Question
fill in todo section QUEST.JAVA public class Quest implements Cloneable, Comparable { protected String questName; protected String questArea; protected Integer experienceValue; protected Integer id; /**
fill in todo section
QUEST.JAVA
public class Quest implements Cloneable, Comparable {
protected String questName;
protected String questArea;
protected Integer experienceValue;
protected Integer id;
/**
* @param questName Name of the quest
* @param questArea Area of the word in which the quest takes place
* @param experienceValue Experience value of the quest once completed.
*/
public Quest(Integer id, String questName, String questArea, Integer experienceValue) {
super();
this.questName = questName;
this.questArea = questArea;
this.experienceValue = experienceValue;
this.id = id;
}
/**
* @return the id
*/
public Integer id() {
return id;
}
/**
* @param id the id to set
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return the questName
*/
public String questName() {
return questName;
}
/**
* @param questName the questName to set
*/
public void setQuestName(String questName) {
this.questName = questName;
}
/**
* @return the questArea
*/
public String questArea() {
return questArea;
}
/**
* @param questArea the questArea to set
*/
public void setQuestArea(String questArea) {
this.questArea = questArea;
}
/**
* @return the experienceValue
*/
public int experienceValue() {
return experienceValue;
}
/**
* @param experienceValue the experienceValue to set
*/
public void setExperienceValue(int experienceValue) {
this.experienceValue = experienceValue;
}
@Override
public String toString() {
return this.id + ", " + this.questName + ", " + this.questArea +
", XP: " + this.experienceValue;
}
@Override
public int compareTo(Quest o) {
return experienceValue.compareTo(o.experienceValue);
}
}
import lib280.graph.Vertex280;
public class QuestVertex extends Vertex280 {
protected Quest quest;
public QuestVertex(int i) {
super(i);
}
/**
* @return the quest
*/
public Quest quest() {
return quest;
}
/**
* @param quest the quest to set
*/
public void setQuest(Quest quest) {
this.quest = quest;
}
public String toString() {
return this.quest.toString() ;
}
}
public class QuestProgression {
// File format for quest data:
// First line: Number of quests N
// Next N lines consist of the following items, separated by commas:
// quest ID, quest name, quest area, quest XP
// (Quest ID's must be between 1 and N, but the line for each quest IDs may appear in any order).
// Remaining lines consist of a comma separated pair of id's i and j where i and j are quest IDs indicating
// that quest i must be done before quest j (i.e. that (i,j) is an edge in the quest graph).
/**
* Read the quest data from a text file and build a graph of quest prerequisites.
* @param filename Filename from which to read quest data.
* @return A graph representing quest prerequisites. If quest with id i must be done before a quest with id j, then there is an edge in the graph from vertex i to vertex j.
*/
public static GraphMatrixRep280> readQuestFile(String filename) {
Scanner infile;
// Attempt to open the input filename.
try {
infile = new Scanner(new File(filename));
} catch (FileNotFoundException e) {
System.out.println("Error: Unable to open" + filename);
e.printStackTrace();
return null;
}
// Set the delimiters for parsing to commas, and vertical whitespace.
infile.useDelimiter("[,\\v]");
// Read the number of quests for which there is data.
int numQuests = infile.nextInt();
// read the quest data for each quest.
LinkedList280 questList = new LinkedList280();
for(int i=0; i < numQuests; i++) {
int qId = infile.nextInt();
String qName = infile.next();
String qArea = infile.next();
int qXp = infile.nextInt();
questList.insertLast(new Quest(qId, qName, qArea, qXp));
}
// Make a graph with the vertices we created from the quest data.
GraphMatrixRep280> questGraph =
new GraphMatrixRep280> (numQuests, true, "QuestPrerequisites.QuestVertex", "lib280.graph.Edge280");
// Add enough vertices for all of our quests.
questGraph.ensureVertices(numQuests);
// Store each quest in a different vertex. The quest with id i gets stored vertex i.
questList.goFirst();
while(questList.itemExists()) {
questGraph.vertex(questList.item().id()).setQuest(questList.item());
questList.goForth();
}
// Continue reading the input file for the quest prerequisite informaion and add an edge to the graph
// for each prerequisite.
while(infile.hasNext()) {
questGraph.addEdge(infile.nextInt(), infile.nextInt());
}
infile.close();
return questGraph;
}
/**
* Test whether vertex v has incoming edges or not
* @param G A graph.
* @param v The integer identifier of a node in G (corresponds to quest ID)
* @return Returns true if v has no incoming edges. False otherwise.
*/
public static boolean hasNoIncomingEdges(GraphMatrixRep280> G, int v) {
return false;
// TODO Write this method
//return false; // replace this with your own return statement -- this is just a placeholder to prevent compiler errors.
}
/**
* Perform a topological sort of the quests in the quest prerequisite graph G, with priority given
* to the highest experience value among the available quests.
* @param G The graph on which to perform a topological sort.
* @return A list of quests that is the result of the
* topological sort, that is, the order in which the quests should be done if
* we always pick the available quest with the largest XP reward first.
*/
public static LinkedList280 questProgression(GraphMatrixRep280> G) {
// TODO Write this method
return null; // Replace this with your own return statement -- this is jsut a placeholder to prevent compiler errors.
}
public static void main(String args[]) {
// Read the quest data and construct the graph.
// If you get an error reading the file here and you're using Eclipse,
// remove the 'QuestPrerequisites-Template/' portion of the filename.
GraphMatrixRep280> questGraph = readQuestFile("QuestPrerequisites-Template/quests16.txt");
// Perform a topological sort on the graph.
LinkedList280 questListForMaxXp = questProgression(questGraph);
// Display the quests to be completed in the order determined by the topologial sort.
questListForMaxXp.goFirst();
while(questListForMaxXp.itemExists()) {
System.out.println(questListForMaxXp.item());
questListForMaxXp.goForth();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
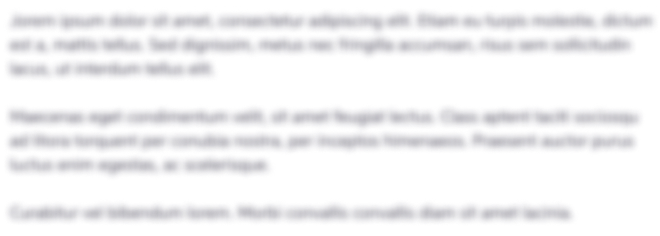
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started