Question
Extend the buildParseTree function to handle mathematical expressions that do not have spaces between every character. Extend the buildParseTree function to handle mathematical expressions that
Extend the buildParseTree function to handle mathematical expressions that do not have spaces between every character. Extend the buildParseTree function to handle mathematical expressions that do not have spaces between every character.
class Stack: def __init__(self): self.items = []
def isEmpty(self): return self.items == []
def push(self, item): self.items.append(item)
def pop(self): return self.items.pop()
def peek(self): return self.items[len(self.items)-1]
def size(self): return len(self.items)
class BinaryTree: """ A recursive implementation of Binary Tree Using links and Nodes approach. """ def __init__(self,rootObj): self.key = rootObj self.leftChild = None self.rightChild = None
def insertLeft(self,newNode): if self.leftChild == None: self.leftChild = BinaryTree(newNode) else: t = BinaryTree(newNode) t.left = self.leftChild self.leftChild = t def insertRight(self,newNode): if self.rightChild == None: self.rightChild = BinaryTree(newNode) else: t = BinaryTree(newNode) t.right = self.rightChild self.rightChild = t
def isLeaf(self): return ((not self.leftChild) and (not self.rightChild))
def getRightChild(self): return self.rightChild
def getLeftChild(self): return self.leftChild
def setRootVal(self,obj): self.key = obj
def getRootVal(self,): return self.key
def inorder(self): if self.leftChild: self.leftChild.inorder() print(self.key) if self.rightChild: self.rightChild.inorder()
def postorder(self): if self.leftChild: self.leftChild.postorder() if self.rightChild: self.rightChild.postorder() print(self.key)
def preorder(self): print(self.key) if self.leftChild: self.leftChild.preorder() if self.rightChild: self.rightChild.preorder()
def printexp(self): if self.leftChild: print('(', end=' ') self.leftChild.printexp() print(self.key, end=' ') if self.rightChild: self.rightChild.printexp() print(')', end=' ')
def postordereval(self): opers = {'+':operator.add, '-':operator.sub, '*':operator.mul, '/':operator.truediv} res1 = None res2 = None if self.leftChild: res1 = self.leftChild.postordereval() #// \label{peleft} if self.rightChild: res2 = self.rightChild.postordereval() #// \label{peright} if res1 and res2: return opers[self.key](res1,res2) #// \label{peeval} else: return self.key
def inorder(tree): if tree != None: inorder(tree.getLeftChild()) print(tree.getRootVal()) inorder(tree.getRightChild())
def printexp(tree): if tree.leftChild: print('(', end=' ') printexp(tree.getLeftChild()) print(tree.getRootVal(), end=' ') if tree.rightChild: printexp(tree.getRightChild()) print(')', end=' ')
def printexp(tree): sVal = "" if tree: sVal = '(' + printexp(tree.getLeftChild()) sVal = sVal + str(tree.getRootVal()) sVal = sVal + printexp(tree.getRightChild()) + ')' return sVal
def postordereval(tree): opers = {'+':operator.add, '-':operator.sub, '*':operator.mul, '/':operator.truediv} res1 = None res2 = None if tree: res1 = postordereval(tree.getLeftChild()) #// \label{peleft} res2 = postordereval(tree.getRightChild()) #// \label{peright} if res1 and res2: return opers[tree.getRootVal()](res1,res2) #// \label{peeval} else: return tree.getRootVal()
def height(tree): if tree == None: return -1 else: return 1 + max(height(tree.leftChild),height(tree.rightChild))
def buildParseTree(fpexp): fplist = fpexp.split() pStack = Stack() eTree = BinaryTree('') pStack.push(eTree) currentTree = eTree for i in fplist: if i == '(': currentTree.insertLeft('') pStack.push(currentTree) currentTree = currentTree.getLeftChild() elif i not in ['+', '-', '*', '/', ')']: currentTree.setRootVal(int(i)) parent = pStack.pop() currentTree = parent elif i in ['+', '-', '*', '/']: currentTree.setRootVal(i) currentTree.insertRight('') pStack.push(currentTree) currentTree = currentTree.getRightChild() elif i == ')': currentTree = pStack.pop() else: raise ValueError return eTree
pt = buildParseTree("( ( 10 + 5 ) * 3 )") pt.postorder()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
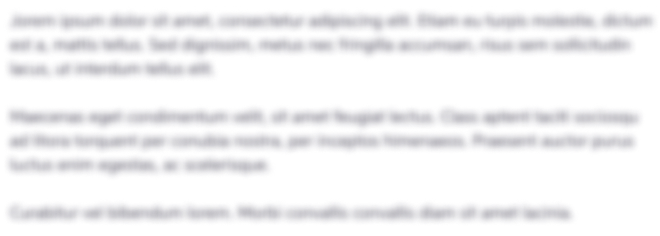
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started