Question
/** * fifteen.c * * Implements Game of Fifteen (generalized to d x d). * * Usage: fifteen d * * whereby the board's dimensions
/** | ||||||
* fifteen.c | ||||||
* | ||||||
* Implements Game of Fifteen (generalized to d x d). | ||||||
* | ||||||
* Usage: fifteen d | ||||||
* | ||||||
* whereby the board's dimensions are to be d x d, | ||||||
* where d must be in [DIM_MIN,DIM_MAX] | ||||||
* | ||||||
* Note that usleep is obsolete, but it offers more granularity than | ||||||
* sleep and is simpler to use than nanosleep; `man usleep` for more. | ||||||
*/ | ||||||
#define _XOPEN_SOURCE 500 | ||||||
#include | ||||||
#include | ||||||
#include | ||||||
#include | ||||||
// constants | ||||||
#define DIM_MIN 3 | ||||||
#define DIM_MAX 9 | ||||||
// board | ||||||
int board[DIM_MAX][DIM_MAX]; | ||||||
// dimensions | ||||||
int d; | ||||||
// prototypes | ||||||
void clear(void); | ||||||
void greet(void); | ||||||
void init(void); | ||||||
void draw(void); | ||||||
bool move(int tile); | ||||||
bool won(void); | ||||||
int main(int argc, string argv[]) | ||||||
{ | ||||||
// ensure proper usage | ||||||
if (argc != 2) | ||||||
{ | ||||||
printf("Usage: fifteen d "); | ||||||
return 1; | ||||||
} | ||||||
// ensure valid dimensions | ||||||
d = atoi(argv[1]); | ||||||
if (d < DIM_MIN || d > DIM_MAX) | ||||||
{ | ||||||
printf("Board must be between %i x %i and %i x %i, inclusive. ", | ||||||
DIM_MIN, DIM_MIN, DIM_MAX, DIM_MAX); | ||||||
return 2; | ||||||
} | ||||||
// open log | ||||||
FILE *file = fopen("log.txt", "w"); | ||||||
if (file == NULL) | ||||||
{ | ||||||
return 3; | ||||||
} | ||||||
// greet user with instructions | ||||||
greet(); | ||||||
// initialize the board | ||||||
init(); | ||||||
// accept moves until game is won | ||||||
while (true) | ||||||
{ | ||||||
// clear the screen | ||||||
clear(); | ||||||
// draw the current state of the board | ||||||
draw(); | ||||||
// log the current state of the board (for testing) | ||||||
for (int i = 0; i < d; i++) | ||||||
{ | ||||||
for (int j = 0; j < d; j++) | ||||||
{ | ||||||
fprintf(file, "%i", board[i][j]); | ||||||
if (j < d - 1) | ||||||
{ | ||||||
fprintf(file, "|"); | ||||||
} | ||||||
} | ||||||
fprintf(file, " "); | ||||||
} | ||||||
fflush(file); | ||||||
// check for win | ||||||
if (won()) | ||||||
{ | ||||||
printf("ftw! "); | ||||||
break; | ||||||
} | ||||||
// prompt for move | ||||||
printf("Tile to move: "); | ||||||
int tile = get_int(); | ||||||
// quit if user inputs 0 (for testing) | ||||||
if (tile == 0) | ||||||
{ | ||||||
break; | ||||||
} | ||||||
// log move (for testing) | ||||||
fprintf(file, "%i ", tile); | ||||||
fflush(file); | ||||||
// move if possible, else report illegality | ||||||
if (!move(tile)) | ||||||
{ | ||||||
printf(" Illegal move. "); | ||||||
usleep(500000); | ||||||
} | ||||||
// sleep thread for animation's sake | ||||||
usleep(500000); | ||||||
} | ||||||
// close log | ||||||
fclose(file); | ||||||
// success | ||||||
return 0; | ||||||
} | ||||||
/** | ||||||
* Clears screen using ANSI escape sequences. | ||||||
*/ | ||||||
void clear(void) | ||||||
{ | ||||||
printf("\033[2J"); | ||||||
printf("\033[%d;%dH", 0, 0); | ||||||
} | ||||||
/** | ||||||
* Greets player. | ||||||
*/ | ||||||
void greet(void) | ||||||
{ | ||||||
clear(); | ||||||
printf("WELCOME TO GAME OF FIFTEEN "); | ||||||
usleep(2000000); | ||||||
} | ||||||
/** | ||||||
* Initializes the game's board with tiles numbered 1 through d*d - 1 | ||||||
* (i.e., fills 2D array with values but does not actually print them). | ||||||
*/ | ||||||
void init(void) | ||||||
{ | ||||||
g | ||||||
} | ||||||
/** | ||||||
* Prints the board in its current state. | ||||||
*/ | ||||||
void draw(void) | ||||||
{ | ||||||
// TODO | ||||||
} | ||||||
/** | ||||||
* If tile borders empty space, moves tile and returns true, else | ||||||
* returns false. | ||||||
*/ | ||||||
bool move(int tile) | ||||||
{ | ||||||
// TODO | ||||||
return false; | ||||||
} | ||||||
/** | ||||||
* Returns true if game is won (i.e., board is in winning configuration), | ||||||
* else false. | ||||||
*/ | ||||||
bool won(void) | ||||||
{ | ||||||
// TODO | ||||||
return false; | ||||||
} | ||||||
0. TODO 1. TODO 2. TODO 3. TODO Read over the code and comments in fifteen.c and then answer the questions below in questions.txt, which is a (nearly empty) text file that we included for you inside of the distributions fifteen directory. No worries if youre not quite sure how fprintf or fflush work; were simply using those to automate some testing. Besides 4 4 (which are Game of Fifteens dimensions), what other dimensions does the framework allow? With what sort of data structure is the games board represented? What function is called to greet the player at games start? What functions do you apparently need to implement? mplement the Game of Fifteen, per the comments in fifteen.c. Implement init. Implement draw. Implement move. Implement won. | ||||||
Step by Step Solution
There are 3 Steps involved in it
Step: 1
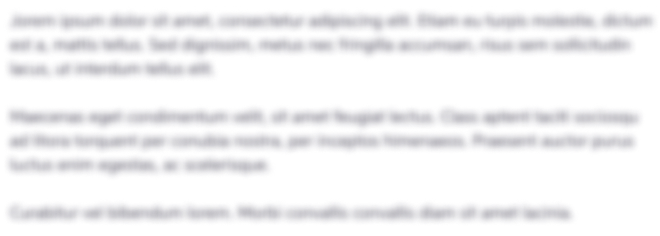
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started