Question
* FILL IN WHERE IT SAYS #YOUR CODE HERE PLEASE* Although we can take the derivatives of various expressions, the results might not be
* FILL IN WHERE IT SAYS "#YOUR CODE HERE " PLEASE*
Although we can take the derivatives of various expressions, the results might not be exactly what we were hoping for. For example, the derivative of 5+1 with respect to should be 5, but it comes out as Plus(Plus(Multiply(0, V('y')), 5), 0). This is equivalent to 5 (because 0=0, 0+5=5, and 5+0=5), but it is rather ugly to read:
[ ]
e = 5 * V('y') + 1
e.derivative('y')
Although calling eval() would help in many cases, in this situation calling eval() on e.derivative('y') would not help, because we do not have a value for the variable V('y'). But what we can do instead is implement a method to simplify expressions like this one.
For this problem, you will write methods implementing simplification of expressions for each subclass of Expr. You should implement the following simplifications:
- Plus(0, e) and Plus(e, 0) should simplify to e, for any e.
- Minus(e, 0) should simplify to e, for any e.
- Minus(0, e) should simplify to Negative(e).
- Multiply(0, e) and Multiply(e, 0) should simplify to 0, for any e.
- Multiply(1, e) and Multiply(e, 1) should simplify to e, for any e.
- Divide(0, e) should simplify to 0, for any e.
- Divide(e, 1) should simplify to e, for any e.
- Power(1, e) should simplify to 1, for any e.
- Power(e, 0) should simplify to 1, for any e.
- Power(e, 1) should simplify to e, for any e.
- Logarithm(1) should simplify to 0.
- Negative(0) should simplify to 0.
The simplification rules should be applied recursively over the expression, so that V('x') * (1 + V('y') * 0) should be simplifed to V('x').
To get you started, we have implemented a simplify method for the Expr class, which relies on each subclass having implemented a simplify_op method. Your task is to implement these simplify_op methods for the Plus, Minus, Multiply, Divide, Logarithm, Power, and Negative classes. The methods you write should implement the simplifications described above for each expression type, and return a simplified expression. If none of the above simplifications are possible, each simplify_op method should call eval() on the expression.
Finally, in order to test your simplify_op implementations, we need to be able to check for expression equality, with a more lenient notion of equality than Python's "same object" notion. The below cell should do the job; be sure to run it before you test your code.
[ ]
def equality_as_same_types_and_attributes(self, other):
return type(self) == type(other) and self.__dict__ == other.__dict__
Expr.__eq__ = equality_as_same_types_and_attributes
[ ]
def expr_simplify(self):
children = [c.simplify() if isinstance(c, Expr) else c
for c in self.children]
e = self.__class__(*children)
return e.simplify_op()
Expr.simplify = expr_simplify
def expr_simplify_op(self):
return self.eval()
Expr.simplify_op = expr_simplify_op
def plus_simplify_op(self):
"""Simplification of Plus expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def minus_simplify_op(self):
"""Simplification of Minus expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def multiply_simplify_op(self):
"""Simplification of Multiply expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def divide_simplify_op(self):
"""Simplification of Divide expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def power_simplify_op(self):
"""Simplification of Power expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def logarithm_simplify_op(self):
"""Simplification of Logarithm expressions."""
# YOUR CODE HERE
raise NotImplementedError()
def negative_simplify_op(self):
"""Simplification of Negative expressions."""
# YOUR CODE HERE
raise NotImplementedError()
Plus.simplify_op = plus_simplify_op
Minus.simplify_op = minus_simplify_op
Multiply.simplify_op = multiply_simplify_op
Divide.simplify_op = divide_simplify_op
Logarithm.simplify_op = logarithm_simplify_op
Power.simplify_op = power_simplify_op
Negative.simplify_op = negative_simplify_op
[ ]
### Tests for simplification of Plus expressions
e = V('x') + 0
assert_equal(e.simplify(), V('x'))
e = 0 + V('x')
assert_equal(e.simplify(), V('x'))
e = 0 + (0 + V('x')) + V('y')
assert_equal(e.simplify(), V('x') + V('y'))
e = 2 + 3 + V('x') + 0
assert_equal(e.simplify(), 5 + V('x'))
[ ]
### Tests for simplification of Minus expressions
e = V('x') - 0
assert_equal(e.simplify(), V('x'))
e = 0 - V('x') # Must evaluate to Negative(V('x'))
assert_equal(e.simplify(), -V('x'))
[ ]
### Tests for simplification of Multiply expressions
e = V('x') * 1
assert_equal(e.simplify(), V('x'))
e = 1 * V('x') * 1 * 1 * 1
assert_equal(e.simplify(), V('x'))
e = 1 * V('x') * 1 * 0 * V('y')
assert_equal(e.simplify(), 0)
[ ]
### Tests for simplification of Divide expressions
e = 0 * V('x') / 3
assert_equal(e.simplify(), 0)
e = V('x') / 1
assert_equal(e.simplify(), V('x'))
[ ]
### Tests for simplification of Power expressions
e = V('x') ** 0
assert_equal(e.simplify(), 1)
e = V('z') ** 1
assert_equal(e.simplify(), V('z'))
e = 1 ** (V('x') + 2)
assert_equal(e.simplify(), 1)
[ ]
### Tests for simplification of Logarithm expressions
e = Logarithm(1)
assert_equal(e.simplify(), 0)
e = Logarithm(1) + 0 * 3
assert_equal(e.simplify(), 0)
e = Logarithm(1) + Logarithm(0.5)
assert_almost_equal(e.simplify(), math.log(0.5))
[ ]
### Overall tests
e = ((3 - 3) * ((V('x') - 2) / (V('y') - 2))) + (V('x') - 1) / (3 - 2)
assert_equal(e.simplify(), V('x') - 1)
*EXPR CLASS*
class Expr:
"""Abstract class representing expressions"""
def __init__(self, *args):
self.children = list(args)
self.child_values = None
def eval(self, env=None):
"""Evaluates the value of the expression with respect to a given
environment."""
# First, we evaluate the children.
# This is done here using a list comprehension,
# but we also could have written a for loop.
child_values = [c.eval(env=env) if isinstance(c, Expr) else c
for c in self.children]
# Then, we evaluate the expression itself.
if any([isinstance(v, Expr) for v in child_values]):
# Symbolic result.
return self.__class__(*child_values)
else:
# Concrete result.
return self.op(*child_values)
def op(self, *args):
"""The op method computes the value of the expression, given the
numerical value of its subexpressions. It is not implemented in
Expr, but rather, each subclass of Expr should provide its
implementation."""
raise NotImplementedError()
def __repr__(self):
"""Represents the expression as the name of the class,
followed by all the children in parentheses."""
return "%s(%s)" % (self.__class__.__name__,
', '.join(repr(c) for c in self.children))
# Expression constructors
def __add__(self, other):
return Plus(self, other)
def __radd__(self, other):
return Plus(self, other)
def __sub__(self, other):
return Minus(self, other)
def __rsub__(self, other):
return Minus(other, self)
def __mul__(self, other):
return Multiply(self, other)
def __rmul__(self, other):
return Multiply(other, self)
def __truediv__(self, other):
return Divide(self, other)
def __rtruediv__(self, other):
return Divide(other, self)
def __pow__(self, other):
return Power(self, other)
def __rpow__(self, other):
return Power(other, self)
def __neg__(self):
return Negative(self)
class V(Expr):
"""Variable."""
def __init__(self, *args):
"""Variables must be of type string."""
assert len(args) == 1
assert isinstance(args[0], str)
super().__init__(*args)
def eval(self, env=None):
"""If the variable is in the environment, returns the
value of the variable; otherwise, returns the expression."""
if env is not None and self.children[0] in env:
return env[self.children[0]]
else:
return self
class Plus(Expr):
def op(self, x, y):
return x + y
class Minus(Expr):
def op(self, x, y):
return x - y
class Multiply(Expr):
def op(self, x, y):
return x * y
class Divide(Expr):
def op(self, x, y):
return x / y
class Power(Expr):
def op(self, x, y):
return x ** y
class Negative(Expr):
def op(self, x):
return -x
Step by Step Solution
There are 3 Steps involved in it
Step: 1
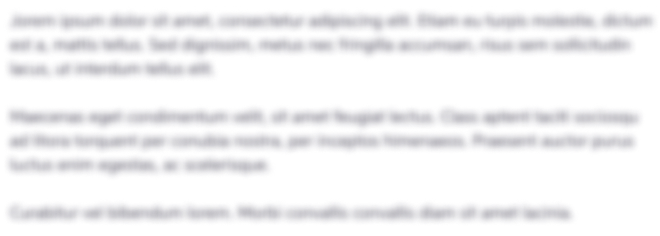
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started