Question
file exp_eval.py outline is given at the bottom with starting code for function infix_to_postfix postfix_eval function has already been written please contain docstrings explaining purposes
file exp_eval.py outline is given at the bottom with starting code for function infix_to_postfix
postfix_eval function has already been written
please contain docstrings explaining purposes
CODE IS IN PYTHON
#exp_eval.py
from __future__ import annotations
from array_stack import ArrayStack, empty_stack, push, pop, peek, is_empty, size
# NOTE: You'll probably need to import some things from array_stack.
def postfix_eval(input_string: str) -> float:
"""Evaluate the given RPN expression.
Args:
input_string: an RPN expression
Returns:
The result of the expression evaluation
Raises:
ValueError: if the input is not well-formed
ZeroDivisionError: if the input would cause division by zero
"""
# Split the input string into tokens
tokens = input_string.split()
# Initialize an empty stack
stack = empty_stack()
# Process the expression from left to right
for token in tokens:
if token.isdigit():
# If the token is a number, push it onto the stack
push(stack, float(token))
elif token in "+-*/":
# If the token is an operator, pop the required number of values from the stack
try:
op2 = pop(stack)
op1 = pop(stack)
except IndexError:
raise ValueError("insufficient operands")
# Perform the operation
if token == "+":
result = op1 + op2
elif token == "-":
result = op1 - op2
elif token == "*":
result = op1 * op2
elif token == "/":
if op2 == 0:
raise ZeroDivisionError("division by zero")
result = op1 / op2
# Push the result back onto the stack
push(stack, result)
else:
# If the token is neither a valid operand nor a valid operator
raise ValueError("invalid token")
# Return the last value remaining on the stack
if size(stack) != 1:
raise ValueError("too many operands")
return pop(stack)
def infix_to_postfix(input_string: str) -> str: """Convert the given infix string to RPN.
Args: input_string: an infix expression
Returns: The equivalent expression in RPN """
array_stacks.py is also provided incase you need anything from there
#array_stack.py
from __future__ import annotations
from typing import Any
class ArrayStack:
"""A class that implements an array-based stack
The stack is initialized with a default capacity of 4, which means the stack can hold up to 4 elements
If the number of elements in the stack exceeds the capacity, the capacity is doubled
Attributes:
capacity: The maximun number of elements the stack can hold.
array: The list to store the elements in the stack.
size: The number of elements in the stack.
"""
def __init__(self) -> None:
self.capacity = 4
self.array: list[Any] = [None] * self.capacity
self.size = 0
def empty_stack() -> ArrayStack:
return ArrayStack()
def push(stack: ArrayStack, value: Any) -> None:
if stack.size == stack.capacity:
stack.capacity *= 2
new_array = [None] * stack.capacity
for i in range(stack.size):
new_array[i] = stack.array[i]
stack.array = new_array
stack.array[stack.size] = value
stack.size += 1
def pop(stack: ArrayStack) -> Any:
if stack.size == 0:
raise IndexError("pop from an empty stack")
value = stack.array[stack.size - 1]
stack.array[stack.size - 1] = None
stack.size -= 1
return value
def peek(stack: ArrayStack) -> Any:
if stack.size == 0:
raise IndexError("peek from an empty stack")
return stack.array[stack.size - 1]
def is_empty(stack: ArrayStack) -> bool:
return stack.size == 0
def size(stack: ArrayStack) -> int:
return stack.size
4 Converting Infix Expressions to Postfix In a file called exp_eval.py, you will implement this algorithm as a function called infix_to_postfix. We can (and you will!) also use a stack to convert an infix expression to an RPN expression via the Shunting-yard algorithm. The steps are: - Process the expression from left to right. - When you encounter a value: - Append the value to the RPN expression - When you encounter an opening parenthesis: - Push it onto the stack - When you encounter a closing parenthesis: - Until the top of the stack is an opening parenthesis, pop operators off the stack and append them to the RPN expression - Pop the opening parenthesis from the stack (but don't put it into the RPN expression) - When you encounter an operator, o1 : - While there is an operator, o2 on the top of the stack and either: * o2 has greater precedence than o1, or * they have the same precedence and o1 is left-associative Pop O2 from the stack into the RPN expression. - Then push o1 onto the stack - When you get to the end of the infix expression, pop (and append to the RPN expression) all remaining operators. The following table summarizes the operator precedence, from the highest precedence at the top to the lowest precedence at the bottom. Operators in the same box have the same precedence. For example, given the expression 3+42/(15)23 You may (and should) assume that a well formatted, correct infix expression containing only numbers, the specified operators, and parentheses will be passed to your function. You may also assume that all tokens are separated by exactly one space. You may (and should) use the Python string methods str.split (to split the string into token) and str . join (to join the RPN expression when you're done)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
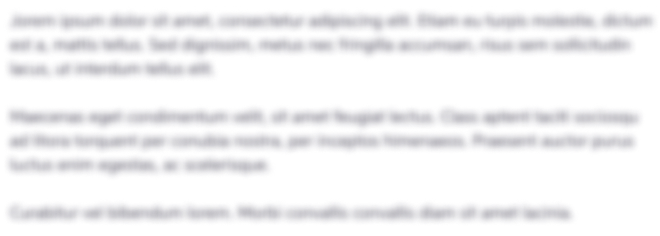
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started