Question
Explain to him the work of running this code, and explain each line of the code with comments #include using namespace std; struct Node {
Explain to him the work of running this code, and explain each line of the code with comments
#include
using namespace std;
struct Node {
int data;
Node* next;
};
class Stack {
private:
Node* top;
public:
Stack() { top = NULL; }
void push(int data) {
Node* newNode = new Node;
newNode->data = data;
newNode->next = top;
top = newNode;
}
int pop() {
if (isEmpty()) {
cout << "Error: Stack is empty" << endl;
return -1;
}
int data = top->data;
Node* temp = top;
top = top->next;
delete temp;
return data;
}
int getTop() {
if (isEmpty()) {
cout << "Error: Stack is empty" << endl;
return -1;
}
return top->data;
}
void display() {
if (isEmpty()) {
cout << "Error: Stack is empty" << endl;
return;
}
Node* temp = top;
while (temp != NULL) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
bool isEmpty() {
return top == NULL;
}
};
//check the balancing of the braces
bool checkBraceBalancing(const string& str) {
Stack stack;
for (char c : str) {
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
} else if (c == ')' || c == ']' || c == '}') {
if (stack.isEmpty()) {
return false;
}
char top = stack.getTop();
if ((c == ')' && top != '(') ||
(c == ']' && top != '[') ||
(c == '}' && top != '{')) {
return false;
}
stack.pop();
}
}
return stack.isEmpty();
}
//check whether palindrome or not
bool isPalindrome(string word) {
Stack stack;
for (char c : word) {
stack.push(c);
}
for (int i = 0; i < word.length(); i++) {
if (word[i] != stack.getTop()) {
return false;
}
stack.pop();
}
return true;
}
//Giving precedence to the operators
int precedence(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
if (op == '^') return 3;
return 0;
}
//Convert an infix expression to its postfix expression
string infixToPostfix(const string& infix) {
string postfix;
Stack stack;
for (char c : infix) {
if (isalnum(c)) {
postfix += c;
} else if (c == '(') {
stack.push(c);
} else if (c == ')') {
while (stack.getTop() != '(') {
postfix += stack.getTop();
stack.pop();
}
stack.pop();
} else {
while (!stack.isEmpty() && precedence(c) <= precedence(stack.getTop())) {
postfix += stack.getTop();
stack.pop();
}
stack.push(c);
}
}
while (!stack.isEmpty()) {
postfix += stack.getTop();
stack.pop();
}
return postfix;
}
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/' || c == '^');
}
//List the expression operators based on their precedence
void listOperatorsByPrecedence(const string& infix) {
Stack stack;
for (char c : infix) {
if (isOperator(c)) {
while (!stack.isEmpty() && isOperator(stack.getTop()) && precedence(c) <= precedence(stack.getTop())) {
cout << stack.getTop() << " ";
stack.pop();
}
stack.push(c);
}
}
while (!stack.isEmpty()) {
cout << stack.getTop() << " ";
stack.pop();
}
}
double performOperation(char op, double operand1, double operand2) {
switch (op) {
case '+': return operand1 + operand2;
case '-': return operand1 - operand2;
case '*': return operand1 * operand2;
case '/': return operand1 / operand2;
case '^': return pow(operand1, operand2);
}
return 0;
}
//Evaluate a postfix expression
double evaluatePostfix(const string& postfix) {
Stack stack;
for (char c : postfix) {
if (isdigit(c)) {
stack.push(c - '0');
} else {
double operand2 = stack.pop();
double operand1 = stack.pop();
double result = performOperation(c, operand1, operand2);
stack.push(result);
}
}
return stack.pop();
}
int main() {
while(1){
int choice, flag=0;
cout<<"Enter 1 for Check the balancing of the braces ";
cout<<"Enter 2 for Check whether the word is a palindrome or not ";
cout<<"Enter 3 for Convert an infix expression to its postfix expression ";
cout<<"Enter 4 for List the expression operators based on their precedence ";
cout<<"Enter 5 for Evaluate a postfix expression ";
cout<<"Enter 6 for Exit ";
cin>>choice;
switch(choice){
case 1: {
string input;
cout<<"Enter a braces expression: ";
cin>>input;
if (checkBraceBalancing(input))
cout << "Braces are balanced." << endl;
else
cout << "Braces are not balanced." << endl;
break;
}
case 2: {
string word;
cout<<"Enter a string: ";
cin>>word;
if (isPalindrome(word))
cout << word << " is a palindrome" << endl;
else
cout << word << " is not a palindrome" << endl;
break;
}
case 3: {
string infixExpression;
cout << "Enter an Infix expression: ";
cin>>infixExpression;
string postfixExpression = infixToPostfix(infixExpression);
cout << "Postfix expression: " << postfixExpression << endl;
break;
}
case 4: {
string infixExpression;
cout << "Enter an Infix expression: ";
cin>> infixExpression;
cout << " Operators by precedence: ";
listOperatorsByPrecedence(infixExpression);
break;
}
case 5: {
string postfixExpression;;
cout<<"Enter the Postfix Expression"< cin>>postfixExpression; double result = evaluatePostfix(postfixExpression); cout << "Result: " << result << endl; break; } case 6: flag=1; break; default: cout<<" Enter a valid choice"; break; } if(flag==1) break; cout<<" "; } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
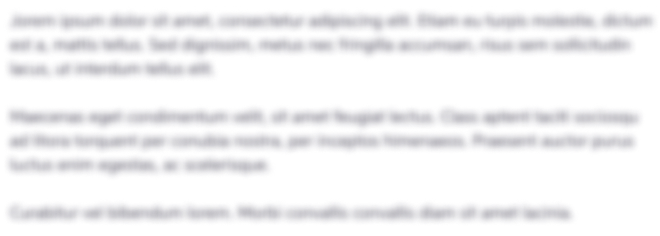
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started