if that number would violate the rules given above:
o RowException if it is the third number in the row, and the total is not exactly 15.
o ColumnException if it is the third number in the column, and the total is not exactly 15.
o NumberAlreadyUsedException if the number is already on the board.
public boolean completed() This inspector returns true if all 9 numbers have been successfully placed, false otherwise. Important design note:
Your SudokuGame methods will only throw exceptions. They will not be catching any exceptions. All exceptions will be caught within the main method of the SudokuApp class.
Designing Additional Exceptions
The above situations are not the only in which an exception can occur. You are to look over your SudokuGame class methods and constructors and identify at least three other instances where it would be appropriate for an exception to be thrown. This is the main design exercise of this assignment. In each case, create an appropriate exception class, and modify the code to throw the exception if it occurs.
The SudokuApp Application Class
I have provided a simple command line application called SudokuApp for running your support class. When run (with a working SudokuGame class), it produces the type of output given above.
Carefully examine the main method in the application to see how this works, particularly with respect to how the place method is called.
Handling SudokuGame Exceptions
Note that there is currently no exception handling in this method. You are to modify this code to handle the three exceptions that may be thrown by the place method. In each case, it should show an approriate error message, as shown in the examples above.
Note: The application is to be modified to display the exception. That is, the SudokuGame class is not to display anything. Nor is it to pass the message to be displayed.
Handling Other Exceptions
Aside from the exceptions thrown by the place method, there is one other type of exception that might be thrown in the main loop in this application due to player error. Add code to catch this exception and to display an appropriate messge.
Hint: When you play the game, think about what unusual input a player could try to enter.
Documentation
Be sure to document the exception handling and testing components of your code. Specifically:
Document each method of your SudokuGame class, particularly the sections related to detecting and throwing exceptions. You are to use Javadoc format for this.
Document the changes you make to the main method related to handling the exceptions thrown by SudokuGame methods.
package sudoku; import java.util.*;
Application to run the SimpleSudoku game
public class SudokuApp { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Construct a new instance of the support class and dispaly it. SudokuGame game = new SudokuGame(); display(game); // Continue until the player has successfully entered values for the // entire square. while (!game.completed()) { /********** The code you will need to modify begins here *********/ // Prompt for the row and column (parsing to integers) System.out.print("Enter row (0 - 2): "); int row = Integer.parseInt(scanner.nextLine()); System.out.print("Enter column (0 - 2): "); int col = Integer.parseInt(scanner.nextLine()); // If that square is empty, prompt for a number and put it in // that square. if (game.isEmpty(row, col)) { System.out.print("Enter number to place there: "); int number = Integer.parseInt(scanner.nextLine()); game.place(number, row, col); } // If the square is not empty, we assume the user wants to remove its contents. else { game.remove(row, col); } /********** The code you will need to modify ends here *********/ // Display the resulting game board display(game); } System.out.println("You win!"); } /** * Support method to display the game in 3x3 form * @param game SudokuGame object */ public static void display(SudokuGame game) { System.out.println("Current board:"); System.out.println("+------+"); for (int row = 0; row < 3; row++) { System.out.print("|"); for (int col = 0; col < 3; col++) { System.out.print(game.getValue(row, col)+" "); } System.out.println("|"); } System.out.println("+------+"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
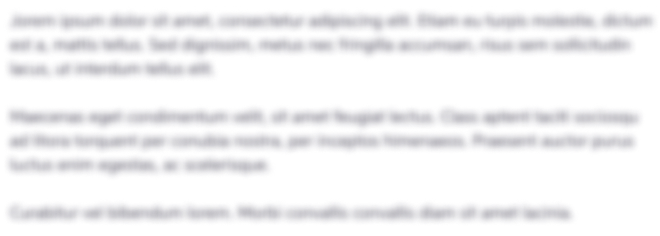
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started