Question
Exercise 7-1 Add exception handling to the simple calculator In this exercise, youll add exception handling to the Simple Calculator form of exercise 6-1. 1.
Exercise 7-1 Add exception handling to the simple calculator
In this exercise, youll add exception handling to the Simple Calculator form of exercise 6-1.
1. Open the SimpleCalculator project in the Assignment Exercises\Chapter 07\SimpleCalculator With Exception Handling directory.
2. Add a TryCatch statement to the btnCalculate_Click event handler that will catch any exceptions that occur when the statements in that event handler are executed. If an exception occurs, display a dialog box with the error message, the type of error, and a stack trace. Test the application by entering a nonnumeric value for one of the operands.
3. Add three additional catch blocks to the TryCatch statement that will catch an InvalidCastException, an OverflowException, and a DivideByZeroException. (If you used the ToDecimal method of the Convert class instead of the CDec function to convert the operands entered by the user to decimals, you should catch a FormatException instead of an InvalidCastException.) These catch blocks should display a dialog box with an appropriate error message.
4. Test the application again by entering a nonnumeric value for one of the operands. Then, enter 0 for the second operand as shown above to see what happens.
Here is my current Visual Basic code:
Public Class Form1 ' Calculate button click event handler Private Sub btnCalculate_Click(sender As Object, e As EventArgs) Handles btnCalculate.Click
' Reading data from text boxes Dim operand1 = Convert.ToDecimal(tbOperand1.Text) Dim operand2 = Convert.ToDecimal(tbOperand2.Text)
' Reading operator Dim operator1 = tbOperator.Text
Dim result As Decimal
' Calculating result result = calculate(operand1, operand2, operator1)
' Writing result tbResult.Text = Decimal.Round(result, 4).ToString()
End Sub
' Function that calculates the appropriate operation based on user input Private Function calculate(ByVal operand1 As Decimal, ByVal operand2 As Decimal, ByVal operator1 As String) As Decimal
Dim result As Decimal
' Executing corresponding statements Select Case operator1
' Add Case "+" result = operand1 + operand2
' Subtraction Case "-" result = operand1 - operand2
' Multiplication Case "*" result = operand1 * operand2
' Divison Case "/" result = operand1 / operand2
' Any other operator Case Else result = 0 End Select
' Returning result Return result
End Function ' Exit button click event handler Private Sub btnExit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnExit.Click
' Exiting Application Application.Exit()
End Sub
Private Sub Form1_KeyDown(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyEventArgs) Handles MyBase.KeyDown
' If Escape key is Pressed If e.KeyCode = Keys.Escape Then btnExit_Click(sender, e) End If
' If enter key is pressed If e.KeyCode = Keys.Enter Then btnCalculate_Click(sender, e) End If
End Sub End Class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
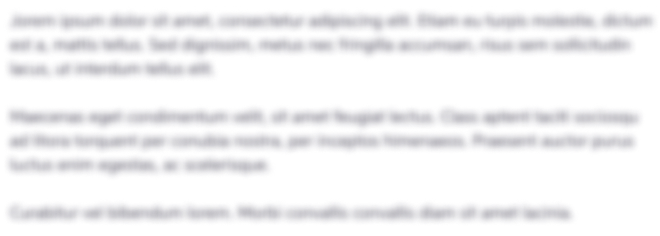
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started