Question
expenselist.java: //ExpenseList class //Defines an unordered list of Expense objects //Uses the generic unordered list (List ) class and Expense class public class ExpenseList {
expenselist.java:
//ExpenseList class
//Defines an unordered list of Expense objects
//Uses the generic unordered list (List
public class ExpenseList
{
private List
public ExpenseList()
{
expenses = new List
}
public void add(Expense exp)
{
expenses.add(exp);
}
public boolean isEmpty()
{
return expenses.isEmpty();
}
public boolean contains(Expense exp)
{
return expenses.contains(exp);
}
public Expense first()
{
return expenses.first();
}
public Expense next()
{
return expenses.next();
}
public void enumerate()
{
expenses.enumerate();
}
public double maxExpense()
{
double max =0.0, amt;
Expense exp = expenses.first();
while (exp!=null)
{
amt = exp.getAmount();
if (amt>max)
max = amt;
exp = expenses.next();
}
return max;
}
public double minExpense()
{
double min =Double.MAX_VALUE, amt;
Expense exp = expenses.first();
if (exp==null) return 0.0;
else
{
while (exp!=null)
{
amt = exp.getAmount();
if (amt min = amt; exp = expenses.next(); } } return min; } /* public double avgExpense() { //TODO: complete this method //Finds the average of all expenses } public double totalExpense() { //TODO: complete this method //Finds the sum of all expenses } public double amountSpentOn(String expItem) { //TODO: complete this method //Finds the amount spent on a particular item } */ } expenselistdemo.java: //ExpenseListDemo //Program reads a text file containing list of expenses //File contains date, description and amount one on each line: //Sep 21, 2018 //Lunch //4.51 //Program then processes the file and displays expense data such as //max expense amount, min expense amount, etc. import java.util.Scanner; import java.io.*; public class ExpenseListDemo { public static void main(String[] args) throws IOException { Scanner keyboard = new Scanner(System.in); System.out.print("Enter the filename to read from: "); String filename = keyboard.nextLine(); File file = new File(filename); Scanner inputFile = new Scanner(file); ExpenseList sepexpenses = new ExpenseList(); String date, desc, cost; Expense exp=null; while (inputFile.hasNext()) { date = inputFile.nextLine(); desc = inputFile.nextLine(); cost = inputFile.nextLine(); exp = new Expense(date, desc, Double.parseDouble(cost)); sepexpenses.add(exp); } inputFile.close(); System.out.println("September Expenses"); System.out.println("Max expense: $" + sepexpenses.maxExpense()); System.out.println("Min expense: $" + sepexpenses.minExpense()); //System.out.println("Average expense: $" + sepexpenses.avgExpense()); //System.out.println("The amount spent on groceries: $" + sepexpenses.amountSpentOn("Groceries")); //System.out.println("Total expense: $" + sepexpenses.totalExpense()); } } sepexpenses.txt: Sep 21, 2018 Lunch 4.51 Sep 21, 2018 Gas 25.00 Sep 21, 2018 Movies 36.36 Sep 21, 2018 Groceries 22.55 Sep 24, 2018 Groceries 72.58 Sep 24, 2018 Breakfast 11.48 Sep 24, 2018 Tennis 15.00 Sep 24, 2018 Hair Salon 34.50 Sep 25, 2018 CreditCard 245.00 Sep 27, 2018 CarRental 360.01 Sep 27, 2018 Pizza 11.50 Sep 28, 2018 Gas 81.1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
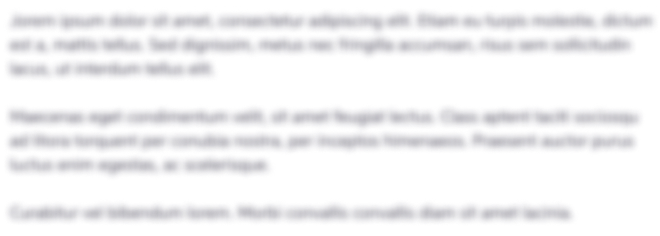
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started