Question
Exercise 1: You are tasked with writing a Item class for a store The class Item has the following data members: -IName: a string to
Exercise 1:
You are tasked with writing a Item class for a store
The class Item has the following data members:
-IName: a string to store the item name.
-IPrice: an real to store the item price
-ISpoil: a character to store if the item is perishable.
-IBreak: a Boolean to store true if the item is breakable or false otherwise.
-Additionally, you need to define:
1- a default constructor that sets the value of the various data members to: Egg for the name,3.00 for the price, S for the ISpoil and true for IBreak.
2- a parameterized constructor that initialize these data members with values provided.
3- the setter and getter functions to set and get all of the above data members.
4- a function member printItemPrice() that prints the price of the item
4- a function member isCheaper(Item I) that compares the price of a current item with a Item I (passed as an argument) and returns the name of the current item if it is cheaper than I or the name of the Item I otherwise.
Write your class definition below:
//Item.h
class Item {//write your code below
private:
public:
}
-in the Item.cpp file below, write the implementation of all the functions members defined above with the required library and header files
// Item.cpp
3- Write your function main() in the file Driver.cpp in which you define 2 objects of class Item: I1 initialized with the default constructor and I2 initialized with the following data (Candy, 1.99, N, false)
-Call the function member printItemPrice to display the price of I2.
-Call the function member isCheaper and use the getter getName() to print the name of the cheaper item between I1 and I2
//Driver.cpp
//Enter your code here
Exercise 2:
Write a C++ program that finds the sum of all values stored in an integer array A with n elements. The number of elements in the array (size of the array) is not known in advance, so the user is prompted to enter the number of elements and initialize the array. (Use dynamic memory allocation )
-Find the Big O function O(?) that describes the performance (running time) of the program you wrote above.
Exercise 3:
Consider the function f(n)= 3n3+ 5n2 + 2n.
Find a constant C1 (worst time) such that 3n3+ 5n2 + 2n C1.Q (n3)
Find a constant C2(best time) such that 3n3+ 5n2 + 2n C2. F(n3)
Growth of Algorithms: O(1) < O(log n) < O(n)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
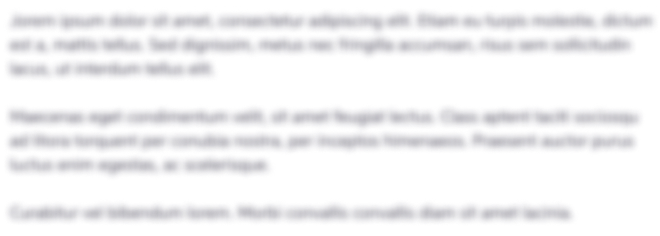
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started