Question
Exception Handling - Java Add scanner for user input for the objects and implement exception handling for them. Implement the following program with exception handling.
Exception Handling - Java
Add scanner for user input for the objects and implement exception handling for them.
Implement the following program with exception handling.
------------------------------Circle.java------------------------------------------
public class Circle extends Shape { //Extension of new subclass private long centerX; //Private variable private long centerY; //Private variable private double radius; //Private variable public Circle(long x, long y, double r) //Constructor { this.centerX = x; //Initializing this.centerY = y; //Initializing this.radius = r; //Initializing calcArea(); //Initializing } @Override public void calcArea() //Override function to share the function to the extended class { this.area = (3.14519 * this.radius * this.radius); //Area of a circle by multiplying pi by radius^2 } @Override public String toString() //Override function to share the function to the extended class { return "The Area of the Circle is: " + this.getArea() + " Radius: " + this.radius + " X-Coordinate: " + this.centerX + " Y-Coordinate: " + this.centerY; } //Printing of information for a circle public void setCenterX(long centerX) //Set method { this.centerX = centerX; } public void setCenterY(long centerY) //Set method { this.centerY = centerY; } public void setRadius(double radius) //Set Method { this.radius = radius; } public long getCenterX() //Get method { return centerX; } public long getCenterY() //Get method { return centerY; } public double getRadius() //Get method { return radius; }
}
---------------------------------------Rectangle.java----------------------------------------
public class Rectangle extends Shape { //Abstract class with extension private long width; //PRivate variable private long length; //Private variable public Rectangle(long width, long length) //Constructor { this.width = width; //Initializing this.length = length; //Initializing calcArea(); //Initializing } public void setWidth(long width) //Set method { this.width = width; } public void setLength(long length) //Set method { this.length = length; } public long getWidth() //Get method { return width; } public long getLength() //Get method { return length; } @Override public void calcArea() //Override function to use the function in a subclass { this.area = (this.length * this.width); //Finding the area length * width } @Override public String toString() //Override function to use the function in a subclass { return "Area of Rectangle is: " + this.getArea() + " Length: " + this.length + " Width: " + this.width; }
}
-------------------------------Shape.java------------------------------------------------------------
public abstract class Shape { //Abstract class that will be extended to the other subclasses protected double area; //Protected public double getArea; //Public public String toString; //Public public abstract void calcArea(); //Abstract method used in all classes public double getArea() //Returns the value { return this.area; } public String toString() //Reports the shape area { calcArea();//Abstract method return "The Area is " + this.area; } }
-----------------------------------------ShapeTest.java-----------------------------------------------------------
import java.util.ArrayList; //Importing of Array List
public class ShapeTest { //Tester File
public static void main(String[] args) { ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
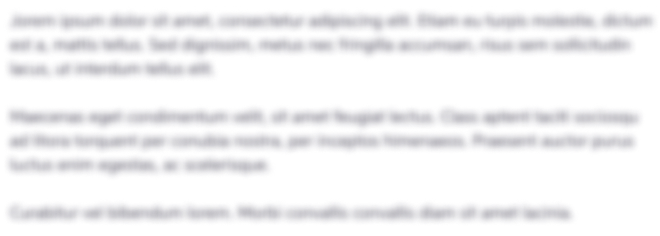
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started