Question
Exception Handling - Java Implement the following program with exception handling. --------------------------HealthProfile.java----------------------------------------------------- import java.util.Calendar; //Calendar used to calculate age public class HealthProfile { private String
Exception Handling - Java
Implement the following program with exception handling.
--------------------------HealthProfile.java-----------------------------------------------------
import java.util.Calendar; //Calendar used to calculate age
public class HealthProfile { private String firstName; //Instance Variable private String lastName; //Instance Variable private String gender; //Instance Variable private int month; //Instance Variable private int day; //Instance Variable private int year; //Instance Variable private int height; //Instance Variable private int weight; //Instance Variable
public HealthProfile(String firstName, String lastName, String gender, int month, int day, int year, int height, int weight) { //Constructor this.firstName = firstName; //Initialization of variables this.lastName = lastName; this.gender = gender; this.month = month; this.day = day; this.year = year; this.height = height; this.weight = weight; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getFirstName() { return firstName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getLastName() { return lastName; } public void setGender(String gender) { this.gender = gender; } public String getGender() { return gender; } public void setMonth(int month) { this.month = month; } public int getMonth() { return month; } public void setDay(int day) { this.day = day; } public int getDay() { return day; } public void setYear(int year) { this.year = year; } public int getYear() { return year; } public void setHeight(int height) { this.height = height; } public int getHeight() { return height; } public void setWeight(int weight) { this.weight = weight; } public int getWeight() { return weight; } public int getAge() //Get age function using the calendar to calculate age of patient { Calendar dOB = Calendar.getInstance(); dOB.set(year, month, day); Calendar now = Calendar.getInstance(); //Using the calendar to find the correct date return (now.get(Calendar.YEAR) - dOB.get(Calendar.YEAR)) - 1; //Equation to use the current year - the year patient was born } public int maximumHeartRate() //Function to find the maximum heart rate possible for patient { return 220 - getAge(); //Taking the standard 220 - the patients age } public String targetHeartRate() //Function taking the maximum heart rate and finding the range { int maxHR = maximumHeartRate(); return Double.toString(maxHR * 0.5) + "-" + Double.toString(maxHR * 0.85) + "BPM"; } public double calcBMI() //Function to calculate BMI { return(weight * 703) / (height * height); //Using the equartion provided we implimented the height and weight recieved from the patient } public String valueBMI() //Function to take the BMI from before and compare it to the chart on values { double bmi = calcBMI(); //Using If and else if statements to find the correct value for the patients BMI if(bmi < 18.5) { return "Underweight"; } else if(bmi > 18.5 && bmi < 24.9) { return "Normal"; } else if(bmi > 25 && bmi < 29.9) { return "Overweight"; } else if(bmi >= 30) { return "Obese"; } return "Error, please try again.";
}
}
--------------------------------HealthProfileTest.java-----------------------------------------------
import java.util.Scanner; //Scanner used to receive data from patient
public class HealthProfileTest {
/** * @param args the command line arguments */ public static void main(String[] args) { //Main function Scanner scanner = new Scanner(System.in); System.out.println("Enter Patient's First Name: "); //Collecting first name String fname = scanner.nextLine(); System.out.println("Enter Patient's Last Name: "); //Collecting last name String lname = scanner.nextLine(); System.out.println("Enter Gender Male or Female: "); //Collecting gender String gender = scanner.nextLine(); System.out.println("Enter month of Birth: "); //Collecting month of birth int month = scanner.nextInt(); System.out.println("Enter the Day of Birth: "); //Collecting day of birth int day = scanner.nextInt(); System.out.println("Enter the Year of Birth: "); //Collecting year of birth int year = scanner.nextInt(); System.out.println("Enter Weight in lbs: "); //Collecting weight int weight = scanner.nextInt(); System.out.println("Enter Height in Inches"); //Collecting height int height = scanner.nextInt(); HealthProfile patientHealthInfo = new HealthProfile(fname, lname, gender, month, day, year, height, weight); //New obect produced System.out.println("Name: "+ patientHealthInfo.getFirstName().concat(" "+ patientHealthInfo.getLastName())); System.out.println("Age of Patient: " + patientHealthInfo.getAge()); System.out.println("Gender of Patient: "+ patientHealthInfo.getGender()); System.out.println("Birthdate: "+ patientHealthInfo.getMonth()+ "/" + patientHealthInfo.getDay()+ "/" + patientHealthInfo.getYear()); System.out.println("Weight of the Patient in lbs: "+ patientHealthInfo.getWeight()); System.out.println("Height of Patient in Inches: "+ patientHealthInfo.getHeight()); System.out.println("Maximum Heart Rate of Patient: " + patientHealthInfo.maximumHeartRate()); System.out.println("Target Heart Rate for Patient: "+ patientHealthInfo.targetHeartRate()); //Printing of data double bmi = patientHealthInfo.calcBMI(); System.out.println("BMI of Patient: "+ bmi); System.out.println("BMI of Patient puts them at: " + patientHealthInfo.valueBMI()); System.out.println(); System.out.println("BMI VALUES CHART"); System.out.println("UNDERWEIGHT = LESS THAN 18.5"); System.out.println("NORMAL = BETWEEN 18.5 & 24.9"); System.out.println("OVERWEIGHT = BETWEEN 25 & 29.9"); System.out.println("OBESE = 30 & OVER"); //Printing of BMI values chart for patient to interpret what their value means } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
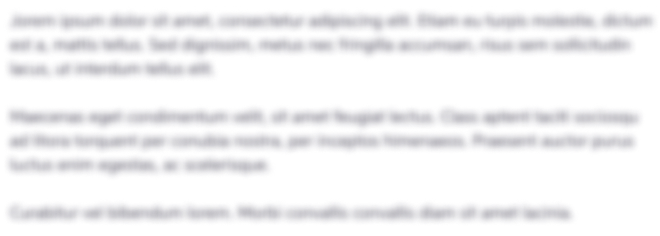
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started