Question
Exercise 1 (50 points) Divide Search Consider the algorithm binarySearch(A, p, r, x): the description of this algorithm is provided below. Inputs: a sorted array
Exercise 1 (50 points) Divide Search
Consider the algorithm binarySearch(A, p, r, x): the description of this algorithm is provided below.
Inputs:
- a sorted array A
- index p of the first element
- index r of last element
- an element x to search
Output:
- index of element x in Sequence A if x exists in A
- -1 if x does not exist in Sequence A.
Algorithm description
int binarySearch(A, p, r, x)
if (r >= p)
midpoint = p + (r-p)/2;
if A[midpoint] == x
return midpoint;
if A[midpoint] > x
return binarySearch(A, p, midpoint-1, x);
else
return binarySearch(A, midpoint+1, r, x);
return -1;
The objective of this exercise is to derive the time complexity (running) time of the divide search. (If interested, you could read about divide search on Wikipedia)
- (6 points) Let A = (-5, 7, 9, 15, 20, 30, 33, 37, 41, 55, 63, 65, 78, 102). Assume that the index of the first element is 1.
- Execute manually binarySearch(A,1, A.length, 65). What is the output?
- Execute manually binarySearch(A,1, A.length, 19). What is the output?
- Execute manually binarySearch(A,1, A.length, 102). What is the output?
- Execute manually binarySearch(A,1, A.length, -5). What is the output?
- (2 points) Which operation should you count to determine the running time T(n) of the divide search in a sequence A of length n?
- (12 points) Let us count the comparisons ((if A[midpoint] == x) and (if A[midpoint] > x)). Express the running time T(n) as a recurrence relation.
- (12 points) Solve the recurrence relation T(n) using the recursion-tree method
- (8 points) Solve the recurrence relation T(n) using the substitution method
- (10 points) Solve the recurrence relation T(n) using the master method
I just need Questions 4, 5, and 6 answered
Step by Step Solution
There are 3 Steps involved in it
Step: 1
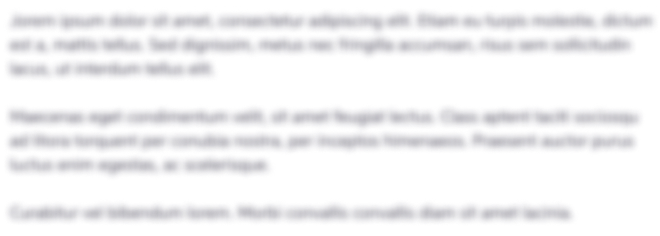
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started